7-Repetition.pptx
Document Details
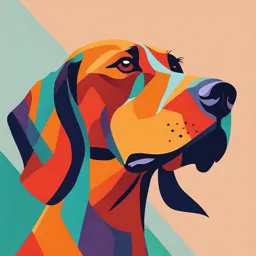
Uploaded by IndulgentPelican
University of Doha for Science and Technology
Full Transcript
Repetition COM136 – Software Development 1 1 Learning Outcomes • At the end of this lecture you should • Learn why repetition constructs are a necessary part of a programming language. • Recognise when repetition constructs should be used to solve programming problems. • Learn how to write progra...
Repetition COM136 – Software Development 1 1 Learning Outcomes • At the end of this lecture you should • Learn why repetition constructs are a necessary part of a programming language. • Recognise when repetition constructs should be used to solve programming problems. • Learn how to write programs that use the Java while loop repetition construct. • Learn about the difference between counter controlled and sentinel controlled while loops. • Be able to perform a dry run in a program containing while loops to analyse what it does. 2 Exercise • You have been told to write out “I must practice my programming” 5 times as a punishment. • Algorithm PRINT “I must practice my programming” PRINT PRINT PRINT PRINT “I “I “I “I must must must must practice practice practice practice my my my my programming” programming” programming” programming” • But what if you were asked to write it out 100 times!! 3 While-loop - Repetition Concept • We can use a while loop which repeats a statement (or compound statement) while some condition is true. Pseudo code counter = 1 WHILE counter <=500 PRINT “I must practice my programming!!” counter = counter + 1 END WHILE 4 While loop Java Syntax • The syntax of the while loop statement is: while ( <boolean expression> ) <statement> • In Java, while is a reserved word which tells the compiler this is a while loop statement. • The <statement> can either be a simple or compound statement { }. • The <boolean expression> must be enclosed in ( ) and is called the loop condition. 5 While Loop Semantics • expression is evaluated and if true body of loop is executed, and expression is re-evaluated. • When expression evaluates to false the loop terminates expression true statement false 6 Java Code // Print a message a fixed number of times public class Counter1 { public static void main(String[] args) { int counter = 1; while ( counter <= 5 ) { Compound System.out.println("I must practice my programming"); statement counter = counter + 1; } } } 7 Initialisation • Loops often use some form of counter variable to determine how many times the loop should be executed • This counter must be initialised (given a starting value) before the loop is entered • A declaration and initialisation assignment can be combined as follows: int counter = 1; 8 ** Counter Controlled Loops ** • Where the loop is executed a fixed number of times controlled by a counter • This is a counter controlled while loop. • Counter-Control Loop Pattern int counter = <start value>; while ( counter <= <end value> ) { <instructions to be repeated> counter = counter + <increment>; } 9 Counter Controlled Loops • Here <start value>, <end value> and <increment> are numbers chosen to suit the particular algorithm being developed. The loop is then executed a fixed number of times, controlled by the counter variable. • Often the loops you will write will simply execute a fixed number of times so typically • <start value> will be 1 • <end value> will be the number of times you want the loop to execute • <increment> will be 1 10 Exercise • Write a program to print the average of five numbers entered by the user. total = 0 counter = 1 WHILE counter <= 5 PRINT “Enter number ” INPUT number total = total + number counter = counter + 1 ENDWHILE average = total / 5 PRINT average Pass# Variable Values 1 number = 2 total = 2 counter =2 2 number = 5 total = 7 counter =3 3 number = 10 total = 17 counter = 4 4 number = 3 total = 20 counter =5 5 number = 30 total = 50 11 counter = 6 Java Solution import uulib.Console; // Calculate average of 5 numbers input by user public class Counter2 { public static void main(String[] args) { final int QTY = 5; // constant qty of numbers read int total = 0, counter = 1; while ( counter <= QTY) { int num = Console.getInt("Enter number " + counter); total = total + num; counter = counter + 1; } int average = total / QTY; System.out.println("The average is " + average); } } 12 Improved Version • An improved algorithm – user specifies how many numbers will be entered at beginning of algorithm. total = 0, counter = 1 PRINT “Enter qty” INPUT qty WHILE counter <= qty PRINT “Enter number “ + counter INPUT number total = total + number counter = counter + 1 ENDWHILE average = total / qty PRINT “Average of “ + qty + “ numbers is “ + average 13 Java Solution import uulib.Console; // Calculate average of fixed number of numbers input from user public class Counter3 { public static void main(String[] args) { int total = 0, counter = 1; int qty = Console.getInt(“Enter qty to average: ”); while ( counter <= qty ) { int num = Cponsole.getInt("Enter number " + counter + “: “); total = total + num; counter = counter + 1; } int average = total / qty; System.out.println("The average is " + average); } } 14 Problem with Previous Solution • If a user enters 0 for qty then the statement average = total/qty; will result in a “divide by zero” error. • Two solutions • • Use if statement to test if qty is zero before calculating the average. Do not allow the user to enter 0 for qty. • Solution 1 allows user to run the program and then decide not to average any numbers, while solution 2 forces the user to average some numbers even if they change their mind. 15 Solution 1- Improved Counter int total = 0, counter = 1; int qty = Console.getInt(“Average how many numbers? ”); while (counter <= qty) { int num = Console.getInt("Enter number "+ counter + “: “); total = total + num; counter = counter + 1; } // ensure qty is greater than zero if (qty > 0) { int average = total / qty; System.out.println("The average is " + average); } 16 Solution 2 – Improved Counter We could use a loop to force the user to enter a positive number as follows int qty = Console.getInt(“Enter qty to average: ”); // ensure positive qty is input while (qty <= 0) { qty = Console.getInt(“Enter positive qty to average: ”); } Loop will only be executed when qty is invalid This type of loop is called a sentinel loop Solution 2 – Improved counter int total = 0, counter = 1; int qty = Console.getInt(“Enter qty to average: ”); while (qty <= 0) { // force user to enter positive value qty = Console.getInt(“Enter positive qty to average: ”); } while ( counter <= qty ) { int num = Console.getInt("Enter number " + counter + “: “); total = total + num; counter = counter + 1; } int average = total / qty; // qty can never be 0 System.out.println("The average is " + average); 18 ** Sentinel-controlled Loops ** • When you don’t know how times the loop will execute you need to use what is known as a sentinel-controlled loop. • Sentinel-Control Loop Pattern int value = <get initial input value>; while ( value != sentinelValue ) { <manipulate value> value = <get next input value>; } 19 Sentinels • Loop will repeat an unknown number of times. • On each repetition a value is input. • The repetition stops when a particular value is input. • This is called the sentinel value. • The program should tell the user what to enter to indicate end of input. • The sentinel value may be declared as a constant using keyword final. 20 Example Sentinel Loop • Write a program which adds a series of numbers input by the user. It is not known in advance how many values will be input, but zero will not be one of the numbers. • We can therefore use zero as the sentinel value. • So keep adding numbers until the last number read is zero, then terminate the loop and print the total value of numbers read to date. 21 Algorithm total = 0 PRINT “enter number 0 to quit” INPUT number WHILE ( number != 0 ) total = total + number PRINT “enter next number” INPUT number END WHILE PRINT “The total is “ + total 22 Partial Java Solution final int SENTINEL = 0; int total = 0; // sentinel // store total int num = Console.getInt("Enter number (0 to finish):"); while ( num != SENTINEL ) { total = total + num; // add num to total // input next number (when 0 allows exit from loop) num = Console.getInt("Enter number (0 to finish): "); } System.out.println("The total is " + total); 23 Dry Running Program with Loop total (num!=SENT 4 (loop1) 4 5 (loop1) 4 6 (loop1) 6 0 4 4 true true 6 (loop2) 2 4 10 10 4 (loop3) 2 7. System.out.println("The total is " + total); 5 (loop3) 2 6 (loop3) 0 10 12 12 true 4 (loop4) 0 12 false final int SENTINEL = 0; 2. int total = 0; 3. int num 4. while ( 5. total 6. num = } Line 2 3 num 0 4 0 = Console.getInt("Enter …"); num != SENTINEL ) { = total + num; // add num to total 4 (loop2) 6 Console.getInt("Enter …"); 5 (loop2) 6 7 0 12 24 Loops with Complex Conditions • A loop condition can be any form of boolean expression. • Consider the algorithm to calculate the Syracuse sequence. Rules are as follows: 1. 2. 3. 4. Think of a positive whole number If number is even half it else triple it and add 1. Print the number Repeat step 2 until the number is 1. • Implement this algorithm, but we only want to determine at most the first 20 numbers in sequence. 25 Syracuse Algorithm v1 PRINT “Enter number” INPUT num WHILE (num != 1) IF (num is even) num = num / 2 What type of loop is this? Counter Controlled? Sentinel Controlled? ELSE num = num * 3 + 1 ENDIF Answer: Sentinel Controlled PRINT “Next number is “ + num ENDWHILE 26 Syracuse Algorithm v2 PRINT “Enter number” INPUT num length = 1 WHILE (num != 1 AND length <= 20) IF (num is even) num = num / 2 ELSE num = num * 3 + 1 ENDIF PRINT num What type of loop is this? Counter Controlled? Sentinel Controlled? Answer: Could be considered both length = length + 1 ENDWHILE 27 Partial Java Solution final int MAX = 20; // constant max sequence int length = 1; // length of sequence int num = Console.getInt("Enter starting number "); while ( num != 1 && length <= MAX ) { // determine if number is odd or even if ( (num % 2) == 0 ) { num = num / 2; // even number so halve } else { num = num * 3 + 1; // odd number so treble plus 1 } System.out.println(“Next number in sequence is “ + num); length = length + 1; } // another number generated 28 Scanner Input Issues Revisited Previously we looked at Scanner to read user input however it did not handle error conditions public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print(prompt); int num sc.nextInt(); Create a scanner using input console Return next input value as an integer or cause a runtime error if input is not an System.out.println(“Number was “ +integer num); sc.close(); } } Close the scanner Revisit Console Class Implementation Scanner has some useful methods. For example we can check if input contains a valid value e.g. hasNextInt(), hasNextDouble(), hasNextBoolean() etc. Also we can just ignore current erroneous input value and move to next input value using next() Using a loop and these methods we can implement the methods available in the Console class Console Class getInt(..) method public class Console { static Scanner sc = new Scanner(System.in); public static int getInt(String prompt) { System.out.print(prompt); while (!sc.hasNextInt()) { Create global scanner using input console repeat while integer is not available System.out.println("Invalid integer. Please re-enter"); sc.next(); } return sc.nextInt(); } // other methods } move scanner to next input value Return the integer which is available and no error will be thrown Source code management – (Version control) Source code management (SCM) is used to track modifications to a source code repository (a software application) over time. Modifications contribute to a release (new version of software) SCM allows us to review/undo changes to a code base (revision history) Generally helps manage a development teams contributions to a software product Source Code Management (SCM) Git is the most popular source code management system in use today (created by Linus Torvalds – creator of the Linux kernel) Github is a cloud based web application that allows developers to store their git repositories in the cloud and share repositories with other developers GitLab is an alternative to Github Both use the Git source code versioning Git & GitHub / GitLab make changes Source code directory push commit Staging (changes) Local Repositor y Interne t reset (commit) pull Remote Repositor y Summary • The while-loop is a common loop structure which allows us to repeat a sequence of instructions multiple times. • Use counter controlled variation if you know in advance the number of times the loop will execute • Use sentinel controlled variation if you don’t know in advance how many times the loop will execute • Loop conditions can be controlled by simple or complex boolean expressions. 35