6-Consolidation.pptx
Document Details
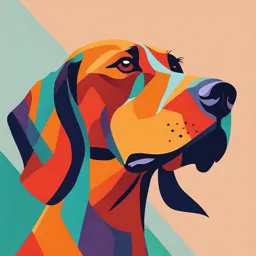
Uploaded by IndulgentPelican
University of Doha for Science and Technology
Full Transcript
COM136 – Software Development 1 Consolidation / Revision 1 Learning Outcomes • At the end of this lecture you should • Fully understand the various types of selection and repetition statements. • Learn how to write programs that use these constructs. • Understand that algorithms can be constructe...
COM136 – Software Development 1 Consolidation / Revision 1 Learning Outcomes • At the end of this lecture you should • Fully understand the various types of selection and repetition statements. • Learn how to write programs that use these constructs. • Understand that algorithms can be constructed using combinations of these statements. • Understand the purpose of source code management systems 2 Review Selection - if The if statement allows code (inside {}) to be executed only when a boolean condition is true if ( condition ) { // code to be executed } Review Selection – if (tutorial 1 ) int rentalDays = Console.getInt(“Number of rental days: ”); // Calculate the cost when each day costs £55 double cost = rentalDays * 55.0; // check if discount due if (rentalDays >= 7) { Use selection double discount = cost / 100 * 25; (if) to conditionally cost = cost – discount; apply a } discount System.out.println(“Rental Cost £ “ + String.format(”%.2f”,cost)); Using constants for 55.0 & 25 would be Review Selection – if-else The if-else statement allows one of two code blocks {} to be executed depending on boolean condition if ( condition ) { // code to be executed when true } else { // code to be executed when false Review Selection – if-else (tutorial 2 ) double SRATE int standard ”); int overtime ”); double wages wages = 10.0, ORATE = 15.0; = Console.getInt(“Number of standard hours: = Console.getInt(“Number of overtime hours: = standard * SRATE; // calculate standard Use selection (if// calculate overtime (only allowed up to 10 hours else) to limit overtime) overtime if (overtime > 10) { payment else wages = wages + (10 * ORATE); apply default } else { Review Selection – if-else-if… The if-else-if… statement allows more than two code blocks to be executed using multiple conditions if ( condition1 ) { // code to be executed when true } else if ( condition2 ) { // code to be executed when false } // optional else-if / else statements Review Selection - if-else-if (Question) Ask two users to let us know if they are feeling great Then check if both are great only one is great or neither are great and and print one of the following messages “both of us are great" “one of us is great” Review Selection – if-else (tutorial 2 ) boolean imGreat = Console.getBoolean("Am I great?: "); boolean yourGreat = Console.getBoolean("Are You great?: “); if (imGreat && yourGreat) { System.out.println("both of us are great"); } else if (imGreat || yourGreat) { System.out.println("one of us is great"); } else { System.out.println(”looks like neither of us is great” "); Because first two if statements were } false the default else will handle scenario when both of us are not great Review Selection – if-else-if... (tutorial 4 ) double double double double balance = Console.getDouble(“Balance £ ”); deposits = Console.getDouble(“Deposits £ ”); withdrawals = Console.getDouble(“Withdrawals £ ”); tax = 0.0; // calculate tax due Use selection if (deposits > 5000) { (if-else-if) to tax = (deposits–1000) / 100 * 1; //1% add one of two } else if (deposits > 1000 { tax rates tax = (deposits–1000) / 100 * 0.5; //0.5%(or none if deposits < } 1000) Review Selection – if-else-if (tutorial 5) Many combinations of if-else-if are possible but not always easy to make correct choice. Practice!@! An architect’s fee is calculated as a % of the cost 8% of first £50,000 of the cost of the building then either 3% on the remainder if the remainder is less than or equal to £80,000 or Review Selection – if-else-if (tutorial 5) v1 double cost = Console.getDouble(“Enter building cost £ “); double fees = 0.0, excessFees = 0.0; if (cost <= 50000) { // 8% band fees = cost / 100 * 8; } else if ((cost – 50000) <= 80000) { // 3% band fees = 50000 / 100 * 8; excessFees = (cost – 50000) / 100 * 3; } else { // 2% band fees = 50000 / 100 * 8; excessFees = (cost – 50000) / 100 * 2; } fees = fees + excessFees; OVERLY COMPLICATED REPETITIVE CODE IN GREEN Review Selection – if-else-if (tutorial 5) v2 double cost = Console.getDouble(“Enter building cost £ “); double fees = 0.0, excessFees = 0.0; if (cost <= 50000) { fees = cost / 100 * 8; } else { fees = 50000 / 100 * 8; double excess = cost – 50000; if (excess <= 80000) { excessFees = excess / 100 * 3; } else { excessFees = excess / 100 * 2; } fees = fees + excessFees } Using a Nested ifelse makes the algorithm clearer Review While – tutorial 6 Design an algorithm that will prompt a user to enter their age in years and months since last birthday (two separate inputs – years, months). The algorithm should calculate and display the users age in months. If the calculated months figure is greater than 500 then, three asterisks should be printed beside the month figure. The algorithm should continue processing Review While – tutorial 6 int years = Console.getInt("Age in years (0 to quit): "); int months = Console.getInt("Months since birthday: "); // output age in months int total = months + (years * 12); if (total > 500) { System.out.println(“Months = “ + total + “ ***”); } else { System.out.println(“Months = “ + total); } How do we repeat this until years is 0? Review While – tutorial 6 Couple of problems with this approach int years = Console.getInt("Age in years (0 to quit): "); int months = Console.getInt("Months since birthday: "); while (years != 0) { What if we entered 0 years? Why ask for months? // output age in months } Once in the loop, how do we get out? Review While – tutorial 6 Correct approach int years = Console.getInt("Age in years (0 to quit): "); while (years != 0) { int months = Console.getInt("Months since birthday: Now only ask for months while "); years != 0 // output age in months Once in the in loop, we ask(0 for next years years = Console.getInt("Age years to quit): "); } and allow possibility of exiting the loop Review While – tutorial 6 Incorrect approach We need to set years to an initial value to get into the loop (any value that is not 0) int years = 1; while (years != 0) { years = Console.getInt("Age in years (0 to quit): "); int months = Console.getInt("Months since birthday: "); // output age in months } To exit loop we enter years of 0, but it still asks us for months and calculates a result Practical 6 – Q2 optional Create a Java algorithm which will force the user to enter an table between 1 and 12 inclusive. The algorithm should then print the times tables for that number to the console. How do we force the user to enter a table number between 1-12?? Practical 6 – Q2 – while or if? Would this work? int table = Console.getInt(“Enter table:”); if (table < 1 || table > 12) { table = Console.getInt(“Enter table:”); } // print table Nonow - only allows 2 opportunities to enter correct table Practical 6 – Q2 – while or if? What type of loop do we need – counter or sentinel? We need a sentinel – the condition will be when the table entered is invalid (i.e. < 1 or > 12) int table = Console.getInt(“Enter table:”); while (table < 1 || table > 12) { table = Console.getInt(“Enter table 112:”); } Practical 7 – Challenge Question Create an algorithm to calculate the results of two people playing the “Rock Paper Scissors” game Both players input (R, P or S) The algorithm should determine the winner. Rules: Rock smashes Scissors Scissors cut Paper Paper covers Rock Print winner Source code management – (Version control) Source code management (SCM) is used to track modifications to a source code repository (a software application) over time. Modifications contribute to a release (new version of software) SCM allows us to review/undo changes to a code base (revision history) Generally helps manage a development teams contributions to a software product Source Code Management (SCM) Git is the most popular source code management system in use today (created by Linus Torvalds – creator of the Linux kernel) Github is a cloud based web application that allows developers to store their git repositories in the cloud and share repositories with other developers GitLab is an alternative to Github Both use the Git source code versioning Git & GitHub / GitLab make changes Source code directory push commit Staging (changes) Local Repositor y Interne t reset (commit) pull Remote Repositor y Summary • The while-loop is a common loop structure which allows us to repeat a sequence of instructions multiple times. • Use counter controlled variation if you know in advance the number of times the loop will execute • Use sentinel controlled variation if you don’t know in advance how many times the loop will execute • Loop conditions can be controlled by simple or complex boolean expressions. 26