ADSAA IMPORTANT QUESTIONS PDF
Document Details
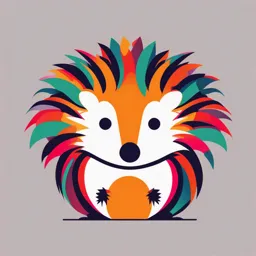
Uploaded by PortableSardonyx8044
Tags
Related
- Algorithm Design and Applications PDF
- Data Structures and Algorithm Analysis in C++ 4th Edition PDF
- MAULANA ABUL KALAM AZAD UNIVERSITY OF TECHNOLOGY, WEST BENGAL BCA 3rd Semester Data Structures and Algorithms Exam 2023-2024 PDF
- Sorting Algorithms PDF
- Algorithms and Data Structures 2 - Algorithm Analysis PDF
- Algorithms and Data Structures Lecture Notes (Winter 2024/25) PDF
Summary
This document contains a collection of important questions on data structures and algorithms, specifically geared towards undergraduate computer science students. The questions cover topics such as algorithm analysis, AVL trees, B-trees, and other essential computer science concepts.
Full Transcript
UNIT 1 2 Marks 1. Algorithm Analysis, Space and Time Complexity Analysis, Asymptotic Notations 1. Define time complexity and space complexity. 2. What is an asymptotic notation? 3. List the common types of asymptotic notations. 4...
UNIT 1 2 Marks 1. Algorithm Analysis, Space and Time Complexity Analysis, Asymptotic Notations 1. Define time complexity and space complexity. 2. What is an asymptotic notation? 3. List the common types of asymptotic notations. 4. State the difference between best-case and worst-case complexity. 5. Identify the Big-O complexity of the binary search algorithm. 1. Explain why analyzing an algorithm's complexity is important. 2. Differentiate between Ω (Omega) and O (Big-O) notations. 3. How does space complexity impact algorithm performance? 4. Illustrate the time complexity of linear search with an example. 5. Describe the role of input size in complexity analysis. 1. Calculate the time complexity of a given pseudocode snippet. 2. Apply the Big-O notation to classify a sorting algorithm. 3. Use an example to determine the space complexity of a recursive function. 4. Solve a problem to compute the worst-case scenario for a nested loop. 5. Demonstrate the use of Θ (Theta) notation in analyzing an algorithm. 1. Compare the space and time complexity of merge sort and quicksort. 2. Examine how loop nesting affects time complexity. 3. Analyze the trade-offs between time and space complexity. 4. Break down the time complexity of a divide-and-conquer algorithm. 5. Critique the impact of asymptotic analysis in real-world problem-solving. 1. Assess the scalability of an algorithm with O(n²) complexity. 2. Justify the choice of O(1) operations in optimizing a program. 3. Evaluate the efficiency of a given algorithm for large inputs. 4. Debate the importance of asymptotic analysis over experimental analysis. 5. Recommend a better algorithm based on complexity comparison. 2. AVL Trees – Creation, Insertion, Deletion Operations, and Applications 1. What is an AVL tree? 2. Define the balance factor in an AVL tree. 3. List the rotations used in AVL trees. 4. Identify the condition that makes an AVL tree unbalanced. 5. Recall the steps for inserting a node into an AVL tree. 1. Explain how AVL trees differ from binary search trees. 2. Why is rebalancing necessary in AVL trees? 3. Describe the purpose of rotations in AVL tree operations. 4. Illustrate with an example how the balance factor is calculated. 5. Explain how AVL trees improve search operations. 1. Insert a set of numbers into an AVL tree and show the result after each step. 2. Perform a deletion operation in an AVL tree and balance it. 3. Apply rotations to balance a given AVL tree. 4. Solve a problem to find the height of an AVL tree. 5. Demonstrate the creation of an AVL tree using provided data. 1. Compare the efficiency of AVL trees and binary search trees for search operations. 2. Analyze the impact of rotations on AVL tree performance. 3. Determine the conditions that require double rotations. 4. Examine the time complexity of AVL tree insertion and deletion. 5. Break down the sequence of operations in creating an AVL tree. 1. Assess the advantages of AVL trees in database indexing. 2. Justify the use of AVL trees in scenarios with frequent updates. 3. Evaluate the performance of AVL trees for large datasets. 4. Debate the importance of maintaining balance in AVL trees. 5. Recommend improvements to optimize AVL tree operations. 3. B-Trees – Creation, Insertion, Deletion Operations, and Applications 1. What is a B-tree? 2. Define the order of a B-tree. 3. List the properties of a B-tree. 4. State the purpose of a B-tree in database systems. 5. Identify the structure of a B-tree node. 1. Explain the difference between B-trees and binary search trees. 2. Why are B-trees suitable for disk-based storage systems? 3. Describe the process of splitting a node in a B-tree. 4. Illustrate how keys are distributed in a B-tree. 5. Explain how B-trees ensure balanced search operations. 1. Insert a sequence of numbers into a B-tree and display the resulting structure. 2. Perform a deletion operation in a B-tree and balance it. 3. Apply the splitting rule during a B-tree insertion. 4. Solve a problem to calculate the height of a B-tree. 5. Demonstrate how to search for a key in a B-tree. 1. Compare the efficiency of B-trees and AVL trees for search operations. 2. Analyze the time complexity of B-tree insertion and deletion. 3. Examine the impact of node splitting on B-tree performance. 4. Break down the structure of a B-tree after multiple insertions. 5. Determine the advantages of using B-trees for indexing. 1. Assess the performance of B-trees for large-scale data storage. 2. Justify the use of B-trees over binary search trees in databases. 3. Evaluate the trade-offs between B-tree height and node size. 4. Debate the importance of maintaining order in B-tree nodes. 5. Recommend modifications to optimize B-tree operations. Essay Type Questions 1. Algorithm Analysis, Space and Time Complexity Analysis, Asymptotic Notations 1. Define and describe the key terms used in algorithm analysis, such as time complexity, space complexity, and asymptotic notations. 2. Enumerate and explain the types of asymptotic notations, providing examples for each. 3. Write a detailed explanation of how best-case, worst-case, and average-case complexities are determined. 4. Discuss the importance of asymptotic notations in evaluating algorithm performance. 5. Outline the process of calculating time complexity for iterative and recursive algorithms. 1. Explain the significance of space complexity in modern computing applications with examples. 2. Discuss the differences between asymptotic notations (Big-O, Omega, and Theta) and their applications in algorithm analysis. 3. Provide an example of an algorithm and describe how its complexity changes with varying input sizes. 4. Explain how asymptotic analysis aids in comparing the efficiency of two algorithms solving the same problem. 5. Illustrate with examples how complexity analysis influences the choice of algorithms in practical scenarios. 1. Analyze the time complexity of a provided algorithm and explain the steps in your analysis. 2. Given a real-world problem (e.g., finding the shortest path), apply algorithm analysis to suggest an efficient solution. 3. Develop a recursive algorithm for a given problem and compute its time complexity. 4. Solve a problem involving nested loops and explain how you calculated its complexity. 5. Apply space complexity analysis to an algorithm and explain how memory usage can be optimized. 1. Compare the time and space complexities of different sorting algorithms and discuss their trade- offs. 2. Analyze a given algorithm to identify the factors that contribute most to its complexity. 3. Break down the process of calculating time complexity for a divide-and-conquer algorithm. 4. Critique the role of asymptotic analysis in designing algorithms for resource-constrained systems. 5. Examine the differences between experimental and theoretical analysis of algorithms with examples. 1. Evaluate the scalability of different algorithms for sorting large datasets and recommend the most efficient one. 2. Discuss the limitations of asymptotic notations in practical algorithm analysis and suggest improvements. 3. Assess the feasibility of using Θ(n log n) algorithms in real-time applications. 4. Justify the preference for algorithms with O(1) space complexity in certain scenarios. 5. Critically evaluate a given algorithm's performance based on its time and space complexity. 2. AVL Trees – Creation, Insertion, Deletion Operations, and Applications 1. Describe the structure and properties of an AVL tree in detail. 2. Write about the significance of balance factors and rotations in AVL trees. 3. Discuss the key steps involved in the insertion and deletion operations in AVL trees. 4. Define the types of rotations (LL, RR, LR, RL) used in AVL trees and explain their purposes. 5. Explain why AVL trees are considered self-balancing binary search trees. 1. Discuss the need for AVL trees over standard binary search trees with examples. 2. Explain how balancing is maintained in AVL trees through rotations. 3. Illustrate with diagrams how insertion of nodes can lead to rebalancing in an AVL tree. 4. Explain the advantages and limitations of AVL trees in real-world applications. 5. Discuss how deletion in an AVL tree differs from deletion in a binary search tree. 1. Perform the insertion of a set of keys into an AVL tree and explain the resulting structure after each step. 2. Solve a given problem using AVL trees and describe the steps involved in the process. 3. Apply AVL trees to a real-world problem, such as maintaining a leaderboard or priority queue. 4. Given a scenario where an AVL tree becomes unbalanced, demonstrate the steps to rebalance it. 5. Use AVL trees to solve a range query problem and explain the advantages of using them. 1. Compare AVL trees with binary search trees and red-black trees in terms of insertion, deletion, and search efficiency. 2. Analyze how AVL tree operations affect its height and overall efficiency. 3. Examine the trade-offs involved in maintaining balance in AVL trees. 4. Discuss the impact of frequent insertions and deletions on the performance of AVL trees. 5. Break down the process of balancing an unbalanced AVL tree with detailed examples. 1. Evaluate the use of AVL trees in applications requiring frequent updates and justify their efficiency. 2. Assess the performance of AVL trees for large datasets compared to other data structures. 3. Justify the use of AVL trees in applications like database indexing and real-time systems. 4. Critically analyze the limitations of AVL trees and propose scenarios where alternative structures are preferable. 5. Debate whether the overhead of balancing in AVL trees is justified for all use cases. 3. B-Trees – Creation, Insertion, Deletion Operations, and Applications 1. Define a B-tree and describe its key properties and structure. 2. Explain the purpose of node splitting in B-trees during insertion operations. 3. List the advantages of B-trees over binary search trees for large datasets. 4. Write the steps involved in the deletion operation in B-trees. 5. Describe the significance of the order of a B-tree. 1. Discuss the differences between B-trees and AVL trees, with examples. 2. Explain why B-trees are widely used in database systems and file storage. 3. Illustrate the process of inserting and balancing nodes in a B-tree with a detailed example. 4. Discuss the role of B-trees in reducing disk I/O operations. 5. Explain the impact of increasing the order of a B-tree on its structure and performance. 1. Insert a given sequence of keys into a B-tree and describe the resulting structure after each operation. 2. Apply B-tree deletion operations to a given dataset and explain the changes step by step. 3. Solve a database indexing problem using B-trees and outline the steps involved. 4. Demonstrate how B-trees manage large datasets in external storage efficiently. 5. Use a B-tree to solve a real-world problem like maintaining a directory or a file system. 1. Compare the performance of B-trees and binary search trees for search and update operations. 2. Analyze the impact of node splitting and merging on the efficiency of B-tree operations. 3. Examine the trade-offs between height and branching factor in B-trees. 4. Discuss the implications of B-tree height on the speed of search operations. 5. Break down the process of managing overflow and underflow conditions in B-trees. 1. Evaluate the suitability of B-trees for applications with frequent read and write operations. 2. Justify the choice of B-trees in database indexing compared to other self-balancing trees. 3. Assess the scalability of B-trees for extremely large datasets. 4. Critically analyze the limitations of B-trees and suggest alternative structures for specific scenarios. 5. Debate the effectiveness of B-trees in minimizing disk I/O and improving performance. UNIT 2 2 Marks 1. Heap Trees (Priority Queues): Min and Max Heaps, Operations, and Applications 1. Define a min-heap and a max-heap. 2. What are the basic properties of a heap tree? 3. List the key operations performed on a priority queue. 4. State the time complexity of insertion and deletion in a binary heap. 5. Identify some real-world applications of heaps. 1. Explain the difference between a min-heap and a max-heap. 2. Why are heap trees suitable for implementing priority queues? 3. Describe the process of heapifying a binary tree. 4. Illustrate with an example how elements are arranged in a max-heap. 5. Explain how heaps can be used in finding the K largest elements in a dataset. 1. Build a max-heap from the array [10, 20, 15, 30, 40] and show the result. 2. Perform an insertion into a min-heap and adjust the heap. 3. Use a heap to implement a priority queue for a task scheduling problem. 4. Solve a problem to find the smallest element in a max-heap. 5. Demonstrate how heapsort works using a given array. 1. Compare heapsort with other sorting algorithms in terms of time and space complexity. 2. Examine how the heap structure changes during the deletion of the root element. 3. Analyze the role of heapify in maintaining heap properties. 4. Discuss the advantages of binary heaps over arrays in priority queues. 5. Break down the operations of a priority queue implemented using a heap. 1. Evaluate the suitability of heaps for real-time systems requiring fast access to priorities. 2. Justify the use of heaps in Dijkstra’s shortest path algorithm. 3. Assess the limitations of heaps compared to other data structures for specific applications. 4. Debate the advantages of min-heaps over max-heaps in particular scenarios. 5. Recommend a scenario where heap-based sorting is the best choice. 2. Graphs: Terminology, Representations, Basic Search and Traversals, Connected and Biconnected Components, Applications 1. Define the terms vertex, edge, and degree in a graph. 2. What is an adjacency matrix? 3. List the basic graph traversal techniques. 4. What are connected and biconnected components in a graph? 5. Identify applications of graph theory in real-world problems. 1. Explain the differences between a directed and an undirected graph. 2. How does an adjacency list differ from an adjacency matrix? 3. Describe the purpose of depth-first search (DFS) and breadth-first search (BFS). 4. Illustrate with an example how connected components are identified in a graph. 5. Explain the significance of biconnected components in network reliability. 1. Represent the given graph using an adjacency list and adjacency matrix. 2. Use BFS to find the shortest path in an unweighted graph. 3. Solve a problem to identify connected components in a graph. 4. Apply DFS to determine articulation points in a graph. 5. Demonstrate how graphs can be used in modeling a social network. 1. Compare adjacency matrix and adjacency list in terms of storage and performance. 2. Analyze the time complexity of DFS and BFS for graph traversal. 3. Examine how biconnected components are identified using DFS. 4. Discuss the importance of graph traversal techniques in solving connectivity problems. 5. Break down the process of finding articulation points in a graph. 1. Evaluate the use of DFS over BFS for certain graph problems. 2. Assess the efficiency of different graph representations for dense and sparse graphs. 3. Justify the choice of graph algorithms in network routing and optimization problems. 4. Critically evaluate the limitations of BFS in weighted graphs. 5. Recommend graph traversal methods for a specific real-world application. 3. Divide and Conquer: The General Method, Quick Sort, Merge Sort, Strassen’s Matrix Multiplication, Convex Hull 1. Define the divide-and-conquer approach in algorithm design. 2. What is the recurrence relation for merge sort? 3. List the key steps in quick sort. 4. State the main advantage of Strassen’s matrix multiplication algorithm. 5. What is the convex hull problem in computational geometry? 1. Explain how divide-and-conquer reduces problem-solving complexity. 2. Describe the difference between merge sort and quick sort with examples. 3. Illustrate how Strassen’s algorithm multiplies matrices with fewer operations. 4. Explain why the convex hull problem is important in computational geometry. 5. Discuss the advantages of divide-and-conquer over brute-force methods. 1. Apply merge sort to a given array and explain the steps. 2. Perform quick sort on a list and show the recursive process. 3. Solve a matrix multiplication problem using Strassen’s algorithm. 4. Use divide-and-conquer to find the closest pair of points in a plane. 5. Construct the convex hull for a given set of points using the divide-and-conquer method. 1. Compare the time complexities of quick sort and merge sort. 2. Analyze the impact of pivot selection on quick sort’s performance. 3. Discuss how Strassen’s algorithm improves matrix multiplication efficiency. 4. Examine the challenges of implementing divide-and-conquer algorithms for large datasets. 5. Analyze the time complexity of finding a convex hull using the divide-and-conquer approach. 1. Evaluate the use of merge sort in scenarios where memory usage is a concern. 2. Justify the choice of quick sort for small datasets over other sorting methods. 3. Assess the trade-offs between classical and Strassen’s matrix multiplication algorithms. 4. Critique the divide-and-conquer approach for solving computational geometry problems. 5. Recommend an efficient divide-and-conquer algorithm for a specific application. Essay Type Questions 1. Heap Trees (Priority Queues): Min and Max Heaps, Operations, and Applications 1. Define heap trees, highlighting the structure and key properties of min-heaps and max-heaps. 2. Write a detailed explanation of the insertion and deletion operations in a binary heap. 3. Enumerate the advantages of using heap trees for priority queue implementations. 4. Explain the significance of heapify in maintaining the heap structure. 5. Describe some real-world applications of priority queues using heaps. 1. Explain the differences between a binary heap and other tree-based data structures like binary search trees. 2. Discuss the role of heap trees in optimizing algorithms like heapsort and Dijkstra's shortest path. 3. Illustrate with an example how the heap property is maintained during an insertion operation. 4. Describe the process of converting an unsorted array into a min-heap. 5. Explain how heap trees are used to solve the Kth smallest or largest element problems efficiently. 1. Demonstrate with an example how a priority queue is implemented using a heap tree for a real- world scenario. 2. Write a program that constructs a max-heap from a given array and explains each step. 3. Apply the concept of heapify to solve a scheduling problem involving task priorities. 4. Solve a problem where a heap is used to merge multiple sorted arrays. 5. Use heap-based algorithms to solve a real-world problem such as resource allocation or task management. 1. Compare the performance of min-heaps and max-heaps in priority queue operations, including trade-offs. 2. Analyze the complexity of heapify and its impact on building a heap from an array. 3. Discuss the differences between binary heaps and Fibonacci heaps, with examples of applications. 4. Examine the role of heap operations in the efficiency of heapsort and other algorithms. 5. Break down how a heap can be used to efficiently find the median in a stream of numbers. 1. Evaluate the use of heap trees in applications requiring dynamic priority adjustments. 2. Assess the suitability of heaps for implementing job scheduling systems in real-time environments. 3. Discuss the limitations of binary heaps and suggest scenarios where they might not be ideal. 4. Justify the use of heap-based priority queues over other data structures like balanced trees. 5. Critically analyze a real-world application of heaps and propose enhancements to improve efficiency. 2. Graphs: Terminology, Representations, Basic Search and Traversals, Connected and Biconnected Components, Applications 1. Define the key terms in graph theory, including vertex, edge, degree, and path, with examples. 2. Write a detailed explanation of adjacency matrix and adjacency list representations of graphs. 3. Enumerate the steps of depth-first search (DFS) and breadth-first search (BFS). 4. Explain the concepts of connected components and biconnected components in graphs. 5. List real-world applications of graph theory and briefly describe their relevance. 1. Discuss the differences between directed and undirected graphs with suitable examples. 2. Explain why BFS is preferred over DFS in certain applications, such as finding the shortest path. 3. Illustrate with an example how connected components in a graph are identified using DFS. 4. Describe the importance of graph traversal techniques in solving real-world problems like navigation. 5. Explain the role of articulation points and bridges in determining biconnected components. 1. Represent a real-world network (e.g., a social network or a city map) as a graph and perform BFS on it. 2. Solve a problem where you use DFS to identify all connected components in a graph. 3. Apply graph traversal algorithms to determine the articulation points in a communication network. 4. Use graph theory to model and solve a logistics optimization problem. 5. Write a program to find all biconnected components in a graph and explain the steps. 1. Compare adjacency matrix and adjacency list representations of graphs, highlighting their trade- offs. 2. Analyze the time complexity of DFS and BFS and discuss their impact on large graphs. 3. Discuss the importance of identifying connected and biconnected components in network reliability. 4. Examine the differences between graph traversal methods in terms of their applications. 5. Analyze the efficiency of graph representations and traversals for sparse and dense graphs. 1. Evaluate the suitability of DFS and BFS for solving problems in graph theory, with examples. 2. Assess the limitations of graph algorithms in handling extremely large datasets. 3. Justify the use of graph-based models for real-world problems like social network analysis. 4. Critically analyze the performance of graph traversal techniques in detecting articulation points. 5. Debate the relevance of connected and biconnected components in optimizing network design. 3. Divide and Conquer: The General Method, Quick Sort, Merge Sort, Strassen’s Matrix Multiplication, Convex Hull 1. Define the divide-and-conquer approach in algorithm design, providing key characteristics. 2. Write detailed steps of the merge sort algorithm and explain its working. 3. State the recurrence relation for quick sort and analyze its time complexity. 4. Describe the key steps of Strassen’s matrix multiplication and its advantages. 5. What is the convex hull problem, and why is it significant in computational geometry? 1. Discuss the advantages of divide-and-conquer over brute-force methods. 2. Explain the differences between merge sort and quick sort in terms of their design and performance. 3. Illustrate with examples how Strassen’s algorithm reduces the complexity of matrix multiplication. 4. Describe the role of divide-and-conquer in solving geometric problems like the convex hull. 5. Explain why pivot selection is crucial for the efficiency of quick sort. 1. Apply the divide-and-conquer method to sort a given array using merge sort. 2. Perform quick sort on an array and explain the recursive steps involved. 3. Solve a problem involving matrix multiplication using Strassen’s algorithm. 4. Use the divide-and-conquer approach to find the convex hull of a set of points. 5. Solve a problem to find the closest pair of points in a plane using divide-and-conquer. 1. Compare the time complexities of merge sort, quick sort, and heapsort. 2. Analyze the impact of poor pivot selection on the performance of quick sort. 3. Discuss the trade-offs of using Strassen’s algorithm for matrix multiplication. 4. Examine the efficiency of divide-and-conquer in solving problems of various sizes and complexities. 5. Analyze the challenges of implementing divide-and-conquer algorithms for multidimensional data. 1. Evaluate the performance of merge sort in memory-constrained environments. 2. Justify the use of quick sort for small datasets over other sorting algorithms. 3. Critically evaluate the limitations of Strassen’s algorithm in practical applications. 4. Debate the suitability of divide-and-conquer for solving computational geometry problems. 5. Assess the effectiveness of the convex hull algorithm in real-world applications. Unit 3 2 Marks 1. Greedy Method: General Method, Job Sequencing with Deadlines, Knapsack Problem, Minimum Cost Spanning Trees, Single Source Shortest Paths 1. Define the greedy method and state its key characteristic. 2. What is the job sequencing with deadlines problem? 3. List the main steps of Kruskal’s algorithm for minimum cost spanning trees. 4. State the formula for the fractional knapsack problem. 5. What is the single-source shortest path problem? 1. Explain the greedy choice property with an example. 2. Describe how the greedy approach is applied in job sequencing with deadlines. 3. Why is Prim’s algorithm suitable for dense graphs in minimum cost spanning trees? 4. Illustrate the difference between fractional and 0/1 knapsack problems. 5. Explain the significance of edge relaxation in Dijkstra’s algorithm. 1. Solve a fractional knapsack problem for a given set of weights and profits. 2. Use Kruskal’s algorithm to construct a minimum spanning tree for a sample graph. 3. Apply the greedy method to schedule jobs with deadlines and maximize profit. 4. Find the shortest path from a source vertex to all other vertices using Dijkstra’s algorithm. 5. Demonstrate how Prim’s algorithm constructs a spanning tree step by step. 1. Compare Kruskal’s and Prim’s algorithms in terms of time complexity and applications. 2. Analyze the conditions under which the greedy method provides an optimal solution. 3. Examine the trade-offs between fractional and 0/1 knapsack problems. 4. Discuss the limitations of Dijkstra’s algorithm for graphs with negative weights. 5. Break down the steps of job sequencing and identify scenarios where the greedy method fails. 1. Evaluate the performance of the greedy method for solving the minimum cost spanning tree problem. 2. Justify the use of Kruskal’s algorithm for sparse graphs. 3. Assess the greedy approach for solving the fractional knapsack problem in terms of optimality. 4. Debate the limitations of using greedy algorithms in single-source shortest path problems. 5. Recommend scenarios where the greedy method is more efficient than dynamic programming. 2. Dynamic Programming: General Method, All Pairs Shortest Paths, Single Source Shortest Paths (Bellman- Ford Algorithm), Optimal Binary Search Trees, 0/1 Knapsack, String Editing, Travelling Salesperson Problem 1. Define dynamic programming and state its primary principle. 2. What is the Bellman-Ford algorithm used for? 3. List the conditions under which dynamic programming is applicable. 4. What is the 0/1 knapsack problem? 5. Define the travelling salesperson problem (TSP). 1. Explain the principle of optimality in dynamic programming with an example. 2. Describe how the Bellman-Ford algorithm handles negative edge weights. 3. Why is dynamic programming suitable for solving the 0/1 knapsack problem? 4. Illustrate how the optimal binary search tree minimizes search costs. 5. Explain the significance of dynamic programming in solving the TSP. 1. Solve a 0/1 knapsack problem using the dynamic programming approach. 2. Apply the Bellman-Ford algorithm to find the shortest paths in a graph with negative weights. 3. Use dynamic programming to calculate the minimum cost of matrix chain multiplication. 4. Solve a string editing problem to convert one string into another with minimum operations. 5. Demonstrate the dynamic programming approach to solve the TSP for four cities. 1. Compare the Bellman-Ford algorithm and Dijkstra’s algorithm in terms of efficiency and applicability. 2. Analyze the time complexity of dynamic programming for the 0/1 knapsack problem. 3. Discuss the trade-offs between greedy and dynamic programming approaches for TSP. 4. Examine how the cost matrix is updated iteratively in the all-pairs shortest path problem. 5. Break down the steps of constructing an optimal binary search tree and analyze its efficiency. 1. Evaluate the use of dynamic programming in solving real-world optimization problems. 2. Justify the choice of Bellman-Ford over Dijkstra’s algorithm for graphs with negative weights. 3. Assess the limitations of dynamic programming in solving large-scale problems like TSP. 4. Debate the efficiency of dynamic programming versus greedy algorithms for the 0/1 knapsack problem. 5. Critique the dynamic programming approach to string editing and propose alternatives. Essay Type questions 1. Greedy Method: General Method, Job Sequencing with Deadlines, Knapsack Problem, Minimum Cost Spanning Trees, Single Source Shortest Paths 1. Define the greedy method and explain its key characteristics with examples. 2. Write an essay on the steps involved in solving the job sequencing with deadlines problem. 3. Describe the process of constructing minimum cost spanning trees using Kruskal’s and Prim’s algorithms. 4. Explain the steps of the fractional knapsack problem using the greedy approach. 5. State and describe Dijkstra’s algorithm for solving the single-source shortest path problem. 1. Explain the greedy choice property and optimal substructure in the context of greedy algorithms. 2. Discuss the importance of deadlines in the job sequencing problem and how they affect scheduling. 3. Illustrate with examples how Kruskal’s and Prim’s algorithms differ in constructing spanning trees. 4. Analyze why the greedy approach is suitable for fractional knapsack but not for 0/1 knapsack. 5. Explain the role of edge relaxation in Dijkstra’s algorithm with a detailed example. 1. Apply the greedy method to solve a job sequencing problem with given deadlines and profits. 2. Solve a fractional knapsack problem using a greedy approach and discuss each step. 3. Write a detailed application of Kruskal’s algorithm to construct a minimum spanning tree for a weighted graph. 4. Solve a single-source shortest path problem using Dijkstra’s algorithm and explain the process. 5. Demonstrate the application of greedy algorithms in a real-world problem, such as network design or resource allocation. 1. Compare Kruskal’s and Prim’s algorithms for minimum cost spanning trees, highlighting their advantages and limitations. 2. Analyze the trade-offs between using greedy and dynamic programming approaches for solving the knapsack problem. 3. Break down the steps of job sequencing with deadlines and discuss scenarios where it may fail. 4. Examine the limitations of Dijkstra’s algorithm for graphs with negative edge weights. 5. Discuss the time complexity of greedy algorithms and its impact on large-scale problem-solving. 1. Evaluate the performance of greedy algorithms in solving optimization problems like spanning trees and knapsack. 2. Justify the use of Kruskal’s algorithm for sparse graphs and Prim’s algorithm for dense graphs. 3. Assess the limitations of greedy methods in solving the job sequencing problem with complex constraints. 4. Critique the greedy approach to the single-source shortest path problem and propose alternatives. 5. Debate the effectiveness of greedy algorithms for solving real-world problems compared to dynamic programming. 2. Dynamic Programming: General Method, All Pairs Shortest Paths, Single Source Shortest Paths (Bellman- Ford Algorithm), Optimal Binary Search Trees, 0/1 Knapsack, String Editing, Travelling Salesperson Problem 1. Define dynamic programming and explain its principle of optimality. 2. Write an essay on the Bellman-Ford algorithm, describing its steps and applications. 3. Describe the 0/1 knapsack problem and how it differs from the fractional knapsack problem. 4. Explain the Travelling Salesperson Problem (TSP) and its significance in optimization. 5. State the recurrence relations used in solving the all-pairs shortest path problem using dynamic programming. 1. Explain how dynamic programming differs from the greedy method, with examples. 2. Discuss the concept of overlapping subproblems and optimal substructure in dynamic programming. 3. Describe the role of dynamic programming in solving the string editing problem, with examples. 4. Illustrate the construction of an optimal binary search tree and its applications. 5. Explain the Bellman-Ford algorithm’s handling of graphs with negative edge weights. 1. Solve a 0/1 knapsack problem using dynamic programming and explain the steps in detail. 2. Use the Bellman-Ford algorithm to solve a single-source shortest path problem in a weighted graph. 3. Apply dynamic programming to solve the all-pairs shortest path problem using Floyd-Warshall’s algorithm. 4. Solve a Travelling Salesperson Problem for a given dataset and explain the dynamic programming approach. 5. Write a detailed example of string editing to transform one string into another using dynamic programming. 1. Compare Dijkstra’s and Bellman-Ford algorithms for solving single-source shortest path problems. 2. Analyze the time complexity of dynamic programming solutions for TSP and knapsack problems. 3. Discuss the advantages and limitations of using dynamic programming for string editing. 4. Examine the trade-offs in constructing optimal binary search trees for different datasets. 5. Break down the steps of the Bellman-Ford algorithm and analyze its suitability for large graphs. 1. Evaluate the effectiveness of dynamic programming in solving complex optimization problems. 2. Justify the use of Bellman-Ford over Dijkstra’s algorithm for graphs with negative weights. 3. Assess the limitations of dynamic programming in solving large-scale Travelling Salesperson Problems. 4. Debate the efficiency of dynamic programming versus greedy algorithms for the 0/1 knapsack problem. 5. Critique the dynamic programming approach to constructing optimal binary search trees and propose improvements. Unit 4 2 Marks Backtracking: General Method, 8-Queens Problem, Sum of Subsets Problem, Graph Coloring, 0/1 Knapsack Problem 1. Define backtracking and state its primary principle. 2. What is the 8-Queens problem? 3. State the criteria for a valid solution in the graph coloring problem. 4. What is the sum of subsets problem? 5. List the differences between backtracking and brute force methods. 1. Explain the concept of a solution space in backtracking with an example. 2. Why does backtracking work well for the 8-Queens problem? 3. Describe how backtracking is applied to solve the sum of subsets problem. 4. Explain the significance of constraints in the graph coloring problem. 5. Why is backtracking suitable for solving the 0/1 knapsack problem? 1. Write the state-space tree for a 4-Queens problem using backtracking. 2. Solve a graph coloring problem with a given graph and explain the steps. 3. Use backtracking to solve a sum of subsets problem with a specific example. 4. Demonstrate how backtracking handles the constraints of the 8-Queens problem. 5. Apply backtracking to solve a small 0/1 knapsack problem. 1. Compare the performance of backtracking and dynamic programming in solving the 0/1 knapsack problem. 2. Break down the steps involved in solving the 8-Queens problem using backtracking. 3. Analyze the time complexity of solving the graph coloring problem using backtracking. 4. Examine the limitations of backtracking when applied to large-scale sum of subsets problems. 5. Discuss the challenges of constructing the state-space tree for the 0/1 knapsack problem. 1. Evaluate the efficiency of backtracking for solving constraint satisfaction problems like 8- Queens. 2. Justify the use of backtracking for solving graph coloring problems over heuristic methods. 3. Assess the limitations of backtracking in solving real-world combinatorial problems. 4. Debate whether backtracking is the optimal choice for the sum of subsets problem. 5. Critique the use of backtracking in solving the 0/1 knapsack problem and suggest improvements. 2. Branch and Bound: General Method, 0/1 Knapsack Problem, Travelling Salesperson Problem 1. Define the branch and bound method. 2. What is the bounding function in branch and bound? 3. State the principle behind solving the 0/1 knapsack problem using branch and bound. 4. What does the term "live node" mean in the branch and bound method? 1. Explain the difference between backtracking and branch and bound. 2. Why is a bounding function critical in branch and bound? 3. Describe how branch and bound is used to solve the 0/1 knapsack problem. 4. Explain the significance of pruning in reducing the search space in branch and bound. 5. Why is branch and bound suitable for solving the TSP? 1. Solve a 0/1 knapsack problem using branch and bound with a given set of weights and profits. 2. Write the steps for solving a TSP using branch and bound with a sample graph. 3. Use branch and bound to solve a small-scale combinatorial optimization problem. 4. Construct a bounding function for a given instance of the 0/1 knapsack problem. 5. Demonstrate how live and dead nodes are processed in a branch and bound solution tree. 1. Compare the effectiveness of branch and bound versus backtracking for solving the 0/1 knapsack problem. 2. Break down the steps of solving TSP using branch and bound and analyze its time complexity. 3. Discuss the impact of the bounding function on the efficiency of branch and bound. 4. Analyze the role of pruning in optimizing the branch and bound method for TSP. 5. Examine the trade-offs between branch and bound and dynamic programming for solving optimization problems. 1. Evaluate the suitability of branch and bound for solving large-scale 0/1 knapsack problems. 2. Justify the use of branch and bound for TSP over heuristic approaches. 3. Assess the limitations of branch and bound in solving highly constrained problems. 4. Debate whether branch and bound is the best choice for solving the 0/1 knapsack problem. 5. Critique the efficiency of branch and bound for real-world applications and suggest modifications. Essay Type Questions Backtracking: General Method, 8-Queens Problem, Sum of Subsets Problem, Graph Coloring, 0/1 Knapsack Problem 1. Define backtracking and explain its general method with examples. 2. Write an essay describing the state-space tree and its role in solving backtracking problems. 3. Outline the steps involved in solving the 8-Queens problem using backtracking. 4. Describe the sum of subsets problem and state the conditions for a valid solution. 5. Write an essay on the key features and steps involved in solving the graph coloring problem. 1. Explain how the backtracking approach ensures an efficient solution to the 8-Queens problem. 2. Discuss how constraints in the sum of subsets problem guide the search process in backtracking. 3. Describe the significance of the solution space and pruning in backtracking algorithms. 4. Explain the challenges of using backtracking for graph coloring in large graphs. 5. Illustrate the steps involved in solving the 0/1 knapsack problem using backtracking. 1. Write a detailed solution for a 4-Queens problem using backtracking, including the construction of the state-space tree. 2. Apply backtracking to solve a sample sum of subsets problem and explain the steps involved. 3. Solve a graph coloring problem for a given graph using backtracking, and describe the process. 4. Demonstrate how backtracking is used to solve a constrained version of the 0/1 knapsack problem. 5. Write an essay applying backtracking to solve a real-world problem, such as sudoku or scheduling. 1. Compare the backtracking and brute force approaches in terms of efficiency and applicability. 2. Analyze the challenges of solving the 8-Queens problem for larger board sizes using backtracking. 3. Discuss the role of constraint satisfaction in solving graph coloring problems using backtracking. 4. Examine the limitations of backtracking in solving large-scale sum of subsets problems. 5. Analyze the computational complexity of using backtracking for the 0/1 knapsack problem. 1. Evaluate the effectiveness of backtracking in solving combinatorial optimization problems. 2. Justify the use of backtracking for the graph coloring problem over heuristic methods. 3. Assess the feasibility of using backtracking for solving high-dimensional sum of subsets problems. 4. Debate whether backtracking is the best choice for solving the 0/1 knapsack problem, and suggest alternatives. 5. Critique the use of backtracking for solving the 8-Queens problem and propose optimizations. 2. Branch and Bound: General Method, 0/1 Knapsack Problem, Travelling Salesperson Problem (TSP) 1. Define the branch and bound method and explain its core principles. 2. Describe the importance of the bounding function in the branch and bound method. 3. Write an essay on the steps involved in solving the 0/1 knapsack problem using branch and bound. 4. Explain the terms "live node" and "dead node" in the branch and bound method. 5. Describe the general structure of a branch and bound solution tree. 1. Explain how the bounding function impacts the efficiency of the branch and bound method. 2. Discuss the differences between backtracking and branch and bound in solving optimization problems. 3. Illustrate the steps of solving the TSP using branch and bound with a simple example. 4. Describe the role of pruning in reducing the computational overhead in branch and bound. 5. Explain how branch and bound is applied to solve the 0/1 knapsack problem. 1. Solve a sample 0/1 knapsack problem using branch and bound, detailing each step. 2. Apply branch and bound to solve a small-scale TSP and write an essay on the process. 3. Construct a bounding function for a given instance of the knapsack problem and demonstrate its use. 4. Write a detailed application of branch and bound to a resource allocation problem. 5. Apply the branch and bound method to solve a constrained version of TSP. 1. Compare the effectiveness of branch and bound versus backtracking for solving the 0/1 knapsack problem. 2. Analyze the computational complexity of branch and bound in solving TSP for increasing numbers of cities. 3. Examine the impact of pruning on the efficiency of branch and bound for large problem spaces. 4. Discuss the challenges of constructing effective bounding functions for the 0/1 knapsack problem. 5. Break down the limitations of branch and bound in solving real-world optimization problems. 1. Evaluate the feasibility of using branch and bound for solving large-scale TSP instances. 2. Assess the trade-offs between branch and bound and dynamic programming for the 0/1 knapsack problem. 3. Justify the use of branch and bound for solving combinatorial problems over heuristic methods. 4. Critique the efficiency of branch and bound for solving highly constrained problems and propose alternatives. 5. Debate the effectiveness of branch and bound for real-world applications like vehicle routing. UNIT 5 2 Marks 1. NP Hard and NP Complete Problems: Basic Concepts, Cook’s Theorem 1. Define NP-hard and NP-complete problems. 2. What is Cook’s theorem? 3. List some examples of NP-complete problems. 4. State the relationship between P, NP, and NP-complete classes. 5. What is the significance of the term "polynomial-time reducibility"? 1. Explain the difference between NP-hard and NP-complete problems. 2. Why is Cook’s theorem considered a cornerstone in computational complexity theory? 3. Describe the role of non-determinism in NP problems. 4. Explain why NP-complete problems are a subset of NP problems. 5. Discuss how polynomial-time reducibility is used to classify problems as NP-complete. 1. Apply Cook’s theorem to explain why the Boolean satisfiability problem (SAT) is NP-complete. 2. Write an example of how a problem is reduced to SAT to prove its NP-completeness. 3. Demonstrate how the traveling salesperson problem can be framed as a decision problem. 4. Solve a small example to show how a reduction between two NP problems works. 5. Construct a graph problem and explain its classification as NP-hard or NP-complete. 1. Compare NP-hard and NP-complete problems in terms of their computational complexity. 2. Analyze the implications of Cook’s theorem on modern cryptography. 3. Discuss the importance of reductions in understanding the hardness of computational problems. 4. Break down the proof structure of Cook’s theorem into its main components. 5. Examine why solving any NP-complete problem in polynomial time implies P = NP. 1. Evaluate the practical significance of studying NP-hard problems in computer science. 2. Assess the role of Cook’s theorem in computational complexity research. 3. Justify the classification of SAT as an NP-complete problem. 4. Debate the likelihood of proving P = NP based on current knowledge. 5. Critique the use of reductions in proving NP-completeness, highlighting their strengths and weaknesses. 2. NP-Hard Graph Problems: Clique Decision Problem (CDP), Chromatic Number Decision Problem (CNDP), Travelling Salesperson Decision Problem (TSP) 1. What is the Clique Decision Problem (CDP)? 2. Define the Chromatic Number Decision Problem (CNDP). 3. What is the Travelling Salesperson Decision Problem (TSP)? 4. List the characteristics of NP-hard graph problems. 5. What is the significance of the term "decision problem" in NP-hard classifications? 1. Explain why CDP is considered NP-hard. 2. Describe the role of graph coloring in CNDP and its computational complexity. 3. Why is TSP classified as NP-hard despite having approximate solutions? 4. Discuss the relationship between CDP and other graph problems in terms of complexity. 5. Explain the significance of reducing graph problems to decision problems in complexity theory. 1. Write a small example graph and determine if it satisfies the conditions of CDP. 2. Solve a sample CNDP problem for a simple graph and explain the steps. 3. Apply the decision version of TSP to a graph with 4 vertices and demonstrate the process. 4. Construct an example showing how CDP can be reduced to CNDP. 5. Write a sample reduction of a general graph problem to CDP or CNDP. 1. Compare CDP and CNDP in terms of their computational challenges. 2. Analyze the structure of TSP as a decision problem and its implications for real-world applications. 3. Break down the role of adjacency matrices in solving CDP for dense graphs. 4. Discuss the implications of approximations for solving NP-hard graph problems. 5. Analyze how reductions between graph problems demonstrate their NP-hardness. 1. Evaluate the real-world significance of solving CDP in network design. 2. Justify the classification of CNDP as an NP-hard problem. 3. Assess the challenges of exact solutions for TSP in logistics and routing. 4. Critique the practicality of exact solutions versus approximations for NP-hard graph problems. 5. Debate whether heuristic methods are sufficient for solving NP-hard graph problems in practice. 3. NP-Hard Scheduling Problems: Scheduling Identical Processors, Job Shop Scheduling 1. What is the Scheduling Identical Processors problem? 2. Define the Job Shop Scheduling problem. 3. List the constraints typically associated with NP-hard scheduling problems. 4. What does it mean for a scheduling problem to be classified as NP-hard? 5. State the basic characteristics of the Job Shop Scheduling problem. 1. Explain why Scheduling Identical Processors is classified as NP-hard. 2. Describe the challenges of sequencing tasks in Job Shop Scheduling. 3. Discuss the impact of resource constraints on NP-hard scheduling problems. 4. Explain how scheduling problems are represented as decision problems. 5. Illustrate the significance of job precedence constraints in Job Shop Scheduling. 1. Solve a simple example of the Scheduling Identical Processors problem with three processors and five tasks. 2. Write a solution for a two-machine Job Shop Scheduling problem with four jobs. 3. Apply a heuristic to solve a small-scale Scheduling Identical Processors problem and explain the process. 4. Demonstrate the importance of resource allocation in solving Job Shop Scheduling problems. 5. Create a scheduling example and classify it as NP-hard based on its constraints. 1. Compare the complexities of Scheduling Identical Processors and Job Shop Scheduling. 2. Analyze the role of constraints in increasing the complexity of scheduling problems. 3. Discuss the trade-offs between exact and approximate methods for Job Shop Scheduling. 4. Examine the challenges of representing scheduling problems as decision problems. 5. Break down the computational requirements for solving Scheduling Identical Processors optimally. 1. Evaluate the effectiveness of heuristics in solving Scheduling Identical Processors problems. 2. Justify the use of NP-hard classifications for Job Shop Scheduling problems. 3. Assess the practicality of solving real-world scheduling problems using exact methods. 4. Critique the scalability of solutions for NP-hard scheduling problems in manufacturing. 5. Debate whether approximate solutions are sufficient for solving complex scheduling problems. Essay Type Questions 1. NP Hard and NP Complete Problems: Basic Concepts, Cook’s Theorem 1. Define NP-hard and NP-complete problems. Explain the key differences between these two classes of problems. 2. What is Cook’s theorem? Provide a brief overview of its importance in computational complexity theory. 3. List the primary characteristics of NP-complete problems. How does a problem become NP- complete? 4. Describe the relationship between P, NP, and NP-complete classes. How do these relationships help in understanding the complexity of algorithms? 5. Explain the concept of polynomial-time reducibility. Why is it important for classifying problems as NP-complete? 1. Discuss why NP-hard problems are considered difficult to solve and how they differ from NP- complete problems. 2. Explain Cook’s theorem in detail, focusing on how it proves that the Boolean satisfiability problem (SAT) is NP-complete. 3. Analyze the implications of NP-complete problems in terms of real-world applications, such as optimization or cryptography. 4. Why is it significant to determine whether P = NP or P ≠NP? Discuss the potential consequences for computer science. 5. Illustrate how polynomial-time reductions are used to prove the NP-completeness of problems. Give an example of a reduction from one problem to another. 1. Apply the concept of Cook’s theorem to demonstrate why the SAT problem is NP-complete. Provide a detailed proof sketch for the reduction of another problem to SAT. 2. Using an example, explain how a problem in NP can be transformed into an NP-complete problem using polynomial-time reductions. 3. Demonstrate how solving an NP-complete problem in polynomial time would imply P = NP, and discuss its broader implications. 4. Solve a practical problem (e.g., scheduling, network design) and show how it can be framed as an NP-complete problem. 5. Using an NP-complete problem as an example, describe how you would use approximation algorithms to find a solution in cases where exact solutions are impractical. 1. Analyze the different techniques used to approach NP-complete problems, such as brute force, approximation algorithms, and heuristic methods. 2. Examine the role of Cook’s theorem in the evolution of complexity theory. How did it change the way researchers viewed computational problems? 3. Discuss the computational complexity of solving NP-hard problems versus NP-complete problems. Provide specific examples and compare their difficulty. 4. Critically analyze the impact of the P vs. NP question on fields such as cryptography, machine learning, and artificial intelligence. 5. Evaluate the role of reductions in proving NP-completeness. How do they contribute to our understanding of problem difficulty? 1. Evaluate the current state of research into NP-completeness and the potential for proving P = NP. What are the challenges that researchers face in attempting to resolve this question? 2. Assess the practical relevance of solving NP-complete problems in polynomial time. How would it impact industries like cybersecurity, logistics, and software development? 3. Debate whether heuristic methods and approximation algorithms are sufficient for dealing with NP-complete problems in real-world scenarios. 4. Critique the use of NP-completeness as a classification tool for problems. Are there cases where this classification does not fully capture the difficulty of solving a problem? 5. Debate the ethical implications of solving NP-complete problems efficiently. How might such advancements affect privacy, security, and fairness? 2. NP-Hard Graph Problems: Clique Decision Problem (CDP), Chromatic Number Decision Problem (CNDP), Travelling Salesperson Decision Problem (TSP) 1. Define the Clique Decision Problem (CDP), the Chromatic Number Decision Problem (CNDP), and the Travelling Salesperson Decision Problem (TSP). 2. What is the significance of decision problems in NP-hard graph problems? 3. List and describe the key components involved in the Clique Decision Problem (CDP). 4. State the basic criteria for the Chromatic Number Decision Problem (CNDP). How do you determine if a graph can be colored with a specific number of colors? 5. Explain the concept of the Travelling Salesperson Decision Problem (TSP) in simple terms, highlighting its NP-hard nature. 1. Discuss how the Clique Decision Problem (CDP) can be used to model real-world problems such as network connectivity or social groupings. 2. Explain the significance of the Chromatic Number Decision Problem (CNDP) in graph theory. How does this problem relate to scheduling and resource allocation? 3. Analyze the Travelling Salesperson Decision Problem (TSP) in the context of optimization problems. Why is TSP classified as NP-hard? 4. Explain the challenges involved in solving these NP-hard graph problems and why exact solutions are computationally expensive. 5. Discuss the relationship between NP-hard graph problems (like CDP, CNDP, and TSP) and real- world applications in areas like transportation, telecommunications, and social networks. 1. Solve a small graph coloring problem using the Chromatic Number Decision Problem and discuss the steps. 2. Apply the Clique Decision Problem (CDP) to a sample graph and show how it is used to identify cliques in social networks. 3. Provide a solution to a simple instance of the Travelling Salesperson Decision Problem and demonstrate its NP-hard nature. 4. Using an example, demonstrate how a problem like the CDP can be reduced to other NP- complete problems. 5. Apply the CNDP to a scheduling problem and discuss how it can help optimize the allocation of resources. 1. Analyze the computational difficulty of solving the Clique Decision Problem and discuss how it compares to other NP-hard graph problems. 2. Break down the steps involved in solving the Chromatic Number Decision Problem (CNDP) for large graphs and analyze its time complexity. 3. Discuss the impact of various heuristics (such as greedy algorithms) on solving the Travelling Salesperson Decision Problem. 4. Examine the importance of graph representation and adjacency matrices in solving NP-hard graph problems. 5. Critically analyze the implications of these NP-hard problems in real-world applications, such as network design or resource allocation. 1. Evaluate the effectiveness of approximation algorithms and heuristics in solving NP-hard graph problems like CDP, CNDP, and TSP. 2. Assess the challenges and limitations of using exact algorithms for solving the Clique Decision Problem in large networks. 3. Debate whether solving the Chromatic Number Decision Problem optimally in large graphs is feasible, given the NP-hard nature of the problem. 4. Critique the current methods for solving the Travelling Salesperson Decision Problem and suggest improvements or alternatives. 5. Evaluate the practical impact of NP-hard graph problems on industries like logistics, transportation, and communications. 3. NP-Hard Scheduling Problems: Scheduling Identical Processors, Job Shop Scheduling 1. Define the Scheduling Identical Processors problem and explain its relation to NP-hard problems. 2. What is the Job Shop Scheduling problem? Describe the main components and constraints involved. 3. List the types of problems classified as NP-hard in the context of scheduling. 4. State the importance of solving scheduling problems in real-world industries like manufacturing or cloud computing. 5. Explain the significance of resource constraints in scheduling problems. 1. Discuss the challenges of solving the Scheduling Identical Processors problem, and why it is classified as NP-hard. 2. Explain the basic concept behind Job Shop Scheduling and why it is computationally difficult to solve. 3. Discuss the difference between scheduling identical processors and scheduling with different processor types. 4. Explain how the constraints in scheduling problems (such as precedence and time) affect the search for optimal solutions. 5. Discuss the relationship between scheduling problems and other NP-hard problems like the 0/1 knapsack problem or the traveling salesperson problem. 1. Solve a simple example of the Scheduling Identical Processors problem and demonstrate the steps involved in finding an optimal solution. 2. Apply Job Shop Scheduling to a small manufacturing setup and explain the process of task assignment and resource allocation. 3. Create a small example that demonstrates the NP-hard nature of the Scheduling Identical Processors problem. 4. Using a scheduling problem with a given set of tasks, demonstrate the application of a heuristic approach to find a feasible solution. 5. Illustrate how Job Shop Scheduling can be used to optimize job allocation in a factory with multiple workstations. 1. Analyze the computational complexity of the Scheduling Identical Processors problem and compare it to other scheduling problems. 2. Break down the steps of solving a Job Shop Scheduling problem and analyze its time complexity. 3. Discuss the role of constraints in increasing the complexity of scheduling problems and how they affect the search for feasible solutions. 4. Examine the trade-offs between exact and approximate solutions for NP-hard scheduling problems. 5. Critically analyze how different heuristic and metaheuristic algorithms perform in solving large- scale Job Shop Scheduling problems. 1. Evaluate the feasibility of solving the Scheduling Identical Processors problem optimally in large systems. 2. Assess the impact of scheduling problems on real-world applications like manufacturing or cloud computing, and the consequences of NP-hardness. 3. Debate whether Job Shop Scheduling can be solved efficiently using current algorithms or if new approaches are needed. 4. Critique the limitations of current solutions to NP-hard scheduling problems and suggest improvements. 5. Evaluate the effectiveness of using approximation algorithms for solving Job Shop Scheduling problems in industries like automotive manufacturing.