Ch2_Fundamentals of c.pdf
Document Details
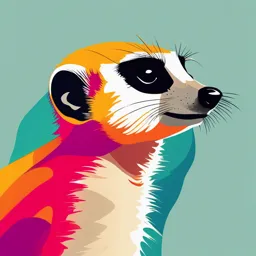
Uploaded by StylizedIvy8770
Tags
Full Transcript
CHAPTER 2 FUNDAMENTALS OF C 2.1 FEATURES OF C ï‚· Simple C is a simple language in the sense that it provides a structured approach (to break the problem into parts), the rich set of library functions, data types, etc. ï‚· General Purpose Language: From system program...
CHAPTER 2 FUNDAMENTALS OF C 2.1 FEATURES OF C ï‚· Simple C is a simple language in the sense that it provides a structured approach (to break the problem into parts), the rich set of library functions, data types, etc. ï‚· General Purpose Language: From system programming to photo editing software, C programming language is used in various applications. Some of the common applications where it’s used are as follows: ï‚· Operating systems: Windows, Linux, iOS, Android, OXS ï‚· Databases: PostgreSQL, Oracle, MySQL, MS SQL Server etc. ï‚· Platform dependent A language is said to be platform dependent whenever the program is execute in the same operating system where that was developed and compiled but not run and execute on other operating system. C is platform dependent programming language. Note:.obj file of C program is platform dependent. ï‚· Machine Independent or Portable It is the concept of carrying the instruction from one system to another system. In C Language.C file contain source code, we can edit also this code..exe file contain application, only we can execute this file. When we write and compile any C program on window operating system that program easily run on other window based system. When we can copy.exe file to any other computer which contain window operating system then it works properly, because the native code of application an operating system is same. But this exe file is not execute on other operation system. ï‚· Mid-level programming language Although, C is intended to do low-level programming. It is used to develop system applications such as kernel, driver, etc. It also supports the features of a high-level language. That is why it is known as mid-level language. ï‚· Structured programming language C is a structured programming language in the sense that we can break the program into parts using functions. So, it is easy to understand and modify. Functions also provide code reusability. ï‚· Rich Library C provides a lot of inbuilt functions that make the development fast. It is a diversified language with a rich set of built-in operators which are used in writing complex or simplified C programs. ï‚· Memory Management It supports the feature of dynamic memory allocation. In C language, we can free the allocated memory at any time by calling the free() function. ï‚· Speed The compilation and execution time of C language is fast since there are lesser inbuilt functions and hence the lesser overhead. Newer languages like java, python offer more features than c programming language but due to additional processing in these languages, their performance rate gets down effectively. C programming language as the been middle-level language provides programmers access to direct manipulation with the computer hardware but higher-level languages do not allow this. That’s one of the reasons C language is considered as the first choice to start learning programming languages. It’s fast because statically typed languages are faster than dynamically typed languages. ï‚· Extensible C language is extensible because it can easily adopt new features.Programs written in C language can be extended means when a program is already written in it then some more features and operations can be added into it. 2.2 STRUCTURE OF C PROGRAM Figure: Basic Structure of C Program Basically structure of the C program is divided into six different sections, 1. Documentation section: The Documentation section consists of a set of comment lines. Example 2. Link section: The link section provides instruction to the compiler to link the header files or functions from the system library. Example: #include 3. Definition section: The definition section defines all symbolic constants such by using the #define directive. Example: #define PI=3.14 4. Global declaration section: There are some variables that are used in more than one function, such variables are called global variables. Example: float area(float r); int a=7; In C there are two types of variable declaration, ï‚· Local variable declaration: Variables that are declared inside the ï‚· main function. Global variable declaration: Variables that are declared outside the main function. 5. Main function section: Every C-program should have one main() function. This section contains two parts: ï‚· Declaration part ï‚· Executable part Example: int main(void) { int a=10; printf(" %d", a); return 0; } 6. Sub-program section: If the program is a multi-function program, then the subprogram section contains all user-defined functions that are called in the main() function. Example: int add(int a, int b) { return a+b; } Sample Program The C program here will find the area of a circle using a user-defined function and a global variable pi holding the value of pi. #include//link section #define pi 3.14;//definition section float area(float r);//global declaration int main()//main function { float r; printf(" Enter the radius:n"); scanf("%f",&r); printf("the area is: %f",area(r)); return 0; } float area(float r) { return pi * r * r;//sub program } CHAPTER 2 FUNDAMENTALS OF C 2.3 COMMENTS There are two types of comment in C: 1) Single Line Comment 2) Multiline Comment 1. Single Line Comment A comment begins with // and extends up to the next line break. For example, // A Simple C Program #include int main(){ //printing information printf("Hello C"); return 0; } 2. Multi Line Comment: The comment lines start with. These statements can be put anywhere in the program. The compiler considers these as non-executable statements. The comment lines are included in a program to describe the variables used and the job performed by a set of program instructions or an instruction. For example, #include int main(){ printf("Hello C"); return 0; } 2.4 HEADER FILES A header file is a file with extension.h which contains C function declarations and macro definitions to be shared between several source files. There are two types of header files: the files that the programmer writes and the files that comes with your compiler. You request to use a header file in your program by including it with the C preprocessing directive #include, like you have seen inclusion of stdio.h header file, which comes along with your compiler. Including a header file is equal to copying the content of the header file but we do not do it because it will be error-prone and it is not a good idea to copy the content of a header file in the source files, especially if we have multiple source files in a program. A simple practice in C or C++ programs is that we keep all the constants, macros, system wide global variables, and function prototypes in the header files and include that header file wherever it is required. Syntax: #include OR #include "file" HOW INCLUDE WORKS? The C's #include preprocessor directive statement exertions by going through the C preprocessors for scanning any specific file like that of input before abiding by the rest of your existing source file. Let us take an example where you may think of having a header file karl.h having the following statement: Example: char *example (void); then, you have a main C source program which seems something like this: Example: #include int x; #include "karl.h" int main () { printf("Program done"); return 0; } So, the compiler will see the entire C program and token stream as: Example: #include int x; char * example (void); int main () { printf("Program done"); return 0; } There are some header files in practise as follows: NAME DESCRIPTION Input/Output functions Console input/output Mathematics functions Date & time functions String functions General Utility functions A set of functions for manipulating complex numbers Character handling functions Diagnostics functions 2.5 DATA TYPES Data types in c refer to an extensive system used for declaring variables or functions of different types. The type of a variable determines how much space it occupies in storage and how the bit pattern stored is interpreted. The types in C can be classified as follows − Sr.No. Types & Description 1 Basic Types They are arithmetic types and are further classified into: (a) integer types and (b) floating-point types. 2 Enumerated types They are again arithmetic types and they are used to define variables that can only assign certain discrete integer values throughout the program. 3 The type void The type specifier void indicates that no value is available. 4 Derived types They include (a) Pointer types, (b) Array types, (c) Structure types, (d) Union types and (e) Function types. The array types and structure types are referred collectively as the aggregate types. The type of a function specifies the type of the function's return value. We will see the basic types in the following section, where as other types will be covered in the upcoming chapters. Integer Types The following table provides the details of standard integer types with their storage sizes and value ranges − Type Storage size Value range char 1 byte -128 to 127 or 0 to 255 unsigned char 1 byte 0 to 255 signed char 1 byte -128 to 127 -32,768 to 32,767 or - int 2 or 4 bytes 2,147,483,648 to 2,147,483,647 unsigned int 2 or 4 bytes 0 to 65,535 or 0 to 4,294,967,295 short 2 bytes -32,768 to 32,767 unsigned short 2 bytes 0 to 65,535 long 8 bytes or (4bytes for 32 bit OS) -9223372036854775808 to 9223372036854775807 unsigned long 8 bytes 0 to 18446744073709551615 To get the exact size of a type or a variable on a particular platform, you can use the sizeof operator. The expressions sizeof(type) yields the storage size of the object or type in bytes. Floating-Point Types The following table provide the details of standard floating-point types with storage sizes and value ranges and their precision − Type Storage size Value range Precision float 4 byte 1.2E-38 to 3.4E+38 6 decimal places double 8 byte 2.3E-308 to 1.7E+308 15 decimal places long double 10 byte 3.4E-4932 to 1.1E+4932 19 decimal places The header file float.h defines macros that allow you to use these values and other details about the binary representation of real numbers in your programs. The void Type The void type specifies that no value is available. It is used in three kinds of situations − Sr.No. Types & Description 1 Function returns as void There are various functions in C which do not return any value or you can say they return void. A function with no return value has the return type as void. For example, void exit (int status); 2 Function arguments as void There are various functions in C which do not accept any parameter. A function with no parameter can accept a void. For example, int rand(void); 3 Pointers to void A pointer of type void * represents the address of an object, but not its type. For example, a memory allocation function void *malloc( size_t size ); returns a pointer to void which can be casted to any data type. 2.6 CONSTANTS & VARIABLES Variables In programming, a variable is a container (storage area) to hold data. To indicate the storage area, each variable should be given a unique name (identifier). Variable names are just the symbolic representation of a memory location. For example: int playerScore = 95; Here, playerScore is a variable of int type. Here, the variable is assigned an integer value 95. The value of a variable can be changed, hence the name variable. char ch = 'a'; // some code ch = 'l'; Rules for naming a variable A variable name can only have letters (both uppercase and lowercase letters), digits and underscore. The first letter of a variable should be either a letter or an underscore. There is no rule on how long a variable name (identifier) can be. However, you may run into problems in some compilers if the variable name is longer than 31 characters. Note: You should always try to give meaningful names to variables. For example: firstName is a better variable name than fn. C is a strongly typed language. This means that the variable type cannot be changed once it is declared. For example: int number = 5; // integer variable number = 5.5; // error double number; // error Here, the type of number variable is int. You cannot assign a floating-point (decimal) value 5.5 to this variable. Also, you cannot redefine the data type of the variable to double. By the way, to store the decimal values in C, you need to declare its type to either double or float. CONSTANTS If you want to define a variable whose value cannot be changed, you can use the const keyword. This will create a constant. For example, const double PI = 3.14; Notice, we have added keyword const. Here, PI is a symbolic constant; its value cannot be changed. const double PI = 3.14; PI = 2.9; //Error 2.7 OPERATORS An operator is a symbol that tells the compiler to perform specific mathematical or logical functions. C language is rich in built-in operators and provides the following types of operators − ï‚· Arithmetic Operators ï‚· Relational Operators ï‚· Logical Operators ï‚· ï‚· Bitwise Operators ï‚· Assignment Operators Misc Operators Arithmetic Operators The following table shows all the arithmetic operators supported by the C language. Assume variable A holds 10 and variable B holds 20 then − Operator Description Example + Adds two operands. A + B = 30 − Subtracts second operand from the first. A − B = -10 * Multiplies both operands. A*B= 200 / Divides numerator by de-numerator. B/A=2 % Modulus Operator and remainder of after an integer division. B%A=0 ++ Increment operator increases the integer value by one. A++ = 11 -- Decrement operator decreases the integer value by one. A-- = 9 Relational Operators The following table shows all the relational operators supported by C. Assume variable A holds 10 and variable B holds 20 then − Show Examples Operator Description Example == Checks if the values of two operands are equal or not. If yes, (A == B) is then the condition becomes true. not true. != Checks if the values of two operands are equal or not. If the (A != B) is values are not equal, then the condition becomes true. true. > Checks if the value of left operand is greater than the value (A > B) is of right operand. If yes, then the condition becomes true. not true. < Checks if the value of left operand is less than the value of (A < B) is right operand. If yes, then the condition becomes true. true. >= Checks if the value of left operand is greater than or equal to (A >= B) is the value of right operand. If yes, then the condition becomes not true. true. Array element reference Indirect member selection Left to right. Direct member selection ! Logical negation ~ Bitwise(1 's) complement + Unary plus - Unary minus ++ Increment -- Decrement Right to left & Dereference (Address) * Pointer reference sizeof Returns the size of an object (type) Typecast (conversion) * Multiply / Divide % Remainder Left to right + Binary plus(Addition) Left to right - Binary minus(subtraction) > Right shift Left to right < Less than Greater than Left to right >= Greater than or equal == Equal to != Not equal to Left to right & Bitwise AND ^ Bitwise exclusive OR Left to right | Bitwise OR Left to right && Logical AND Left to right || Logical OR Left to right ?: Conditional Operator Left to right = Simple assignment Right to left *= Assign product /= Assign quotient %= Assign remainder += Assign sum -= Assign difference &= Assign bitwise AND ^= Assign bitwise XOR Right to left |= Assign bitwise OR = Assign right shift , Separator of expressions Left to right IO Functions: The function by which we can give data to program is known as input function. With the use of Output function we can display our data on screen or we can take print out of it. C programming language provides many built-in functions to read any given input and to display data on screen when there is a need to output the result. All these built-in functions are present in C header files, we will also specify the name of header files in which a particular function is defined while discussing about it. scanf() and printf() functions: The standard input-output header file, named stdio.h contains the definition of the functions printf() and scanf(), which are used to display output on screen and to take input from user respectively. For example: #include void main() { // defining a variable int i; printf("Please enter a value..."); scanf("%d", &i); printf( "\nYou entered: %d", i); } getchar() & putchar() functions: The getchar() function reads a character from the terminal and returns it as an integer. This function reads only single character at a time. You can use this method in a loop in case you want to read more than one character. The putchar() function displays the character passed to it on the screen and returns the same character. This function too displays only a single character at a time. In case you want to display more than one characters, use putchar() method in a loop. #include void main( ) { int c; printf("Enter a character"); c = getchar(); putchar(c); } Difference between scanf() and gets() The main difference between these two functions is that scanf() stops reading characters when it encounters a space, but gets() reads space as character too. If you enter name as Study Tonight using scanf() it will only read and store Study and will leave the part after space. But gets() function will read it completely. For entering new equation, go to Insert ïƒ Quick Parts ïƒ Equation