2_C Fundamentals.pdf
Document Details
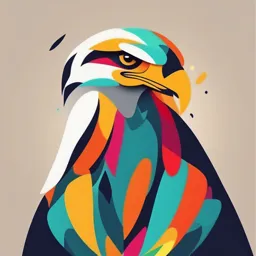
Uploaded by DoctorVisionaryAngel
Seneca Polytechnic
Full Transcript
PRG 155 – Programming Fundamentals Using C 2. C Programming Language Developed at AT&T Laboratories in 1972 by Dennis Ritchie for the UNIX operating system Building block for many known programming languages Used for: o Operating systems o Compilers o Ass...
PRG 155 – Programming Fundamentals Using C 2. C Programming Language Developed at AT&T Laboratories in 1972 by Dennis Ritchie for the UNIX operating system Building block for many known programming languages Used for: o Operating systems o Compilers o Assemblers o Editors o Network drivers o Utilities o Embedded systems o General-purpose programs Features of C programming language C is a structured programming language C is highly portable language – C program is platform independent. This applies to both, choice of operating system and hardware platform. C brings together the features of high-level programming languages and the bit manipulation capability. C is fast – Compilation and execution are faster than with most other programming languages. C can extend itself by adding functions to its collection of build-in functions. C is easy to learn – It has only 32 keywords. 1 PRG 155 – Programming Fundamentals Using C Structure of a C program Comments are optional; they are ignored by the compiler Preprocessor directive #include is used to access header files (files with extension.h) which contain a set of macro definitions and declarations of common C functions (build-in function). The actual executable code is stored in C libraries. It can be only one main( ) function in every C program. Global/local declarations state the program’s need for memory. { } are used to group statements together and to define the body of the function. For every open bracket {, there must be a closing bracket }. Statement return 0; is used to end the program and to return 0 to the operating system indicating normal termination. Non-zero value indicates abnormal termination and it is usually 1. C is case-sensitive language 2 PRG 155 – Programming Fundamentals Using C C Language Elements C Character Set Alphabets Uppercase: A – Z Lowercase: a –z Digits 0–9 Special characters -~‘!@#%^&*()_-+=|\{}[]:;"',.?/ White space characters blank space, new line, horizontal tab, carriage return, form feed C Keywords (Reserved Words) All keywords are in lowercase letters Cannot be used for identifiers (user-defined names) “32 Keywords in C Programming Language with their Meaning.” n.d. Online Image. BSC btechsmartclass. 23 Jan, 2017. http://www.btechsmartclass.com/CP/c-keywords.htm 3 PRG 155 – Programming Fundamentals Using C Identifiers Identifier is a user defined name given to variable, function, constant and/or other program entity. Rules for creating identifiers: Identifiers are case sensitive (varName is not the same as varname) Can only consist of letters (uppercase and lowercase), digits, and underscore (_) Cannot start with a digit (0-9) Underscore can be used as the first character (example: _result) Cannot contain any special character other than underscore (_) Cannot use reserved words (keywords) The identifier length limit is compiler dependent. Given names should be descriptive, meaningful, and unique. 4 PRG 155 – Programming Fundamentals Using C Function printf() printf() is a build-in C function used to perform output operations such as displaying data on the screen, sending data to printer or file. printf() is defined in “stdio.h” header file. When printf() is used in the program, the “stdio.h” header must be included as follows: #include Syntax: printf(“format_string”, variable1, variable2, variable3,...); where format_string is a string that can contain 3 types of elements: Plain characters that will be displayed on the screen (unchanged) Format specifiers (conversion specifiers) used as placeholders, which will be replaced by the values of variables listed after the format_string. o Format specifier begins with %, followed by the data type code. Data type code must match the variable data type. Example: %c for a variable of character data type o For each listed variable, there should be one format specifier. o Format specifiers are replaced in sequential order. Escape sequences used to control the cursor or insertion point Examples: Output: int size = 7; Welcome! float average = 80.5; a=3 b=7 printf("Welcome!\n"); printf("a=%d b=%d\n\n", 3, size); printf("Test Results:\nAverage=%.2f", average); Test Results: Average = 80.50 5 PRG 155 – Programming Fundamentals Using C Format Specifiers Format specifier Description Supported data types char %c Character unsigned char short unsigned short %d Signed Integer int long float %e or %E Scientific notation of float values double %f Floating point float float %g or %G Similar as %e or %E double short unsigned short %i Signed Integer int long %l or %ld or %li Signed Integer long %lf Floating point double %Lf Floating point long double unsigned int %lu Unsigned integer unsigned long short unsigned short %o Octal representation of Integer. int unsigned int long %p Address of pointer to void void * void * %s String char * unsigned int %u Unsigned Integer unsigned long short unsigned short Hexadecimal representation of %x or %X int Unsigned Integer unsigned int long %% Prints % character 6 PRG 155 – Programming Fundamentals Using C Escape Sequences Escape Name Description Sequence \a Alert Sounds a beep \b Back space Backs up 1 character \f Form feed Starts a new screen of page \n New line Moves to the beginning of next line \r Carriage return Moves to the beginning of current line \t Horizontal tab Moves to the next tab position \v Vertical tab Moves down a fixed amount \\ Back slash Prints a back slash \’ Single quotation Prints a single quotation \” Double quotation Prints a double quotation \? Question mark Prints a question mark References Tan, H.H., and T.B. D’Orazio. C Programming for Engineering & Computer Science. USA: WCB McGRaw-Hill. 1999. Print. Hock-Chuan, Chua. C programming Tutorial. Programming notes, n.d. Web. 23 Jan, 2017. https://www3.ntu.edu.sg/home/ehchua/programming/index.html BSC btechsmartclass. n.d. Web. 23 Jan, 2017. http://www.btechsmartclass.com/CP/computer-languages.htm 7