UNIT 2.pdf
Document Details
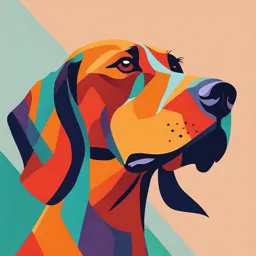
Uploaded by BlitheIron
Tags
Full Transcript
UNIT-2 Introduction to AngularJS and MVC Architecture Introduction AngularJS is a very powerful JavaScript Framework. It is used in Single Page Application (SPA) projects. It extends HTML DOM with additional attributes and makes it more responsive to user actions...
UNIT-2 Introduction to AngularJS and MVC Architecture Introduction AngularJS is a very powerful JavaScript Framework. It is used in Single Page Application (SPA) projects. It extends HTML DOM with additional attributes and makes it more responsive to user actions. AngularJS is open source, completely free, and used by thousands of developers around the world. It is licensed under the Apache license version 2.0. Single page application A Single Page Application (SPA) is a web application or website that interacts with the user by dynamically rewriting the current page rather than loading entire new pages from the server. This approach allows for a more fluid and responsive user experience, similar to a desktop application. SPAs aim to provide a seamless user experience by reducing the amount of data that needs to be sent back and forth between the client and server, often resulting in faster interactions. Key Characteristics of SPAs: 1.Dynamic Content Loading: Content is dynamically loaded and updated as needed without refreshing the entire page. 2.Rich User Interaction: SPAs allow for more interactive features, like modals, tooltips, and animations, without the need for multiple page reloads. 3.Routing on the Client Side: SPAs use client-side routing to manage different views and states within the application without triggering full page reloads. 4.API-Centric: SPAs often interact with backend services via APIs (e.g., REST or GraphQL) to fetch or manipulate data. Example of a Single Page Application: Gmail is a classic example of a SPA. When you use Gmail, the entire application runs within a single page, and as you navigate through your inbox, open emails, compose messages, or switch between folders, the application updates content dynamically. In Gmail: When you open an email: The content of the email loads dynamically in the main view without reloading the entire page. When you navigate to different sections (e.g., Inbox, Sent, Drafts): The navigation happens instantly without a full page refresh. When composing a new email: The email composer opens within the same page, providing a smooth experience without disrupting the overall flow. Benefits of SPAs: Faster Loading Times: Once the application is loaded, only specific data is retrieved from the server, making interactions faster. Better User Experience: The application feels more responsive and similar to native apps. Easier to Develop for Multiple Platforms: SPAs can easily be adapted for mobile, desktop, and web platforms using a common codebase. Drawbacks of SPAs: Initial Load Time: The initial loading of the application might take longer since all the necessary resources (HTML, CSS, JavaScript) are loaded at once. SEO Challenges: Since SPAs rely heavily on JavaScript, search engine optimization can be more challenging, though this is improving with techniques like server-side rendering (SSR). Browser Compatibility Issues: Some older browsers may not fully support SPAs, though this is less of an issue with modern web development. Why to Learn AngularJS? AngularJS was originally developed in 2009 by Misko Hevery and Adam Abrons. It is now maintained by Google. AngularJS is a efficient framework that can create Rich Internet Applications (RIA). AngularJS provides developers an options to write client side applications using JavaScript in a clean Model View Controller (MVC) way. Applications written in AngularJS are cross-browser compliant. AngularJS automatically handles JavaScript code suitable for each browser. Overall, AngularJS is a framework to build large scale, high- performance, and easy-to-maintain web applications. Core Features 1. Data-binding: It is the automatic synchronization of data between model and view components. 2. Scope: These are objects that refer to the model. They act as a glue between controller and view. 3. Controller: These are JavaScript functions bound to a particular scope. 4. Services: AngularJS comes with several built-in services such as $http to make a XML Http Requests. These are singleton objects which are instantiated only once in app. 5. Filters: These select a subset of items from an array and returns a new array. 5. Directives: Directives are markers on DOM elements such as elements, attributes, css, and more. These can be used to create custom HTML tags that serve as new, custom widgets. AngularJS has built-in directives such as ngBind, ngModel, etc. 6. Templates: These are the rendered view with information from the controller and model. These can be a single file (such as index.html) or multiple views in one page using partials. 7. Routing: It is concept of switching views. 8. Model View Whatever: MVW is a design pattern for dividing an application into different parts called Model, View, and Controller, each with distinct responsibilities. AngularJS does not implement MVC in the traditional sense, but rather something closer to MVVM (Model-View-ViewModel). 9. Deep Linking: Deep linking allows to encode the state of application in the URL so that it can be bookmarked. The application can then be restored from the URL to the same state. 10. Dependency Injection: AngularJS has a built-in dependency injection subsystem that helps the developer to create, understand, and test the applications easily. DATA BINDING Data binding is the synchronization of data between business logic and view of the application. It serves as a bridge between two components of angular that is model part and view part. Data Binding is automatic and provides a way to wire the two important part of an application that is the UI and application data. Whenever some changes are done at the model side it is reflected at view side too and vice versa is also possible. This happens so rapidly to make sure that view and the model part will get update all the time. DATA BINDING The directives in AngularJs used to bind the value of the input field (such as text field, text area) to the HTML element is ng-model. An ng-model directive is used to perform data binding in angular. We don’t have to write extra code to link the model part with view part by adding few snippets of code we can bind the data with the HTML control. Input something in the input box: Name: You are learning: {{ language }} Types of Data Binding in AngularJS 1) One Way Data Binding The flow of data restricts to one side only and that is from model to view. It follows a unidirectional approach. In the case of updating in the model part, same will sync in the view part also but the vice versa is not possible as in one way binding data can’t flow from view to model. Interpolation binding (an expression is taken as an input and it is changed as a text using HTML elements), property binding (Value of a property is set on an HTML element) comes in the category of one-way data binding in AngularJS. 2) Two Way Data Binding in AngularJS The flow of data does not restricts to one side only. It is from model to view as well as the view to model is also possible. Two way data binding follows a bidirectional approach as a flow of data is possible in both the side. Any changes made in the model part will sync in view part as well as any changes made in view part is synced in model part also. Event binding (an event triggers at view side by the user event binding allows components to listen to that event) comes in the category of two-way data binding in AngularJS. The syntax for event binding: Update (click) is the event here. An event is always written in parenthesis. ‘updateproduct()’ is the method here. A method is written just after the event Whenever the event triggers by the user, the method is called. SCOPE Concepts Advantages of AngularJS It provides the capability to create Single Page Application in a very clean and maintainable way. It provides data binding capability to HTML. Thus, it gives user a rich and responsive experience. AngularJS code is unit testable. AngularJS uses dependency injection and make use of separation of concerns. AngularJS provides reusable components. With AngularJS, the developers can achieve more functionality with short code. In AngularJS, viewsare pure html pages, and controllers written in JavaScript do the business processing. Disadvantages of AngularJS Not Secure: Being JavaScript only framework, application written in AngularJS are not safe. Server side authentication and authorization is must to keep an application secure. Not degradable: If the user of your application disables JavaScript, then nothing would be visible, except the basic page. AngularJS - MVC Architecture Model View Controller or MVC as it is popularly called, is a software design pattern for developing web applications. A Model View Controller pattern is made up of the following three parts: Model: It is the lowest level of the pattern responsible for maintaining data. View: It is responsible for displaying all or a portion of the data to the user. Controller: It is a software Code that controls the interactions between the Model and View. MVC is popular because it isolates the application logic from the user interface layer and supports separation of the concerns. The controller receives all requests for application and then works with the model to prepare any data needed by the view. The view then uses the data prepared by the controller to generate a final presentable response. The MVC abstraction can be graphically represented as follows. The Model: The model is responsible for managing application data. It responds to the request from view and to the instructions from controller to update itself. The View: A presentation of data in a particular format, triggered by the controller's decision to present the data. They are script-based template systems such as JSP, ASP, PHP and very easy to integrate with AJAX technology. The Controller: The controller responds to user input and performs interactions on the data model objects. The controller receives input, validates it, and then performs business operations that modify the state of the data model. AngularJS Directives AngularJS directives are used to extend HTML. They are special attributes starting with ng-prefix. Let us discuss the following directives: The AngularJS framework can be divided into three major parts: ng-app: This directive defines and links an AngularJS application to HTML. ng-model: This directive binds the values of AngularJS application data to HTML input controls. ng-bind: This directive binds the AngularJS application data to HTML tags. Creating AngularJS Application Step 1: Load framework Being a pure JavaScript framework, it can be added using tag. Step 2: Define AngularJS application using ng- app directive... Step 3: Define a model name using ng-model directive Enter your Name: Step 4: Bind the value of above model defined using ng-bind directive Hello ! Code Hello AngularJS First Application Sample Application Enter your Name: Hello ! ng-app directive The ng-app directive starts an AngularJS Application. It defines the root element. It automatically initializes or bootstraps the application when the web page containing AngularJS Application is loaded. In the following example, we define a default AngularJS application using ng-app attribute of a element.... ng-init directive The ng-init directive initializes an AngularJS Application data. It is used to assign values to the variables. In the following example, we initialize an array of countries. We use JSON syntax to define the array of countries.... ng-model directive The ng-model directive defines the model/variable to be used in AngularJS Application. In the following example, we define a model named name.... Enter your Name: ng-repeat directive The ng-repeat directive repeats HTML elements for each item in a collection. In the following example, we iterate over the array of countries.... List of Countries with locale: {{ 'Country: ' + country.name + ', Locale: ' + country.locale }} ng-controller Attaches a controller to the view ng-bind Binds the value with an HTML element Repeats HTML template once per each item in the specified ng-repeat collection. ng-show Shows or hides the associated HTML element Conditionally hides an HTML element based on the truthiness of an ng-hide expression. ng-readonly Makes HTML element read-only ng-disabled Use to disable or enable a button dynamically ng-if Removes or recreates HTML element ng-click Custom step on click Conditionally applies CSS classes to an element based on the ng-class evaluation of expressions. Binds a function to the submit event of an HTML form, allowing ng-submit execution of custom behavior on form submission. AngularJS Expressions AngularJS expressions can be written inside double braces: {{ expression }}. AngularJS expressions can also be written inside a directive: ng-bind="expression". AngularJS will resolve the expression, and return the result exactly where the expression is written. AngularJS expressions are much like JavaScript expressions: They can contain literals, operators, and variables. Example {{ 5 + 5 }} or {{ firstName + " " + lastName }} Example My first expression: {{ 5 + 5 }} AngularJS Controllers AngularJS applications are controlled by controllers. The ng-controller directive defines the application controller. A controller is a JavaScript Object, created by a standard JavaScript object constructor. Example First Name: Last Name: Full Name: {{firstName + " " + lastName}} var app = angular.module('myApp', []); app.controller('myCtrl', function($scope) { $scope.firstName = "John"; $scope.lastName = "Doe"; }); AngularJS Filters AngularJS provides filters to transform data: currency - Format a number to a currency format. date - Format a date to a specified format. filter - Select a subset of items from an array. json - Format an object to a JSON string. limitTo - Limits -an array/string, into a specified number of elements/characters. lowercase - Format a string to lower case. number - Format a number to a string. orderBy - Orders an array by an expression. uppercase - Format a string to upper case. Example The name is {{firstName + " " + lastName | uppercase }} angular.module('myApp', []).controller('personCtrl', function($scope) { $scope.firstName = "John", $scope.lastName = "Doe" }); AngularJS Tables The ng-repeat directive is perfect for displaying tables. Example {{ x.Name }} {{ x.Country }} AngularJS HTML DOM AngularJS has directives for binding application data to the attributes of HTML DOM elements. Click Me! Button {{ mySwitch }} The ng-disabled directive binds the application data mySwitch to the HTML button's disabled attribute. The ng-model directive binds the value of the HTML checkbox element to the value of mySwitch. If the value of mySwitch evaluates to true, the button will be disabled. AngularJS Forms Forms in AngularJS provides data-binding and validation of input controls. Input controls are the HTML input elements: 1. input elements 2. select elements 3. button elements 4. textarea elements Data-Binding Input controls provides data-binding by using the ng-model directive. The application does now have a property named firstname. The ng-model directive binds the input controller to the rest of your application. The property firstname, can be referred to in a controller. Checkbox A checkbox has the value true or false. Apply the ng-model directive to a checkbox, and use its value in your application. Check to show a header: My Header Radiobuttons Bind radio buttons to your application with the ng- model directive. Radio buttons with the same ng-model can have different values, but only the selected one will be used. Pick a topic: Dogs Tutorials Cars Select box Bind select boxes to your application with the ng- model directive. The property defined in the ng-model attribute will have the value of the selected option in the select box. Select a topic: