CO1105 Lecture 3: Control Statements PDF
Document Details
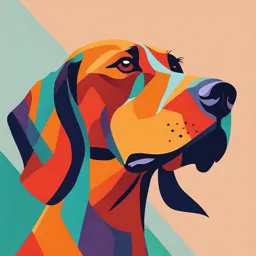
Uploaded by UndisputableFlerovium
University of Leicester
Ghaith Al-Tameemi
Tags
Summary
This document is a lecture on control statements in object-oriented programming using Java. It covers basic problem-solving techniques, algorithms, pseudocode, and various control structures like sequence, selection, and repetition statements. The document is intended for undergraduate-level students in computing.
Full Transcript
CO1105: Introduction to Object Oriented Programming Lecture 3: Control Statements Ghaith Al-Tameemi School of Computing and Mathematical Sciences, University of Leicester https://le.ac.uk/ 0-2 Content Learning Objectives Problem Solving Control Structures if sele...
CO1105: Introduction to Object Oriented Programming Lecture 3: Control Statements Ghaith Al-Tameemi School of Computing and Mathematical Sciences, University of Leicester https://le.ac.uk/ 0-2 Content Learning Objectives Problem Solving Control Structures if selection statement Repetition Statement Compound Assignment Operators Counter-Controlled Repetition Introduction to Java Learning Objectives 1-1 Learning Objectives To learn and use basic problem-solving techniques. To develop algorithms using pseudo codes. To use if and if...else selection statements. To use while repetition statement. To use for and do..while statements. To use switch statement. To use continue and break statements. To use logical operators. Introduction to Java Problem Solving 2-1 Programming like a Professional! Before writing a program, you should have a thorough understanding of the problem and a carefully planned approach to solving it. Understand the types of building blocks that are available and employ proven program-construction techniques. Introduction to Java Problem Solving 2-2 Algorithms Any computing problem can be solved by executing a series of actions in a specific order. An algorithm describes a procedure for solving a problem in terms of ▶ the actions to execute. ▶ the order in which these actions execute. Specifying the order in which statements (actions) execute in a program is called program control. Introduction to Java Problem Solving 2-3 Pseudocode Pseudocode is an informal language for developing algorithms. Similar to everyday English. Helps you "think out" a program. Pseudocode normally describes only statements representing the actions, e.g., input, output or calculations. Carefully prepared pseudocode can be easily converted to a corresponding Java program. Introduction to Java Control Structures 3-1 Control Structures Sequential execution: normally, statements in a program are executed one after the other in the order in which they are written. Transfer of control: various Java statements enable you to specify the next statement to execute, which is not necessarily the next one in sequence. All programs can be written in terms of only three control structures: ▶ the sequence structure ▶ the selection structure ▶ the repetition structure Introduction to Java Control Structures 3-2 Sequence Structure Unless directed otherwise, computers execute Java statements one after the other in the order in which they’re written. The activity diagram below illustrates a typical sequence structure in which two calculations are performed in order. \\Corresponding Java statement: total = total + grade; \\Corresponding Java statement: counter = counter + 1; Introduction to Java Control Structures 3-3 Selection Structure Three types of selection statements: ▶ if statement ▶ if...else statement ▶ switch statement Introduction to Java Control Structures 3-4 Repetition Structure Three repetition statements (looping statements). Perform statements repeatedly while a loop-continuation condition remains true. ▶ while statement ▶ for statement ▶ do...while statement Introduction to Java if selection statement 4-1 if Single-Selection Statement Pseudocode: If student's grade is greater than or equal to 60 Print "Passed" Java code: if ( studentGrade >= 60 ) System.out.println( "Passed" ); Introduction to Java if selection statement 4-2 if..else Double-Selection Statement Pseudocode: If student's grade is greater than or equal to 60 Print "Passed" Else Print "Failed" Value Java code: if ( grade >= 60 ) System.out.println( "Passed" ); else System.out.println( "Failed" ); Introduction to Java if selection statement 4-3 A More Complex Example Pseudocode: If student's grade is greater than or equal to 90 Print "A" else If student's grade is greater than or equal to 80 Print "B" else If student's grade is greater than or equal to 70 Print "C" else If student's grade is greater than or equal to 60 Print "D2" else Print "F" Introduction to Java if selection statement 4-4 A More Complex Example Translate the pseudocode to real Java code: if ( studentGrade >= 90 ) System.out.println( "A" ); else if ( studentGrade >= 80 ) System.out.println( "B" ); else if ( studentGrade >= 70 ) System.out.println( "C" ); else if ( studentGrade >= 60 ) System.out.println( "D" ); else System.out.println( "F" ); Introduction to Java Repetition Statement 5-1 while Repetition Statement Repeat an action while a condition remains true Pseudocode While there are more items on my shopping list Purchase next item and cross it off my list The repetition statement’s body may be a single statement or a block. Eventually, the condition should become false, and the repetition terminates, and the first statement after the repetition statement executes (otherwise, endless loop). Introduction to Java Repetition Statement 5-5 Example Example of Java’s while repetition statement: find the first power of 3 larger than 100. int product = 3; while ( product