Chapter04.pptx
Document Details
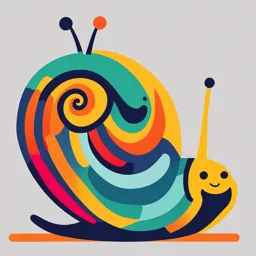
Uploaded by HighSpiritedGorgon
2016
Tags
Full Transcript
PART TWO The C# Language Essentials © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C1, Slide 1 ...
PART TWO The C# Language Essentials © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C1, Slide 1 Chapter 4 How to work with numeric and string data © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 2 Objectives Applied 1. Given an arithmetic expression and the values for the variables in the expression, evaluate the expression. 2. Use numeric and string data as needed within your applications. That means you should be able to do any of the following: declare and initialize variables and constants code arithmetic expressions and assignment statements use the static methods of the Math class cast and convert data from one type to another use the correct scope for your variables declare and use enumerations work with nullable types and the null-coalescing operator © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 3 Objectives (cont.) Knowledge 1. Distinguish between a variable and a constant and give the naming conventions for each. 2. Describe any of these data types: int, double, decimal, bool, and string. 3. Describe any of these terms: literal value, null value, empty string, concatenate, append, escape sequence, string literal, verbatim string literal, and nullable data type. 4. Describe the order of precedence for arithmetic expressions. 5. Distinguish between implicit casting and explicit casting. 6. Distinguish between a value type and a reference type. 7. Describe the differences in the ways that casting, the ToString method of a data structure, the Parse and TryParse methods of a data structure, and the methods of the Convert class can be used to convert data from one form to another. 8. Describe the differences between class scope and method scope. © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 4 1) The built-in value types © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 5 2) How to declare and initialize a variable in two statements Description A variable stores a value that can change as a program executes. Naming convention Start the names of variables with a lowercase letter, and capitalize the first letter of each word after the first word. This is known as camel notation. Syntax type variableName; variableName = value; Example int counter; counter = 1; © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 6 3) How to declare and initialize a variable in one statement Syntax type variableName = value; Examples int counter = 1; long numberOfBytes = 20000; float interestRate = 8.125f; double price = 14.95; decimal total = 24218.1928m; double starCount = 3.65e+9; char letter = 'A'; bool valid = false; int x = 0, y = 0; © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 7 4) How to declare and initialize a constant Description A constant stores a value that can’t be changed. Naming convention Capitalize the first letter of each word of a constant name. This is known as Pascal notation. Syntax const type ConstantName = value; Examples const int DaysInNovember = 30; const decimal SalesTax =.075m; © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 8 5) How to work with scope The scope of a variable determines what code has access to it. If you try to refer to a variable outside of its scope, it will cause a build error. If you declare a variable within a method, it has method scope. A variable with method scope can only be referred to by statements within that method. If you declare a variable within a class but not within a method, it has class scope. A variable with class scope can be referred to by all of the methods in the class. The lifetime of a variable is the period of time that it’s available for use. A variable with method scope is only available while the method is executing. A variable with class scope is available while the class is instantiated. © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 9 6) Arithmetic operators The order of precedence for arithmetic operations 1. Increment and decrement 2. Positive and negative 3. Multiplication, division, and modulus 4. Addition and subtraction © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 10 6) Arithmetic operators… Arithmetic expressions that use integer int x = 14; int y = 8; int result1 = x + y; // result1 = 22 int result2 = x - y; // result2 = 6 int result3 = x * y; // result3 = 112 int result4 = x / y; // result4 = 1 int result5 = x % y; // result5 = 6 int result6 = -y + x; // result6 = 6 int result7 = --y; // result7 = 7, y = 7 int result8 = ++x; // result8 = 15, x = 15 Arithmetic expressions that use decimal decimal a = 8.5m; decimal b = 3.4m; decimal result11 = a + b; // result11 = 11.9 decimal result12 = a - b; // result12 = 5.1 decimal result13 = a / b; // result13 = 2.5 decimal result14 = a * b; // result14 = 28.90 decimal result15 = a % b; // result15 = 1.7 decimal result16 = -a; // result16 = -8.5 decimal result17 = --a; // result17 = 7.5, a = 7.5 decimal result18 = ++b; // result18 = 4.4, b = 4.4 © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 11 6) Arithmetic operators… A calculation that uses the default order of precedence decimal discountPercent =.2m; // 20% discount decimal price = 100m; // $100 price price = price * 1 – discountPercent; // price = $99.8 A calculation that uses parentheses to specify the order of precedence decimal discountPercent =.2m; // 20% discount decimal price = 100m; // $100 price price = price * (1 – discountPercent); // price = $80 The use of prefixed and postfixed increment and decrement operators int a = 5; int b = 5; int y = ++a; // a = 6, y = 6 int z = b++; // b = 6, z = 5 © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 12 7) Assignment operators Operator Name = Assignment += Addition -= Subtraction *= Multiplication /= Division %= Modulus © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 13 7) Assignment operators… The syntax for a simple assignment statement variableName = expression; Typical assignment statements counter = 7; newCounter = counter; discountAmount = subtotal *.2m; total = subtotal – discountAmount; © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 14 7) Assignment operators… Statements that use the same variable on both sides of the equals sign total = total + 100m; total = total – 100m; price = price *.8m; Statements that use the shortcut assignment operators total += 100m; total -= 100m; price *=.8m; © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 15 In Class Exercise © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 16 8) Casting C# provides for two types of casting. A) Implicit casts are performed automatically and can be used only to convert data with a less precise type to a more precise type. This is called a widening conversion Note: No implicit casts can be done between floating-point types and the decimal type. B) Explicit casts are performed using special methods to: convert data with a less precise type to a more precise type. This is called a widening conversion. convert data from a more precise data type to a less precise data type. This is called a narrowing conversion © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 17 8) Casting… A) implicit casting Casting from less precise to more precise data types byteshortintlongdecimal intdouble shortfloatdouble charint Examples double grade = 93; // convert int to double int letter = 'A'; // convert char to int double a = 95.0; int b = 86, c = 91; double average = (a+b+c)/3; // convert b and c to doubles // (average = 90.666666...) © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 18 8) Casting… B) Explicit casting Widening and Narrowing conversion using special methods. 1) Explicit casting using (type) keyword: The syntax: (type) expression Examples int grade = (int)93.75; // convert double to int (grade = 93) char letter = (char)65; // convert int to char (letter = 'A') double a = 95.0; int b = 86, c = 91; int average = ((int)a+b+c)/3; // convert a to int value (average = 90) decimal result = (decimal)b/(decimal)c; // result has decimal places © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 19 8) Casting… B) Explicit casting… 2) Explicit casting using Convert Class: The covert class contains static methods for converting all of the built-in types. Some of the static methods of the Convert class Method Description ToDecimal(value) Converts the value to the decimal data type. ToDouble(value) Converts the value to the double data type. ToInt32(value) Converts the value to the int data type. ToChar(value) Converts the value to the char data type. ToBool(value) Converts the value to the bool data type. ToString(value) Converts the value to a string object. © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 20 8) Casting… B) Explicit casting… 2) Explicit casting using Convert Class: … Examples decimal subtotal = Convert.ToDecimal(txtSubtotal.Text); // string to decimal int years = Convert.ToInt32(txtYears.Text); // string to int txtSubtotal.Text = Convert.ToString(subtotal); // decimal to string int subtotalInt = Convert.ToInt32(subtotal); // decimal to int © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 21 8) Casting… B) Explicit casting… 3) Explicit casting using Common methods: Method Description ToString([format]) A method that converts the value to its equivalent string representation using the specified format. If the format is omitted, the value isn’t formatted. Parse(string) A static method that converts the specified string to an equivalent data value. If the string can’t be converted, an exception occurs. TryParse(string, result) A static method that converts the specified string to an equivalent data value and stores it in the result variable. Returns a true value if the string is converted. Otherwise, returns a false value. © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 22 8) Casting… B) Explicit casting… 3) Explicit casting using Common methods:… Examples: Statements that use ToString, Parse, and TryParse decimal sales = 2574.98m; string salesString = sales.ToString(); // decimal to string sales = Decimal.Parse(salesString); // string to decimal Decimal.TryParse(salesString, out sales); // string to decimal An implicit call of the ToString method double price = 49.50; string priceString = "Price: $" + price; // automatic ToString call A TryParse method that handles invalid data string salesString = "$2574.98"; decimal sales = 0m; Decimal.TryParse(salesString, out sales); // sales is 0 © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 23 9) Formatting You can use different methods to format numbers. There are two ways to format numbers: A) Using ToString common method B) Using Format method of the Sting Class Table below summarize the standard codes used to format a number © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 24 9) Formatting… A) Using ToString method to format a number Statement Example string monthlyAmount = amount.ToString("c"); $1,547.20 string interestRate = interest.ToString("p1"); 2.3% string quantityString = quantity.ToString("n0"); 15,000 string paymentString = payment.ToString("f3"); 432.818 © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 25 9) Formatting… B) Using Format method of the String class to format number You must also provide two arguments: The first argument is a string literal that contains the format specification for the value to be formatted. The second argument is the value to be formatted. syntax of the format specification used by the Format method {index:formatCode} Note: the index indicates the value to be formatted. So, If we want to format single value the the index value will be 0 Examples Statement Result string monthlyAmount = String.Format("{0:c}", 1547.2m); $1,547.20 string interestRate = String.Format("{0:p1}",.023m); 2.3% string quantityString = String.Format("{0:n0}", 15000); 15,000 string paymentString = String.Format("{0:f3}", 432.8175); 432.818 © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 26 In Class Exercise © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 27 10) Math class Math class provide some methods for performing mathematical operations. The common five methods of math class are as follwoing: 1) The syntax of the Round method Math.Round(decimalNumber[, precision[, mode]]) The Round method rounds a decimal argument to the specified precision, which i s the number o f significant decimal digits. If the precision i s omitted, the number is rounded to the nearest whole n u m b e r. By d e f a u l t , i f you round a decimal value that ends in 5, the number i s rounded to t h e nearest even d e c i m a l v a l u e. This is referred to as banker's r o u n d i n g. Y o u can a l s o c o d e the mode argument with a value o f M i d p o i n t R o u n d i n g. A w a y F r o m Z e r o to r o u n d positive values up and negative values d o w n. 2) The syntax of the Pow method Math.Pow(number, power) 3) The syntax of the Sqrt method Math.Sqrt(number) 4) The syntax of the Min and Max methods Math.{Min|Max}(number1, number2) © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 28 10) Math class… Example1 Math.Round(23.75, 1) 23.8 Math.Round(23.85, 1) 23.8 Math.Round(23.744, 2) 23.74 Math.Round(23.745, 2) 23.74 Math.Round(23.745, 2, MidpointRounding.AwayFromZero) 23.75 Math.Pow(5, 2) 25 Math.Sqrt(20.25) 4.5 Math.Max(23.75, 20.25) 23.75 Math.Min(23.75, 20.25) 20.25 Example2 int shipWeight = Math.Round(shipWeightDouble); // round to a whole number double orderTotal = Math.Round(orderTotal, 2); // round to 2 decimal places double area = Math.Pow(radius, 2) * Math.PI; // area of circle double sqrtX = Math.Sqrt(x); double maxSales = Math.Max(lastYearSales, thisYearSales); int minQty = Math.Min(lastYearQty, thisYearQty); © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 29 11) String Data Type How to declare and initialize a string string message1 = "Invalid data entry."; string message2 = ""; //known value but the string doesn't contain any character string message3 = null; //unknown value How to join strings To j o i n , or c o n c a t e n a t e , a string with another s t r i n g or a value data t y p e , use a p lu s sig n +. string firstName = "Bob"; // firstName is "Bob" string lastName = "Smith"; // lastName is "Smith" string name = firstName + " " + lastName; // name is "Bob Smith" How to join a string and a number If y o u c o n c a te n a te a v a lu e d a ta ty p e to a s tr in g , C # w ill a u to m a tic a lly c o n v e r t th e v a lu e to a s tr in g double price = 14.95; string priceString = "Price: $" + price; // priceString is "Price: $14.95" © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 30 11) String Data Type… Common escape sequences Key Description \n New line \t Tab \r Return \\ Backslash \" Quotation Examples that use escape sequences Code Result string code = "JSPS"; decimal price = 49.50m; string result = "Code: " + code + "\n" + "Price: $" + price + "\n"; Code: JSPS Price: $49.50 string names = "Joe\tSmith\rKate\tLewis\r"; Joe Smith Kate Lewis string path = "c:\\c#.net\\files"; c:\c#.net\files string message = "Type \"x\" to exit"; Type "x" to exit © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 31 11) String Data Type… Use verbatim string literals T o code a verbatim s t r i n g l i t e r a l , you can code an @ sign, followed by an o p e n i n g double q u o t e , followed by the string, followed by a closing double q u o t e. W i t h i n the string, you can enter backslashes, tabs, new line characters, and other s p e c i a l characters without using escape s e q u e n c e s. H o w e v e r , to enter a double quote w i t h i n a verbatim string literal, you must enter two double q u o t e s. Examples Code Result string names = @"Joe Smith Joe Smith Kate Lewis"; Kate Lewis string path = @"c:\c#.net\files"; c:\c#.net\files string message = @"Type ""x"" to exit"; Type "x" to exit © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 32 12 NULL Value A nullable type is a value type that can store a null value. Null values are typically used to indicate that the value of the variable is unknown. There are three ways to declare a value type that can contain null values Syntax Nullable variableName [= expression]; type? variableName [=expression]; type ?variableName [=expression]; Examples Nullable quantity; quantity = 20; quantity = null; decimal? salesTotal; decimal totalSales?; bool? isValid = null; string? message = null; // not necessary or allowed © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 33 12 NULL Value… There are Two properties for working with nullable types Property Description HasValue Returns a true value if the nullable type contains a value. Returns a false value if the nullable type is null. Value Returns the value of the nullable type. How to check if a nullable type contains a value bool hasValue = quantity.HasValue; How to get the value of a nullable type int qty = quantity.Value; © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 34 12 NULL Value… How to use the null-coalescing operator to assign a default value T h e n u l l - c o a l e s c i n g o p e r a to r ( ? ? ) returns the value o f t h e l e f t operand i f i t i s n ' t n u l l or the r i g h t operand i f it i s. Example: int? quantity=null; int qty = quantity ?? -1; //qty = -1 Note: I f you use a v a ri a b l e w i t h a n u l l a b l e t y p e in an arithmetic e x p r e s s i o n and the v a l u e o f t h e v a ri a b l e i s n u l l , the re s u l t o f the a r i t h m e t i c e x p r e s s i o n is always n u l l. Example: decimal? sales1 = 3267.58m; decimal? sales2 = null; decimal? salesTotal = sales1 + sales2; // result = null © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 35 In Class Exercise With Null values Without Null values Note: we need to use simple if statement © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 36 In Class Exercise Solution private void btncalculate_Click(object sender, EventArgs e) { double? basicsalary; int? numberofchilds; //calculate childs bonus if (txtnumberofchilds.Text = = "") numberofchilds = null; else numberofchilds = Convert.ToInt16(txtnumberofchilds.Text); int cb; cb = (numberofchilds * 10) ?? 0; txtcb.Text = cb.ToString(); //calculate income tax double ix; if (txtnetsalary.Text = = "") basicsalary = null; else basicsalary = Convert.ToDouble(txtbs.Text); ix = (basicsalary * 0.05) ?? (1450 * 0.05); txtix.Text = ix.ToString(); //calculate net salary double ns = (basicsalary ?? 1450) + cb - ix; txtnetsalary.Text = ns.ToString(); } © 2016, Mike Murach & Associates, Inc. Murach's C# 2015 C4, Slide 37