Queue Data Structure Explained
Document Details
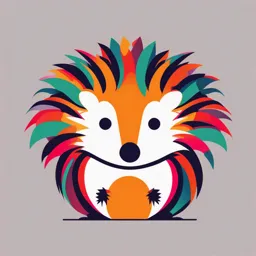
Uploaded by EnterprisingOrchid199
Tags
Related
- Data Structures Midterms Reviewer PDF
- CPP253 - Data Structures PDF
- Data Structures and Algorithm Analysis in C++ 4th Edition PDF
- MAULANA ABUL KALAM AZAD UNIVERSITY OF TECHNOLOGY, WEST BENGAL BCA 3rd Semester Data Structures and Algorithms Exam 2023-2024 PDF
- Data Structures & Algorithms PDF
- Data Structure Lecture Notes PDF
Summary
This document provides an explanation of the queue data structure, covering its concepts, types (simple, priority, circular, double-ended), and applications in computer science and software.
Full Transcript
concept in computer science used for storing and managing data in a specific order. It follows the principle of “First in, First out” (FIFO), where the first element added to the queue is the first one to be removed. - Queues are commonly used in various algorithms and applications for their simplic...
concept in computer science used for storing and managing data in a specific order. It follows the principle of “First in, First out” (FIFO), where the first element added to the queue is the first one to be removed. - Queues are commonly used in various algorithms and applications for their simplicity and efficiency in managing data flow. Applications of Queue -Task scheduling in operating systems -Data transfer in network communication -Simulation of real-world systems (e.g., waiting lines) -Priority queues for event processing queues for event processing Types of Queue Data Structure: Queue data structure can be classified into 4 types: There are different types of queues: 1-Simple Queue: Simple Queue simply follows FIFO Structure. We can only insert the element at the back and remove the element from the front of the queue. 2-Double Queue (Dequeue): In a double-ended queue the insertion and deletion operations, both can be performed from both ends. They are of two types: -Input Restricted Queue: This is a simple queue. In this type of queue, the input can be taken from only one end but deletion can be done from any of the ends. -Output Restricted Queue: This is also a simple queue. In this type of queue, the input can be taken from both ends but deletion can be done from only one end. 3-Circular Queue: In Circular Queue, all the nodes are represented as circular. It is similar to the linear Queue except that the last element of the queue is connected to the first element. It is also known as Ring Buffer, as all the ends are connected to another end. The representation of circular queue is shown in the below image – 4-Priority Queue: It is a special type of queue in which the elements are arranged based on the priority. It is a special type of queue data structure in which every element has a priority associated with it. Suppose some elements occur with the same priority, they will be arranged according to the FIFO principle. The representation of priority queue is shown in the below image - There are two types of priority queue that are discussed as follows Ascending priority queue - In ascending priority queue, elements can be inserted in arbitrary order, but only smallest can be deleted first. Suppose an array with elements 7, 5, and 3 in the same order, so, insertion can be done with the same sequence, but the order of deleting the elements is 3, 5, 7. Descending priority queue - In descending priority queue, elements can be inserted in arbitrary order, but only the largest element can be deleted first. Suppose an array with elements 7, 3, and 5 in the same order, so, insertion can be done with the same sequence, but the order of deleting the elements is 7, 5, 3. Basic Operations in Queue Data Structure: Some of the basic operations for Queue in Data Structure are: *Enqueue: Adds (or stores) an element to the end of the queue.. *Dequeue: Removal of elements from the queue. *front: Acquires the data element available at the front node of the queue without deleting it. *rear: This operation returns the element at the rear end without removing it. *isFull: Validates if the queue is full. *isEmpty: Checks if the queue is empty. // C++ program to illustrate how to enqueue an element into // a queue #include #include using namespace std; int main() { // Creating a queue of integers queue myQueue; myQueue.push(10); myQueue.push(20); myQueue.push(30); // enqueue an element in a queue int element = 40; myQueue.push(element); // Displaying the queue elements cout