Repetition and Loops: C Programming Lesson #5
Document Details
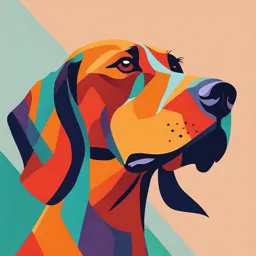
Uploaded by CoolestSard3147
Ryerson University
Denis Hamelin
Tags
Summary
This document is lesson #5 about repetition and loops in C programming. It covers various types of loops including counting loops, sentinel-controlled loops, end-of-file controlled loops, input validation loops, general conditional loops, and nested loops. Code examples are provided for each type of loop.
Full Transcript
Lesson #5 Repetition and Loops 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University Repetition and Loops Loop: A control structure that repeats a group of steps (statements) in a program. Loop body: Contains the statements that are repeated in the loop....
Lesson #5 Repetition and Loops 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University Repetition and Loops Loop: A control structure that repeats a group of steps (statements) in a program. Loop body: Contains the statements that are repeated in the loop. 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University Do I Need a Loop? 1. Are there any steps you must repeat to solve the problem? If yes, you need a loop. 2. Do you know in advance the number of repetitions? If yes, you need a counting loop. 3. Do you know when to stop the repetition? If not, you will not be able to program the loop. 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University Types of Loops Counting Loop: A loop with a fixed number of repetitions. Ex: Repeat 100 times... Sentinel-Controlled Loop: A loop that reads values from a file or keyboard and stops reading when a certain value (called a sentinel) is read. Ex: Read numbers continuously until you encounter a value of -99. 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University Types of Loops End-of-File Controlled Loop: A loop that reads values from a file and stops when there are no more values to read. Ex: Read numbers continuously until you encounter the end of the file. Input Validation Loop: A loop that keeps asking a value from the user until the user gets it right. Ex: Keep asking the user for a positive number until the user enters one. 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University Types of Loops General Conditional Loop: A loop that checks a certain condition and repeats the statements in the loop body if the condition is true. When the condition is false, the loop is ended and the statements are not repeated. This kind of loop encompasses all the other kinds. Ex: Print numbers on the screen while the numbers are below 100. 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University Loops in C In C, all loop are implemented with general conditional loops. By programming them properly, we can achieve all the types of loops. For example, a counting loop (repeat 100 times) will be done by starting a variable at 1, then have a condition to stop the loop when the variable becomes larger than 100. Inside the loop the program will add 1 to the variable at each repetition. 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University The while Statement The while statement permits the repetition of the statements until the condition becomes false. Syntax: while (condition) { statements executed/repeated if condition is true } 5. Repetition and Loops - Copyright © Denis Hamelin - Ryerson University A Counting Loop int n; n = 1; while (n