Chapter 1- Java Programming PDF
Document Details
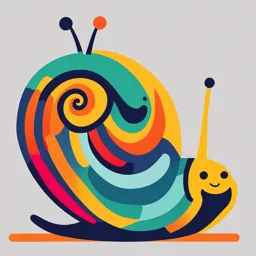
Uploaded by SelfSufficientGreatWallOfChina2872
JU CS
Mekdes.S(Msc)
Tags
Summary
This document provides an overview of Java programming, including data types, arrays, decision and repetition statements, and exception handling. It also discusses the characteristics of Java, such as its platform independence, security features, and high performance compared to other programming languages.
Full Transcript
Java Programming (Cosc3053) Pre-request: OOP For Computer Science 3rd year (BSc. Degree ) Instructor:Mekdes.S(Msc) 1 JU CS Compiled by MS 12/19/2024 Chapter One: Overview of Java Programming Outline – Data types and variables...
Java Programming (Cosc3053) Pre-request: OOP For Computer Science 3rd year (BSc. Degree ) Instructor:Mekdes.S(Msc) 1 JU CS Compiled by MS 12/19/2024 Chapter One: Overview of Java Programming Outline – Data types and variables – Arrays – Decision and Repetition statement – Exception handling overview 2 JU CS Compiled by MS 12/19/2024 What is Java & why Java? Ø Java is a high level programming language and a platform. Ø Java is a robust, secured and object-oriented programming language. Ø Platform: Any hardware or software environment in which a program runs, is known as a platform. Ø Since Java has its own runtime environment (JRE) and API, it is called platform(support Software platform). Ø Java is a general purpose programming language. Why java?:-Java enables users to develop and deploy applications on the Internet for servers, desktop computers(standalone applications), and small hand-held devices. 3 JU CS Compiled by MS 12/19/2024 Types Of Java Programs Cont... Java Applet program Java Application program No. Small Program. Large Program 1) Used to run a program on client Browser Can be executed on standalone computer 2) system like any other program Applet is portable and can be executed by Need JDK, JRE, JVM installed on client 3) any JAVA supported browser. machine to executed executed in a Restricted Environment. Application can access all the resources of 4) the computer No main() method Application have main() method 5) Applet is said to be UNTRUSTED so need Application does not need high security. 6) high security. Applet runs on client side. Application directly runs on your machine. 7) Applet requires an HTML file before it can Application don’t requires an HTML file 8) be executed. before Applets are run Application run using java command 9) 4 JU CS Compiled by MS 12/19/2024 using appletviewer command. Characteristics of Java 5 JU CS Compiled by MS 12/19/2024 Cont... q Simple: Ø Java is partially modeled on C++, but greatly simplified and improved. Ø Removed many confusing and/or rarely-used features e.g. pointers, operator overloading and multiple inheritance etc. Ø Java is designed to be easy to learn. If you understand the basic concept of OOP java would be easy to master. q Object-oriented: Ø Java is truly/inherently object oriented language. In java almost everything is an object, because all program code and data exist in objects and classes. Ø Java comes with extensive set of classes organized in packages that an be used in program by in heritance. Ø Object oriented programming (OOP) is a popular programming approach that is replacing traditional procedural programming techniques 6 JU CS Compiled by MS 12/19/2024 Cont... qCompiled and Interpreted:- Ø Basically computer language is either compiled or interpreted; but java comes together both these approaches thus making java a two-stage system. Ø Java compiler translates java code to Java virtual machine code which is called Bytecode. q Robust and secure: Ø Java compilers can detect many errors that would first show up at execution time in other languages. Ø Java has a runtime exception handling feature to provide programming support for robustness. Ø Java implements several security mechanisms to protect your system against harm caused by stray programs 7 JU CS Compiled by MS 12/19/2024 Cont... qPlatform independent and Portable:- Ø Java code can be run on multiple platforms e.g. Windows, Linux, Sun Solaris, Mac/OS and etc. Ø Java program can be easily moved from one computer system to another and anywhere(Portability). Ø Java certifies portability feature in two ways: üJava compiler generate bytecode that can be executed on any machine. üThe size of primitive data types are fixed/machine independent(Architecture--Neutral). q High Performance:- Ø Java is faster than traditional interpretation since bytecode is "close" to native code still somewhat slower than a compiled language (e.g., C++) 8 JU CS Compiled by MS 12/19/2024 Cont... qDistributed:- ØWe can create distributed applications in java. RMI is used for creating distributed applications. We may access files by calling the methods from any machine on the internet. q Multithreaded:- Ø A thread is like a separate program, executing concurrently. We can write Java programs that deal with many tasks at once by defining multiple threads. ØThe main advantage of multi-threading is that it doesn't occupy memory for each thread. It shares a common memory area 9 JU CS Compiled by MS 12/19/2024 Basic Synatx It is very important to keep in mind the following points in java programming. Case Sensitivity : Java is case sensitive, which means identifier Hello and hello would have different meaning in Java. Class Names : For all class names the first letter should be in Upper Case. If several words are used to form a name of the class, each inner word's first letter should be in Upper Case. Example: class MyFirstJavaClass Method Names : All method names should start with a Lower Case letter. If several words are used to form the name of the method, then each inner word's first letter should be in Upper Case. 10 JU CS Compiled by MS 12/19/2024 Cont... Program File Name: Name of the program file should exactly match the class name. public static void main(String args[]): Java program processing starts from the main() method which is a mandatory part of every Java program. 11 JU CS Compiled by MS 12/19/2024 Creating, Compiling, and Executing/Running java Programs Java programs have five phases Phase1: Create/Edit Source code. v Use an editor to type Java program v Text editor(e.g. Notepad ) and IDE (e.g. netbean & Eclipse ) v Source code must save with filename.java and filename must have the same name with class name v Program is created in the editor and store on disk. Phase2:- Compile v The java compiler translate Source code into bytecodes and create filename.class file(again filename is same with class name) v Bytecode stored on disk, understood by Java interpreter v javac command used to compile source code: javac Welcome.java v If your program has compilation errors, you have to modify the program to fix them, then compile it. 12 JU CS Compiled by MS 12/19/2024 Cont... Phase 3: Loading v JVM loads the Bytecode of the class to Main memory using a program called class loader. v If your program uses other classes, the class loader dynamically loads them just before they are needed. Phase 4: Verify v JVM uses a program called Bytecode verifier to check the valid of the bytecodes and to ensure that the bytecode does not violate java’s security v Java must be secure - Java programs transferred over networks, possible to damage files (viruses) Phase 5: Execute v To execute java program is to run program’s bytecode which can be executed on any platform with a JVM. v Java bytecode is interpreted. Interpreting translates the individual steps in the bytecode into the target machine language code one at a time rather than translating the whole program as a single unit. v Each step is executed immediately after it is translated 13 JU CS Compiled by MS 12/19/2024 Cont... v Java command used to execute the.class file e.g. java Welcome v If your program has runtime errors or does not produce the correct result , you have to modify the program, recompile it, and execute again. 14 JU CS Compiled by MS 12/19/2024 A Simple Java program // write java program that prints Welcome to Java! public class Welcome { public static void main(String args[]) { System.out.println("Welcome to Java!"); } } 15 JU CS Compiled by MS 12/19/2024 Anatomy of a Java Program Ø Comments Ø Package Ø Reserved words Ø Modifiers Ø Classes Ø Methods(main method) Ø Statements Ø Blocks 16 JU CS Compiled by MS 12/19/2024 Comments Ø comment: A note written in the source code by the programmer to make the code easier to understand. Comments are not executed when your program runs. Ø Comment, general syntax: // or In this example 1. // This program prints Welcome to Java! 2. public class Welcome { 3. public static void main(String[] args) { 4. System.out.println("Welcome to Java!"); 5. } 6. } 17 JU CS Compiled by MS 12/19/2024 Package ØPackage is a collection of related classes Ø It can be predefined and user defined package. Ø Predefined package is built by java developers. e.g. java.lang, java.util, javax.swing, java.io, and etc. Syntax; import.java.lang.Object; import.java.util.Scanner; import.java.swing.*; Ø user defined package is defined by java users; e.g. package person; package shape; 18 JU CS Compiled by MS 12/19/2024 Reserved words ØReserved words or keywords are words that have a specific meaning to the compiler and cannot be used for other purposes in the program. Øwhen the compiler sees the word class, it understands that the word after class is the name for the class. 1. // This program prints Welcome to Java! 2. public class Welcome { 3. public static void main(String args [] ) { 4. System.out.println("Welcome to Java!"); 5. } 6. } 19 JU CS Compiled by MS 12/19/2024 Modifiers Øava uses certain reserved words called modifiers that specify the properties of the data, methods, and classes and how they can be used. Ø Examples of modifiers are public, private, protected, static, final, and abstract. 1. // This program prints Welcome to Java! 2. public class Welcome { 3. public static void main(String args[]) { 4. System.out.println("Welcome to Java!"); 5. } 6. } 20 JU CS Compiled by MS 12/19/2024 Classes ØEvery Java program must have at least one class(predefined or user defined class). Ø Each class has a name. Ø By convention, class names start with an uppercase letter. In this example, the class name is Welcome 1. // This program prints Welcome to Java! 2. public class Welcome { 3. public static void main(String args[]) { 4. System.out.println("Welcome to Java!"); 5. } 6. } 21 JU CS Compiled by MS 12/19/2024 Main Method: ØMain Method: Line 3 defines the main method. Ø In order to run a class, the class must contain a method named main. Ø The program is executed from the main method. 1. // This program prints Welcome to Java! 2. public class Welcome { 3. public static void main(String args[]) { 4. System.out.println("Welcome to Java!"); 5. } 6. } 22 JU CS Compiled by MS 12/19/2024 Statements ØA statement represents an action or a sequence of actions. Ø The statement System.out.println("Welcome to Java!") in this example is a statement to display the greeting "Welcome to Java!“. Ø Every statement in Java ends with a semicolon (;). 1. // This program prints Welcome to Java! 2. public class Welcome { 3. public static void main(String[] args) { 4. System.out.println("Welcome to Java!"); 5. } 6. } 23 JU CS Compiled by MS 12/19/2024 Blocks A pair of curly braces in a program forms a block that groups components of a program. public class Test { public static void main(String[] args) { Class block System.out.println("Welcome to Java!"); Method block } } 24 JU CS Compiled by MS 12/19/2024 Blocks styles Use end-of-line style for braces Next-line public class Test style { public static void main(String[] args) { System.out.println("Block Styles"); } } End-of-line style public class Test { public static void main(String[] args) { System.out.println("Block Styles"); } } 25 JU CS Compiled by MS 12/19/2024 Data Types in Java Data types specify the different sizes and values that can be stored in the variable. There are two types of data types in Java: Primitive data types: The primitive data types include Boolean, char, byte, short, int, long, float and double. Non-primitive data types: The non-primitive data types include Classes, Interfaces, and Arrays. Eg.Customer myCustomer1 = new Customer(); Java is a statically-typed programming language. It means, all variables must be declared before its use. That is why we need to declare variable's type and name. 26 JU CS Compiled by MS 12/19/2024 Variables in Java A variable is a container which holds the value while the Java program is executed. A variable is assigned with a data type and it is a name of memory location. There are three types of variables in java: local, instance and static. 27 JU CS Compiled by MS 12/19/2024 Cont... Local Variable: A variable declared inside the body of the method is called local variable. You can use this variable only within that method and the other methods in the class aren't even aware that the variable exists. A local variable cannot be defined with "static" keyword. Instance Variable: A variable declared inside the class but outside the body of the method and it is not declared as static. It is called instance variable because its value is instance specific and is not shared among instances. 28 JU CS Compiled by MS 12/19/2024 Cont… Static variable: It is a variable which is declared as static and cannot be local. You can create a single copy of static variable and share among all the instances of the class. Memory allocation for static variable happens only once when the class is loaded in the memory. class A { int data=50;//instance variable static int m=100;//static variable void method() { int n=90;//local variable } }//end of class 29 JU CS Compiled by MS 12/19/2024 Java- Arrays: Introductions § An array is a set of variables that are referenced using a single variable name combined with an index number. § Each item of an array is called an element. § All the elements in an array must be of the same type. § Thus, the array itself has a type that specifies what kind of elements it can contain. § For example, ü an int array can contain int values, and ü a String array can contain strings values. 30 JU CS Compiled by MS 12/19/2024 What is an Array? § An array is itself an object. ü You can refer to the array object as a whole rather than a specific element of the array by using the array’s variable name without an index. ü e.g if x refers to an element of an array, then x refers to the array itself. § An array has a fixed length that’s set when the array is created. ü This length determines the number of elements that can be stored in the array. ü The maximum index value you can use with any array is one less than the array’s length. ü Thus, if you create an array of 10 elements, you can use index values from 0 to 9. § You can’t change the length of an array after you create the array. 31 JU CS Compiled by MS 12/19/2024 Declaring Array Variables § To use an array in a program, you must declare a variable to reference the array, and you must specify the type of array the variable can reference. § Here is the syntax for declaring an array variable − § Syntax § dataType[] arrayRefVar; // preferred way.or dataType arrayRefVar[]; // works but not preferred way. § Note − The style dataType[] arrayRefVar is preferred. § The style dataType arrayRefVar[] comes from the C/C++ language and was adopted in Java to accommodate C/C++ programmers. 32 JU CS Compiled by MS 12/19/2024 Declaring Array Variables: Example 1 double[] myList; // preferred way.or double myList[]; // works but not preferred way. 33 JU CS Compiled by MS 12/19/2024 Creating Arrays § You can create an array by using the new operator with the following syntax − § Syntax: arrayRefVar = new dataType[arraySize]; § The above statement does two things − § It creates an array using new dataType[arraySize]. § It assigns the reference of the newly created array to the variable arrayRefVar. 34 JU CS Compiled by MS 12/19/2024 Cont... § Declaring an array variable, creating an array, and assigning the reference of the array to the variable can be combined in one statement, as shown below − dataType[] arrayRefVar = new dataType[arraySize]; § Alternatively you can create arrays as follows − dataType[] arrayRefVar = {value0, value1,..., valuek}; § The array elements are accessed through the index. § Array indices are 0-based; that is, they start from 0 to arrayRefVar.length-1. 35 JU CS Compiled by MS 12/19/2024 Example 1 § Following statement declares an array variable, myList, creates an array of 10 elements of double type and assigns its reference to myList – double[] myList = new double; § Following picture represents array myList. § Here, myList holds ten double values and the indices are from 0- 9 36 JU CS Compiled by MS 12/19/2024 Example 2 37 JU CS Compiled by MS 12/19/2024 Array dimensions Array is one type of variable which stores multiple values with same data type. It has many dimensions such as one dimension, two dimensions, or multidimensional. Note: Array is non-primitive data type. 38 JU CS Compiled by MS 12/19/2024 One-Dimensional Arrays (1-D Array) § A 1-D array is a list of similar data typed variables. § Single dimensional arrays store only one column of data. § The general form of a one-dimensional array declaration is type var-name[ ]; Here, type declares the base type of the array. § Thus, the data type for the array determines what type of data the array will hold. § For example, the following declares an array named month_days with the type “array of int”: int month_days[]; 39 JU CS Compiled by MS 12/19/2024 Cont... § All objects in Java (including arrays) must be allocated dynamically with operator new. § For an array, the programmer specifies the type of the array elements and the number of elements as part of the new operation. § The general form of new as it applies to one-dimensional arrays appears as follows: array-var = new type[size]; 40 JU CS Compiled by MS 12/19/2024 Cont... § Here, type specifies the type of data being allocated, § size specifies the number of elements in the array, and § array-var is the array variable that is linked to the array. § That is, to use new to allocate an array, you must specify the type and number of elements to allocate. § The elements in the array allocated by new will automatically be initialized to zero. 41 JU CS Compiled by MS 12/19/2024 Example 1 § This example allocates a 12-element array of integers and links them to month_days. int c[]; // declares the array c = new int[ 12 ]; // allocates the array § After this statement executes, month_days will refer to an array of 12 integers. § Further, all elements in the array will be initialized to zero. § The preceding declaration also can be performed in a single step as follows: int month_days[] = new int; 42 JU CS Compiled by MS 12/19/2024 Initializing an Array § Arrays can be initialized in different ways. § One way to initialize the values in an array is to simply assign them one by one: String[] days = new Array; Days = “Sunday”; Days = “Monday”; Days = “Tuesday”; Days = “Wednesday”; Days = “Thursday”; Days = “Friday”; Days = “Saturday”; 43 JU CS Compiled by MS 12/19/2024 Cont... Java has a shorthand way to create an array and initialize it with constant values: String[] days = { “Sunday”, “Monday”, “Tuesday”, “Wednesday”, “Thursday”, “Friday”, “Saturday” }; In the second case, each element to be assigned to the array is listed in an array initializer. E.g. int[] primes = { 2, 3, 5, 7, 11, 13, 17 }; The length of an array created with an initializer is determined by the number of values listed in the initializer. An alternative way to code an initializer is like this: int[] primes = new int[] { 2, 3, 5, 7, 11, 13, 17 }; To use this type of initializer, you use the new keyword followed by the array type and a set of empty brackets. Then, you code the initializer. 44 JU CS Compiled by MS 12/19/2024 Multidimensional Arrays § In Java, multidimensional arrays are actually arrays of arrays. § When the elements of an array are themselves arrays, we say that the array is multidimensional. § If the number of dimensions is only two, then the array is called a two-dimensional array. § Multidimensional arrays are often used to represent tables of values consisting of information arranged in rows and columns. § To identify a particular table element, we must specify the two subscripts — the first identifies the element’s row and the second identifies the element’s column. § Arrays that require two subscripts to identify a particular element are called two- dimensional arrays. 45 JU CS Compiled by MS 12/19/2024 Two-dimensional arrays § Two-dimensional arrays are often used to track data in a column and row format, much the way a spreadsheet works. § For example, suppose you’re working on a program that tracks five years worth of sales (2001 through 2005) for ABC company, with the data broken down for each of four sales territories (North, South, East, and West). § You could create 20 separate variables, with names such as sales2001North, sales2001South, sales2001East, and so on to store these values. § But that gets a little tedious. 46 JU CS Compiled by MS 12/19/2024 Cont... Thinking of a two-dimensional array as a table or spreadsheet is common, like this: North South East West 2001 23,853 22,838 36,483 31,352 2002 25,483 22,943 38,274 33,294 2003 24,872 23,049 39,002 36,888 2004 28,492 23,784 42,374 39,573 2005 31,932 23,732 42,943 41,734 47 JU CS Compiled by MS 12/19/2024 2-D Example #1 § For example, the following declares a two-dimensional array variable called sales to store. double[][] sales = new double; § Here’s an example that initializes the sales array: double[][] sales = { {23853.0, 22838.0, 36483.0, 31352.0}, // 2001 {25483.0, 22943.0, 38274.0, 33294.0}, // 2002 {24872.0, 23049.0, 39002.0, 36888.0}, // 2003 {28492.0, 23784.0, 42374.0, 39573.0}, // 2004 {31932.0, 23732.0, 42943.0, 41734.0} // 2005 }; 48 JU CS Compiled by MS 12/19/2024 2-D Example #2 Java program to read and print a two dimensional array. (Consider student marks below) Subject 1 Subject 2 Subject 3 Student1 50 80 90 Student2 60 70 89 Student3 65 66 80 49 JU CS Compiled by MS 12/19/2024 Sample Solution Public class Two_D{ Output Public Static void main(String args []){ int [][]StudentsMark=new int ;// 50 allocate memory 80 //store values 80 Studentsmark =50; 0 Studentsmark =80; Studentsmark =80; System.out.println(studentsMark); System.out.println(studentsMark); System.out.println(studentsMark); System.out.println(studentsMark); } } 50 JU CS Compiled by MS 12/19/2024 Decision and Repetition Statements – Overview Topics of cover we will Java While loop statements Do while loop – If statement – Switch statement – For loop 51 JU CS Compiled by MS 12/19/2024 Overview of Java statements Decision making is an important part of programming. It is used to specify the order in which statements are executed. create decisions using different forms of if...else statement. o if Statement o if-else statements o if...else if Statement 52 JU CS Compiled by MS 12/19/2024 if Statement § In Java, an if statement is a conditional statement that runs a different set of statements, § if statement is depending on whether an expression is true or false. § Syntax: if (expression){ statement; //code to be executed if the condition is true } In the above syntax, the if statement evaluates the test expression inside parenthesis. If test expression is evaluated to true (nonzero) , statements inside the body of if is executed. If test expression is evaluated to false (0), statements inside the body of if are skipped. 53 JU CS Compiled by MS 12/19/2024 Example : if Statement § Java Program to demonstrate the use of if statement. public class TestClass { public static void main(String[] args) { int totalMarks=55; if(totalMarks>50){ System.out.print("You have passed the exam !!"); } } } Output: You have passed the exam !! 54 JU CS Compiled by MS 12/19/2024 if-else statements § An if-else statement adds an additional element to a basic if statement: § The else statement is to specify a block of code to be executed, if the condition in the if statement is false. § The basic syntax is if (condition){ //code to be executed if the condition is true } else { //code to be executed if the condition is false } 55 JU CS Compiled by MS 12/19/2024 Example: if…else public class IfElseStat { public static void main(String[] args) { int totalMarks=48; if(totalMarks>50){ System.out.print("You have passed the exam !!"); } else { System.out.print("You have failed the exam !!"); } } } Output: You have failed the exam !! 56 JU CS Compiled by MS 12/19/2024 if...else if Statement § Used to evaluate more than one conditions at the same time. § Multi selection enables the developer to determine the actions that have to be accomplished in certain conditions by imposing a requisite. 57 JU CS Compiled by MS 12/19/2024 if...else if Statement… General Syntax: if(condition1){ //code to be executed if the condition1 is true } else if(condition2){ //code to be executed if condition2 is true} else if(condition3){ //code to be executed if condition3 is true }... else { //code to be executed if all the above conditions are false } 58 JU CS Compiled by MS 12/19/2024 How if...else if Statement works § The if statements are executed from the top down. § As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the ladder is bypassed. § If none of the conditions is true, then the final else statement will be executed. § The final else acts as a default condition; that is, if all other conditional tests fail, then the last else statement is performed. § If there is no final else and all other conditions are false, then no action will take place. 59 JU CS Compiled by MS 12/19/2024 Example 1 § A program that accepts mark and displays letter grade int score = 76; char grade; if (score >= 90) { grade = 'A'; } else if (score >= 80){ grade = 'B'; } else if (score >= 70){ grade = 'C'; } else if (score >= 60){ grade = 'D'; } else{ grade = 'F'; } System.out.println("Grade = " + grade); 60 JU CS Compiled by MS 12/19/2024 Switch Statement § The Java switch statement is used to perform different actions based on different conditions. § It also works with enumerated types , the String class, and a few special classes that wrap certain primitive types: ü Character, ü Byte, ü Short, and ü Integer. 61 JU CS Compiled by MS 12/19/2024 Switch Statement… General Syntax: switch (expression) { case value1: // statement sequence break; case value2: // statement sequence break;... default: // default statement sequence } 62 JU CS Compiled by MS 12/19/2024 How Switch Statement is works ? § The switch expression (condition) is evaluated once and the value of the expression is compared with the values of each case. § If there is a match, the associated block of code is executed. § If no matching case clause is found, the program looks for the optional default clause. § The optional break statement associated with each case label ensures that the program breaks out of switch once the matched statement is executed and continues execution at the statement following switch. § If break is omitted, the program continues execution at the next statement in the switch statement. 63 JU CS Compiled by MS 12/19/2024 Example #1: Switch statement A program that accepts week number and displays week name. public class SwitchStatement { public static void main(String[] args) { // TODO code application logic here int inDay=4; String day; switch (inDay){ case 1: day="Sunday"; break; case 2: day="Monday"; break; case 3: day="Tuesday"; break; case 4: day="Wednesday"; break; case 5: day="Thrusday"; break; case 6: day="Friday"; break; case 7: day="saturday"; Output: select day is: Wednesday break; default: day="Invalid day"; } System.out.println("select day is:"+ day); 64 } JU CS Compiled by MS 12/19/2024 } Switch Statement… § The break statement is optional. § If you omit the break, execution will continue on into the next case. § It is sometimes desirable to have multiple cases without break statements between them. § For example, consider the next program: 65 JU CS Compiled by MS 12/19/2024 Example #2: public class SwitchWoutBreak { public static void main(String[] args) { // TODO code application logic here int inDay=4; String day; switch (inDay){ case 1: day="Sunday"; case 2: day="Monday“; case 3: day="Tuesday"; break; case 4: day="Wednesday; case 5: day=" Thursday "; break; case 6: day="Friday"; break; case 7: day="Saturday"; break; default: day="Invalid day"; } System.out.println("select day is:"+ day); } } Output: select day is: Thursday 66 JU CS Compiled by MS 12/19/2024 Loop § In Java, a loop repeatedly executes the same set of instructions until a termination condition is met. § Java provides three iteration statements as shown in fig: 67 JU CS Compiled by MS 12/19/2024 i. For Loop § The Java for loop is used to iterate a part of the program several times. § If the number of iteration is fixed, it is recommended to use for loop. § There are three types of for loops in java. a. Simple For Loop, b. For-each or Enhanced For Loop and c. Labeled For Loop. 68 JU CS Compiled by MS 12/19/2024 a. Simple For Loop § A simple for loop is the same as C/C++. § We can initi a l i z e t h e v a r i ab l e , c he c k c o ndi t i o n and increment/decrement value. § General Syntax: for(initialization; condition; incr/decr){ //statement or code to be executed } 69 JU CS Compiled by MS 12/19/2024 Simple For Loop… § It consists of four parts: § Initialization: It is the initial condition which is executed once when the loop starts. we can initialize the variable, or we can use an already initialized variable. It is an optional condition. 70 JU CS Compiled by MS 12/19/2024 Simple For Loop… § Condition: § is executed each time to test the condition of the loop. § It continues execution until the condition is false. § It must return boolean value either true or false. ü It is an optional condition. § Increment/Decrement: It increments or decrements the variable value. It is an optional condition. § Statement: The statement of the loop is executed each time until the second condition is false. 71 JU CS Compiled by MS 12/19/2024 Example #1: Simple For Loop //Java Program to demonstrate the example of for loop public class ForExample { public static void main(String[] args) { //Code of Java for loop for(int i=1;i