NumPy and Pandas Practice Questions - PDF
Document Details
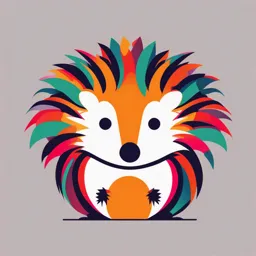
Uploaded by KnowledgeableAntigorite7564
Tags
Summary
This document contains a series of practice questions covering NumPy and Pandas, including questions about array manipulation, random number generation, DataFrame creation and operations. The questions have a focus on basic usage and comprehension of NumPy and Pandas.
Full Transcript
1. What does the np.arange(5) function return? A. [0, 1, 2, 3, 4] B. [1, 2, 3, 4, 5] C. [0, 1, 2, 3, 4, 5] D. [1, 2, 3] Answer: A. [0, 1, 2, 3, 4] 2. What will be the output of the following code? python CopyEdit import numpy as np arr = np.array([[1, 2, 3], [4, 5, 6]]) print(arr.sum(axis=1)...
1. What does the np.arange(5) function return? A. [0, 1, 2, 3, 4] B. [1, 2, 3, 4, 5] C. [0, 1, 2, 3, 4, 5] D. [1, 2, 3] Answer: A. [0, 1, 2, 3, 4] 2. What will be the output of the following code? python CopyEdit import numpy as np arr = np.array([[1, 2, 3], [4, 5, 6]]) print(arr.sum(axis=1)) A. [6, 15] B. [3, 6] C. [1, 2] D. [5, 7] Answer: A. [6, 15] 3. Which of the following will create a 3x3 identity matrix in NumPy? A. np.zeros((3, 3)) B. np.ones((3, 3)) C. np.identity(3) D. np.diag([1, 1, 1]) Answer: C. np.identity(3) 4. Which of the following NumPy functions is used to generate random numbers between 0 and 1? A. np.random.random() B. np.random.randint() C. np.random.uniform() D. np.random.randn() Answer: A. np.random.random() 5. What is the output of the following code? python CopyEdit import numpy as np a = np.array([1, 2, 3]) b = np.array([4, 5, 6]) print(np.dot(a, b)) A. 32 B. 8 C. 14 D. 3 Answer: C. 14 6. Which method is used to add a new row at the end of a NumPy array? A. np.append() B. np.insert() C. np.concatenate() D. np.add_row() Answer: A. np.append() Pandas (7-12) 7. What is the output of the following code? python CopyEdit import pandas as pd data = {'A': [1, 2], 'B': [3, 4]} df = pd.DataFrame(data) print(df['A'].sum()) A. 5 B. 1 C. 6 D. 3 Answer: A. 5 8. How can you check if there are any missing values in a Pandas DataFrame? A. df.isnull() B. df.null() C. df.check() D. df.isna() Answer: A. df.isnull() 9. What will be the output of the following code? python CopyEdit import pandas as pd data = {'A': [1, 2], 'B': [3, 4]} df = pd.DataFrame(data) df['C'] = df['A'] * df['B'] print(df) A. A DataFrame with three columns and new values in column C. B. Only columns 'A' and 'B' are printed. C. The code will throw an error. D. The code will print column 'C' only. Answer: A. A DataFrame with three columns and new values in column C. 10. Which method is used to drop rows with missing values from a Pandas DataFrame? A. df.dropna() B. df.remove_missing() C. df.clean() D. df.fillna() Answer: A. df.dropna() 11. What does the df.groupby() method do in Pandas? A. Groups data based on column values for aggregation or transformation. B. Sorts the DataFrame by a given column. C. Removes duplicate entries from a DataFrame. D. Splits the DataFrame into smaller DataFrames. Answer: A. Groups data based on column values for aggregation or transformation. 12. How do you select rows where the values of column 'A' are greater than 2? python CopyEdit df = pd.DataFrame({'A': [1, 2, 3, 4]}) A. df.loc[df['A'] > 2] B. df.query('A > 2') C. df[df['A'] > 2] D. All of the above Answer: D. All of the above