5-Selection.pptx
Document Details
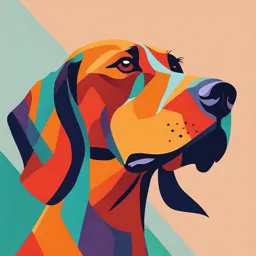
Uploaded by IndulgentPelican
University of Doha for Science and Technology
Full Transcript
1 Selection & Boolean Expressions COM136 – Software Development 1 Learning Outcomes • At the end of this lecture you should • Learn how to write programs that can make decisions (branching programs). • Learn how to evaluate and create Boolean expressions. • Be able to explain the Java selection s...
1 Selection & Boolean Expressions COM136 – Software Development 1 Learning Outcomes • At the end of this lecture you should • Learn how to write programs that can make decisions (branching programs). • Learn how to evaluate and create Boolean expressions. • Be able to explain the Java selection statement constructs used to implement branching programs. • Be able to identify errors in branching programs. • Be able to perform a dry run in a branching program to analyse what it does. 2 A Problem • Students have been given their grades (out of 100) and need to know whether they have passed. The pass mark is 40 in this test. • Write a program that asks a student for their mark and then tells them whether they have passed. 3 An Algorithm 1. PRINT “Enter mark” 2. INPUT mark 3. IF mark is greater than the pass mark then PRINT “Congratulations you have passed.” ELSE PRINT “Unfortunately you have failed”. ENDIF 4. PRINT “Thank you for using the marks checker” 4 Boolean Expressions • The statement “mark is greater than the pass mark” is an example of a boolean expression. • You are familiar with integer expressions such as (a + 5) * 7 • They evaluate to an integer value e.g. -5, 1, 42… • A boolean expression is one which evaluates to a boolean value i.e. true or false • Is the sun hot true 5 Relational Operators • Boolean expressions are constructed using the relational operators < > <= >= == != less than e.g. 5 < 6 greater than e.g. m > n less than or equal e.g. 5 <= (a+1) greater than or equal e.g. a >= 7 equals e.g. (a+1)==(b+1) not equals e.g. 5 != 6 • Their operands are integer values 6 Examples • Given mark=45 and n=25 what is value of following mark mark mark mark mark mark mark > 40 < 100 >= 45 <= 45 > (n*2) == n != n TRUE TRUE TRUE TRUE FALSE FALSE TRUE 7 Operator Precedence • Precedence determines the order in which operations are evaluated and the order is as shown below • 1. 2. 3. 4. () * / % + < <= = > >= • So for example expression count + 6 < 8 * b • is evaluated as (count + 6) < (8 * b) 8 Java If-Else Selection Statement - Syntax • We will use the Java if-else selection statement to implement this solution. The syntax is as follows: if (<boolean expression>) <statement s1> else <statement s2> • The ‘if’ and ‘else’ are reserved words in Java and give the if-else statement its structure. The <boolean expression> must be enclosed in ( ) 9 Java If-Else Selection Statement Semantics • When the if-else statement is encountered the <boolean expression> is evaluated, if it evaluates to true, then <statement s1> is executed and if it evaluates to false then <statement s2> is evaluated. false statement s2 expression true statement s1 10 In Java – (pass/fail program) import uulib.Console; public class PassFail1 { public static void main(String[] args) { final int PASS = 40; // declare constant pass mark //input and store the mark int mark = Console.getInt("Please enter your mark: "); if (mark > PASS) // test if mark is a pass System.out.println ("Congratulations you passed"); else System.out.println("Sorry you failed"); System.out.println("Thank you"); // print thank you message } } 11 Question 1 • Program PassFail1 contains a semantic error. Perform a dry run using the following test data for mark to find the error. 34 56 40 FALSE TRUE FALSE if (mark > PASS) System.out.println("Congratulations..."); else System.out.println("Sorry you failed"); Correct boolean expression if (mark >= PASS) ….. 12 Exercise • Write a program that inputs 2 values from the user and prints out the larger of the two. Algorithm INPUT value1 INPUT value2 IF value1 is larger than value2 then PRINT value1 ELSE PRINT value2 ENDIF 13 In Java import uulib.Console; public class Bigger1 { public static void main(String[] args) { // enter the numbers int num1 = Console.getInt("Enter first number"); int num2 = Console.getInt("Enter second number"); // test if num1 is greater than num2 if (num1 > num2) System.out.println(num1 + " is the largest"); else System.out.println(num2 + " is the largest"); } // end main } // end class 14 One way selection statement • An ‘if-statement‘ is a variation on the ‘if-else statement’ • It differs in that it has no ‘else’ part so it only has one branch, the true branch, which is executed if the condition is true, otherwise execution continues with the statement following the ‘if statement’. • Syntax of if Statement if (<boolean expression>) statement; 15 One way selection statement • For example: char grade = ‘F’; int mark = 50; if (mark >=70) grade = ‘A’; System.out.println(“Grade is “ + grade); • Here the grade printed is ‘F’ as it’s only changed to ‘A’ if the mark is at least 70. 16 If statement Semantics • The boolean expression is evaluated. If true then the statement is executed, if false then the statement is not executed. In both conditions execution continues with the statement following the if-statement. boolean expression true statement 17 Compound Statements • Consider the following. Is anything printed? char grade = ‘F’; int mark = 65; if (mark >= 70) grade = ‘A’; System.out.println(“Wow, grade: ”+ grade); Program Prints: Wow, grade : F boolean expression was false so only body of selection statement (grade=‘A’;) not executed. The print statement is not part of the if 18 Indentation is misleading Compound Statements • If either branch of an if..else contains more than one statement then curly brackets are needed on that branch compound statement . So following now prints correct message char grade = ‘F’; int mark = 65; if (mark >= 70) { grade = ‘A’; System.out.println(“Wow, grade: ”+ grade); } 19 Compound Statements • Another example if (mark >= 70) { grade = ‘A’; System.out.println(“Wow, grade :“ + grade); } else { grade = ‘F; System.out.println(“Oops, grade: ” + grade); } • Note the layout style used 20 Nested Selection Statements • Given the statement(s) in each branch of an if/if-else statement can be any valid java statement – then those branches could be other if/if-else statements. • A Problem: Consider that we need a program which accepts an exam mark entered by the user and it will print the grade achieved by the user. You may assume the usual A-D pass and F fail bands. 21 An Algorithm IF mark is greater than 69 grade = ‘A’ ELSE IF mark is greater than 59 grade = ‘B’ ELSE IF mark is greater than 49 grade = ‘C’ ELSE IF mark is greater than 39 grade = ‘D’ ELSE grade = ‘F’ END IF END IF END IF END IF 22 Java Code – very un-readable char grade; int mark = Console.getInt(“Enter Mark”); if (mark > 69) grade = ‘A’; else if (mark > 59) grade = ‘B’; else if (mark > 49) grade = ‘C’; else if (mark > 39) grade = ‘D’ else grade = ‘F’ Note how this layout ‘creeps’ across the screen! Lack of {} makes it unclear where if-else blocks start/end Unclear if the print statement is executed System.out.println(“Your grade was: “ + grade); 23 Nesting Layout - a clearer alternative if (mark > 69) { grade = ‘A’; } else if (mark > 59) { Note use of brackets grade = ‘B’; which allows multiple } else if (mark > 49) { statements in each ifgrade = ‘C’; else when required (compound } else if (mark > 39) { statements) grade = ‘D’; } else { This is the preferred grade = ‘F’; style } System.out.println(“Your grade was: “ + grade); 24 Layout Guidelines • Indent each branch of an if/if..else by the same amount • With nested ifs, be consistent with indentation style. • Put a blank line before and after each if/if..else statement to separate it out. • Use {} around if/if..else blocks (even if they only contain a single statement 25 Problem • A student must pass both coursework and exam elements of a module. The student must achieve at least 40 in both elements to pass the module. • Write a program to input the 2 marks and say whether or not the student passed or failed the module. 26 An Algorithm INPUT exam INPUT coursework IF exam >= 40 IF coursework >= 40 PRINT “student passed” ELSE PRINT “student failed” ENDIF ELSE PRINT “student failed” ENDIF 27 A Different Algorithm INPUT exam INPUT coursework IF exam >= 40 AND coursework >= 40 PRINT “student passed” ELSE PRINT “student failed” ENDIF We call this a compound Boolean expression AND is an example of a logical operator 28 The Logical Operators • Java logical operators produce boolean results • ! logical NOT • && logical AND • || logical OR • Often used to construct complex boolean expressions. e.g. assuming a = 1 and b = 4 • AND ( a <= l && b > 3 ) => TRUE • OR ( a == l || b == 5 ) => TRUE • NOT ( !(a == l) && b == 4 ) => FALSE • Alternative ( a != 1 to && b == 4 )expression? way write last 29 Operator Precedence 1. () 2. ! 3. * / % 4. + 5. < <= == > 6. != 7. && 8. || >= 30 Partial Java Solution ... final int PASS = 40; // constant pass mark // input marks int coursework = Console.getInt("Coursework mark: "); int exam = Console.getInt("Exam mark: "); // test if mark is a pass if (exam >= PASS && coursework >= PASS) { System.out.println("Congratulations you passed"); } else { System.out.println("Sorry you failed"); } ... 31 Another Example – Tutorial Question Assuming tax is deducted on deposits as follows double First 1000 = 0%, 1001-5000 = 0.5%, >5000 = deposit = 1500; double 1% deduction = 0; // test if tax deductions due if (deposit > 1000 && deposit <= 5000){ deduction = (deposit – 1000) / 100 * 0.5; } else { deduction = (deposit – 1000) / 100 * 1.0; } 32 Truth Tables We can use truth tables to describe the operation of the logical operators a false false true true b false true false true !a true a && b false a || b false !(a || b) true true false true false false false true false false true true false 33 Truth Tables - Example Consider the following expression if ( !done && count > MAX ){ System.out.println (“Finished”); } Under what conditions would println method be executed? done count > MAX !done !done && count > MAX false false true true false true false true true true false false false true false false 34 Using Brackets • We can use brackets to override normal precedence. • For example assuming a is 1 and b is 4 • ( !(a == l) && b == 2 ) Without brackets !a == 1 would generate a compiler error as ! cannot be applied to a as it is not a boolean value, but (a==1) is boolean. • Assuming a is 1 and b is 4, what are the results of the following expressions? • (a == 2 && b == 4 || a >= 1) • (a == 2 && (b == 4 || a >= 1)) 35 Using Brackets • Assuming a is 1 and b is 4, what are the results of the following expressions? Remember && has higher precedence than || Brackets have highest precedence (a == 2 && b == 4 || a >= 1) (a == 2 && (b == 4 || a >= 1)) 36 Using Brackets • We can also use brackets just to make statements more readable. • What is the order of evaluation in this expression? ( ((a >= 4) && (b < 3)) || (x == a) ) 1 2 4 3 5 The following version without brackets has the same value but is more difficult to read ( a >= 4 && b < 3 || x == a ) Practical Q4 A leap year is a year that is divisible by four and is not a century. Only exceptions are centuries that are divisible by 400 as these are leap years. IF ( year is div by 4 AND year is NOT a century OR year is div by 400 ) Summary • Programs can execute different instructions depending on the inputs by using selection statements if/if-else • Selection statements use boolean expressions to determine which branch to execute. • Boolean expressions are built using relational, equality and logical operators. • Selection statements can be nested to provide complex conditions. Proper layout is important when using nested selection statements to maintain readability. • Selection statements can use compound statements for their branches – allows more than one statement to be executed in a branch. 39