AC-S24-OOP-Lect04-Part1-Testing-Javadoc.pdf
Document Details
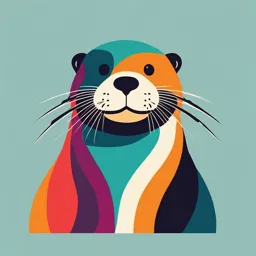
Uploaded by LuxuryAbundance
Algonquin College
2024
Tags
Full Transcript
Lect 04A CST8132 OOP Algonquin College Lect 04A Computer Engineering Technology CST8132 OOP Summer, 2024 Based on resources developed by prof....
Lect 04A CST8132 OOP Algonquin College Lect 04A Computer Engineering Technology CST8132 OOP Summer, 2024 Based on resources developed by prof. Howard Rosemblum, James Mwangi, Anu Thomas and Ramanjeet Singh. Prof. Paulo Sousa Algonquin College Lect 04A Computer Engineering Technology Testing / Javadoc CST8132 OOP Summer, 2024 Based on resources developed by prof. Howard Rosemblum, James Mwangi, Anu Thomas and Ramanjeet Singh. Prof. Paulo Sousa L1 L2 Week 4A: Testing / Javadoc A2W01 A2W02 A2W09 A2W10 A2W03 A2W11 A2W04 A2W12 A2W05 A2W13 Basic concepts A2W06 A2W14 Examples A2W07 A2W15 A2W08 4 OOP – Week 4 UML Basics 5 Topics UML Relationships Using Visuals Collections ArrayList Testing Junit 5 test cases Javadoc Documentation 6 Warm up – Brief Reviews Q1. 1 minute Explain the difference between Overloading and Overriding Q2. 1 minute Array of Primitives vs. Array of References Q3. 1 minute What does UML stand for? What is it, and what is its purpose? 3 7 Brief Answers Overriding – inherit a method and customize to meet local needs of subclass Overloading – methods in same scope sharing a common name but having different method signatures Arrays – Simple homogeneous collection: Array of primitives – holds actual values Array of references – holds references of addresses to objects UML – Unified Modeling Language. UML is a visual representation of class/object relationships. 3 8 UML Notation: Object-Oriented Analysis and Design Class Diagram Class Visibility Class Operations 9 UML Notation: Object-Oriented Analysis and Design Parameter Directionality Class Diagram Perspectives Conceptual: represents high-level concepts in the domain Specification: focus on interfaces of Abstract Data Type (ADTs) in the software Implementation: describes how classes will implement their interfaces 10 UML Notation: Object-Oriented Analysis and Design Super vs subclass Interface and implementing class Person (hasRead() vs book Part-of type of association Is-a type of association 11 Class Diagram Example: Order System Cardinality One-to-one One-to-many Many-to-many 12 OOP – Week 4 ArrayList 13 ArrayList Definitions Is an example of Java Collection. There are other collections (to be covered in higher levels of your course QUESTION: How is arrayList different from an array? 3 14 Note 15 ArrayList collection OOP – Week 4 Testing 17 Testing Definitions The way to build robust software is through testing. We will creating JUnit Test Cases Unit Testing: the process of examining a small ‘unit’ of application such as a class to verify its works as per requirements specification and meets expectations. 3 18 Testing 19 JUnit Junit is a facility for automated unit testing It supports test classes that run your methods and “look for the unexpected” Your tests will use the static methods of the Assert class to assert the condition you are testing for See sections 1 – 4 of http://www.vogella.com/tutorials/JUnit/article.html 20 Writing Tests (Using JUnit 5) Steps Open project on eclipse Select the class to test Right click class => New => JUnit test cases => edit the test methods as required. 21 Writing Tests (Using JUnit 5) 22 Writing Tests (Using JUnit 5) 23 Test Plans A test plan is a comprehensive strategy for ensuring that the system does not contain errors: Unit testing: Testing the individual units of our programs (classes, methods) Integration testing: Putting the units together, and testing their use of each other’s interfaces etc Functional testing: Tests whether the overall system matches the behaviour defined in the system requirements Acceptance testing Evaluate the system’s compliance with the business requirements and assess whether it is acceptable for delivery. 24 V model (Waterfall) 25 Iterative Development Model (Agile) 21 26 12 Boundary Conditions In developing good tests for your code, you will need to identify all the boundary conditions You want to test with data that probes the boundary conditions What are the boundaries in an array? int [] num = new int; Array indices: -1, 0 , 1 Array indices: 99, 100 Boundaries on int values? Integer.MAX_VALUE, Integer.MIN_VALUE, int values change sign: -1, 0, 1 27 Summary Testing – How are you going to test if the program is working properly Unit – lowest level testing (Class and methods) Integration - test the integration between a set of components Functional/System – test if the system works as a whole JUnit – Automated tool to unit test your code 28 OOP – Week 4 Javadoc 29 Javadoc Definition Generates a web-based documentation that we can use to inspect the source-level structures of programs and libraries, including API comments embedded in the source. Comes with Java (not only IDE). 30 Javadoc Package jdk.javadoc.doclet The Doclet API provides an environment which, in conjunction with the Language Model API and Compiler Tree API, allows clients to inspect the source-level structures of programs and libraries, including API comments embedded in the source. 31 Javadoc Documentation generator created for the Java language Intended for other developers and represents the API of your Java class. Produces formatted HTML documentation easily for your code. Can produce automatic documentation from your code, however you can supplement this using special comments throughout your code. 32 How To Generate Javadoc Steps 1. Open project in Eclipse and select it on package explorer 2. Project => Generate Javadoc 3. Select destination 4. Select visibility – package, private, protected, public 33 Javadoc Sample generated Javadoc 34 Javadoc Javadoc comments must be enclosed by Javadoc comments MUST appear on the line(s) immediately preceding the code they describe. Wherever a description is required, a proper descriptive sentence or sentence fragment is expected. Javadoc is REQUIRED on all assignments going forward. Javadoc is not a replacement for comments. 35 Javadoc Consist of descriptive text, and tagged descriptors. Tags begin with an @ symbol, and always use lower-case names. Some tags are required, and some are optional. Some may be conditionally repeated. Conditionally required whether you are documenting classes, properties or methods. Class documentation does not replace the required header as described in requirements. 36 Javadoc Java Class Documentation Every class requires the following Javadoc to appear immediately above the class declaration (below any package or import statements). 37 Javadoc Java Property Documentation Every non-private property (instance or class variable) requires a Javadoc description to appear immediately above the variable declaration. protected doublebalance; protected long accountNum; public Client client; 38 Javadoc Java Property Documentation Declare every property on a new line. Private properties do not require Javadoc. Do not simply repeat the variable name – this is not sufficient. Javadoc gets the name and data type automatically. Your job is to write qualitative information about the property. The valid range of values is a good thing to include in Javadoc documentation. For example: protected int hour; 39 Javadoc Java Method Documentation Every non-private method requires a Javadoc description to appear immediately above the method declaration. Constructors and the main method require Javadoc!!! Methods with parameters require the Javadoc to contain one @param tag for each parameter. Methods with a return type require the Javadoc to contain one and only one @return tag for the return value. In the begin of exception handling, methods which throw exceptions should have an @throws tag for each exception. 40 Javadoc Javadoc Requirements [Description] @author [author name] If more than one author, each author has their own @author tag. Order from mostrecent author at the top of the list to the oldest at the end of the list. @version [software version] For your assignments, use a sensible version such as 1.0. @since [jdk version] We are using version 1.8. Could provide full version. @param [name] [description] The name of the parameter must match the Javadoc name. @return [description] 41 Javadoc Javadoc Details Javadoc descriptions may contain HTML mark-up for additional formatting opportunities. Javadoc does NOT replace the need for comments in your code. Code blocks such as control structures still require comments. Javadoc should not appear inside of any methods – only comments belong inside of methods. To enable Javadoc validation in Eclipse: Window > Preferences > Java > Compiler > Javadoc 42 Generation Javadoc Javadoc will only be generated based on comments within frame. To generate Javadoc in Eclipse: Select the File → Export → Java→ Javadoc menu option. Click the Configure… button next to the Javadoc Command text box, and browse to your installed javadoc.exe file. For example: C:\Program Files\Java\jdk1.8.0_40\bin\javadoc.exe. Ensure that the project and ALL java files within are selected. From Create Javadoc for members with visibility, select the Package option. Leave the Destination with the default value. Click Finish. 43 Generation Javadoc 44 Generation Javadoc API Documentation - https://docs.oracle.com/en/java/javase/11/docs/api/index.html# String - https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/la ng/String.html 45 Summary 1. Inheritance Super-class, sub-class Super keyword – super(), super.method() 2. Polymorphism Taking multiple forms Static polymorphism – compile time (ex. Method overloading) Dynamic polymorphism – runtime (ex. Method overriding) 3. Abstract class Providing abstract of methods…. Will be overridden in child classes Can have non-abstract methods – they can have their definitions in the abstract class 4. Interface Only abstract methods and public static final variables Abstract keyword not required – by default, methods are abstract 46 Open questions… Any doubts / questions? How we are until now? 47 See you… Enjoy our course and season… 48 OOP – Week 2 Thank you for your attention! Contact: [email protected] 49