Humidity Sensors (Soil and DHT11) with Arduino
Document Details
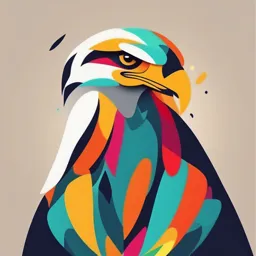
Uploaded by SpiritedHibiscus6249
University of Petra
Tags
Summary
This document provides an introduction to humidity sensors, focusing on soil moisture sensors and DHT11 sensors. It includes information about theory of operation, components, coding examples and circuit diagrams. The content details how to use Arduino to measure and display humidity data.
Full Transcript
Humidity Sensors (Soil and DHT11)with Arduino Introduction to Humidity Sensors What is a Humidity Sensor? A humidity sensor measures the amount of water vapor in the air. Applications include weather monitoring, greenhouse control, and home automation. Types of Humidity...
Humidity Sensors (Soil and DHT11)with Arduino Introduction to Humidity Sensors What is a Humidity Sensor? A humidity sensor measures the amount of water vapor in the air. Applications include weather monitoring, greenhouse control, and home automation. Types of Humidity Sensors Soil moisture sensors (analog and digital output). DHT sensors (e.g., DHT11 and DHT22 for air humidity and temperature). Components 1.Soil Moisture Sensor Kit 1.Probes: Detect soil moisture levels. 2.Control Module: Includes an LM393 comparator and potentiometer. 3.Pins: VCC, GND, Digital, Analog. 2.DHT11 Sensor 1.Measures air temperature and humidity. 2.Has digital output with calibrated signals. Part 1: Soil Moisture Sensor with Arduino Theory of Operation The soil moisture sensor measures resistance in the soil. Wet Soil: Low resistance, high conductivity. Dry Soil: High resistance, low conductivity. Outputs: Analog Output: Continuous values based on moisture level. Digital Output: High or low based on the threshold set by the potentiometer. Circuit Diagram 1.Connect the VCC pin to the 5V pin on Arduino. 2.Connect the GND pin to GND on Arduino. 3.Connect the A0 (Analog) pin to an analog input pin (e.g., A0) on Arduino. 4.Connect the D0 (Digital) pin to a digital input pin (optional). Coding const int analogPin = A0; void setup() { Serial.begin(9600); } void loop() { int analogValue = analogRead(analogPin); int moisturePercentage = map(analogValue, 0, 1023, 100, 0); // float moisture_percentage = ( 100 - ( (sensorValue/1023.00) * 100 ) Serial.print("Moisture Percentage: "); Serial.println(moisturePercentage); delay(1000); } Low Analog Value (closer to 0): Indicates high moisture in the soil (wet soil). High Analog Value (closer to 1023): Indicates low moisture in the soil (dry soil). If we want to express the soil moisture as a percentage, we typically want: 100% to represent very wet soil. 0% to represent very dry soil. Using map(analogValue, 0, 1023, 100, 0) achieves this mapping: Part 2: DHT11 Sensor with Arduino Theory of Operation The DHT11 sensor provides digital temperature and humidity data. Uses a single-wire communication protocol for simple interfacing. Features of DHT11 Humidity range: 20-90% RH with ±5% accuracy. Relative Humidity (RH): This is the amount of water vapor present in the air compared to the maximum amount of water vapor the air can hold at a given temperature, expressed as a percentage. 0% RH: Completely dry air. 100% RH: Air that is fully saturated with water vapor. 20-90% RH: This indicates the sensor's operational range. It can reliably measure relative humidity levels between 20% (dry air) and 90% (very humid air). Outside this range, the sensor may not provide accurate readings. Temperature range: 0-50°C with ±2°C accuracy. Circuit Diagram Connect VCC pin of DHT11 to 5V on Arduino. Connect GND pin of DHT11 to GND on Arduino. Connect the Signal pin of DHT11 to a digital input pin (e.g., D2). Arduino Code and DHT library Install the DHT library from the Arduino IDE Library Manager. Functions: DHT dht(DHTPIN, DHTTYPE); is used to create and configure an instance of the DHT sensor object dht: This is the name of the object you're creating. You can name it whatever you like (e.g., myDHT). DHTPIN: The Arduino pin connected to the signal pin of the DHT sensor (e.g., 2 or 4). DHTTYPE: The type of the DHT sensor you're using. This could be DHT11, DHT22, or DHT21, depending on your hardware. DHT library DHT.begin() Description: Initializes the DHT sensor. This function must be called in the setup() function before any readings are taken. Usage: Use this function once in your setup() to initialize the sensor. dht.readTemperature() Description: Reads the temperature in Celsius from the sensor. Parameters: false (default): Returns the temperature in Celsius. true: Returns the temperature in Fahrenheit. Returns: A floating-point value representing the temperature or NAN if the reading failed. Usage: Use this function to get the current temperature reading. DHT library dht.readHumidity() Description: Reads the relative humidity from the sensor. Returns: A floating-point value representing the humidity percentage or NAN if the reading failed. Usage: Use this function to get the current humidity reading. dht.computeHeatIndex(temp, hum, isFahrenheit) Description: Calculates the heat index (apparent temperature) based on the current temperature and humidity. Parameters: temp: Temperature value (float). hum: Humidity value (float). isFahrenheit (optional): true if temp is in Fahrenheit; otherwise false (default). Returns: A floating-point value representing the heat index. Usage: Use this function to calculate how hot it feels, considering both temperature and humidity. DHT library isnan(value) Description: Checks if the sensor reading returned NAN (not a number), which indicates a failed reading. Parameters: value: The reading to check (e.g., temperature or humidity). Returns: true if the reading is NAN, otherwise false. Usage: Use this to verify if the sensor reading is valid. Code example #include "DHT.h" #define DHTPIN 2 #define DHTTYPE DHT11 DHT dht(DHTPIN, DHTTYPE); void setup() { Serial.begin(9600); dht.begin(); } void loop() { float humidity = dht.readHumidity(); float temperature = dht.readTemperature(); if (isnan(humidity) || isnan(temperature)) { Serial.println("Failed to read from DHT sensor!"); return; } Serial.print("Humidity: "); Serial.print(humidity); Serial.print(" %\t"); Serial.print("Temperature: "); Serial.print(temperature); Serial.println(" °C"); delay(2000); } Example with LCD example Arduino program that reads data from both a Soil Moisture Sensor and a DHT11 Sensor, and displays the results on an I2C LCD. Required Components Soil Moisture Sensor DHT11 Sensor I2C LCD Display Arduino Board Jumper Wires Breadboard Circuit Connections Soil Moisture Sensor: VCC → 5V GND → GND A0 → A0 (Analog pin on Arduino) DHT11 Sensor: VCC → 5V GND → GND Signal → Digital pin 2 I2C LCD: SDA → A4 (on Arduino Uno) or dedicated SDA pin SCL → A5 (on Arduino Uno) or dedicated SCL pin VCC → 5V GND → GND Arduino Code #include #include #include // Constants for DHT11 #define DHTPIN 8 // Signal pin connected to digital pin 8 #define DHTTYPE DHT11 // Define DHT11 type // Initialize DHT object DHT dht(DHTPIN, DHTTYPE); // Initialize I2C LCD (adjust address as needed) LiquidCrystal_I2C lcd(0x27, 16, 2); // 0x27 is a common I2C address for LCD // Soil Moisture Sensor pin const int soilPin = A0; Arduino Code void setup() { Serial.begin(9600); // Initialize DHT sensor dht.begin(); // Initialize LCD lcd.init(); lcd.backlight(); // Display a startup message lcd.setCursor(0, 0); lcd.print("Initializing..."); delay(2000); lcd.clear(); } void loop() { float temperature = dht.readTemperature(); float humidity = dht.readHumidity(); // Check if DHT readings are valid if (isnan(temperature) || isnan(humidity)) { lcd.setCursor(0, 0); lcd.print("DHT Error"); lcd.setCursor(0, 1); lcd.print("Check Sensor"); } else { // Display temperature and humidity on LCD lcd.setCursor(0, 0); lcd.print("Temp: "); lcd.print(temperature); lcd.print("C "); lcd.setCursor(0, 1); lcd.print("Hum: "); lcd.print(humidity); lcd.print("%"); } delay(2000); // Read soil moisture sensor value int soilValue = analogRead(soilPin); int soilMoisture = map(soilValue, 0, 1023, 100, 0); // Map to percentage // Display soil moisture on LCD lcd.clear(); lcd.setCursor(0, 0); lcd.print("Soil Moisture:"); lcd.setCursor(0, 1); lcd.print(soilMoisture); lcd.print("%"); delay(2000); // Delay before updating the display }