Javascript II Lecture Notes PDF
Document Details
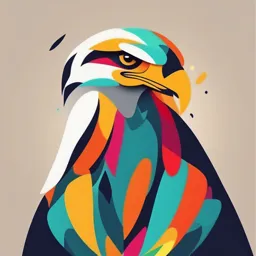
Uploaded by RockStarGraph3453
MSA University
Tags
Summary
This document covers topics in JavaScript, including constant variables, functions, objects, and HTML events. It includes examples and code.
Full Transcript
CS283 Lecture 05 Introduction to JavaScript - II Constant Variables Variables defined with const cannot be Redeclared. Variables defined with const cannot be Reassigned. Variables defined with const have Block Scope. Functions in Javascript ❑A JavaScript function is a block of cod...
CS283 Lecture 05 Introduction to JavaScript - II Constant Variables Variables defined with const cannot be Redeclared. Variables defined with const cannot be Reassigned. Variables defined with const have Block Scope. Functions in Javascript ❑A JavaScript function is a block of code designed to perform a particular task. ❑A JavaScript function is executed when it is invoked. function toCelsius(fahrenheit) { return (5/9) * (fahrenheit-32); } document.getElementById(“p1").innerHTML = toCelsius(85); 3 JavaScript Objects A JavaScript object is a flexible collection of key-value pairs. It allows you to group related data (properties) and behavior (methods). Objects are variables with set of properties and set of functions. const car = {type:“Mercedes", model:“GLC", color:“Black"}; const person = { firstName: “Ahmed", lastName: “Ali", age: 40, eyeColor: "blue" }; 4 JavaScript Objects To retrieve an object property: objectName["propertyName"] OR objectName.propertyName person["lastName"]; OR person.lastName; const person = { firstName: “Ahmed", lastName : “Ali", id : 12345, fullName : function() { return this.firstName + " " + this.lastName; } 5 JavaScript Objects objectName["propertyName"] OR objectName.propertyName person["lastName"]; OR person.lastName; const person = { firstName: “Ahmed", lastName : “Ali", id : 12345, fullName : function() { return this.firstName + " " + this.lastName; } }; 6 JavaScript Objects JavaScript Objects To call an object method: objectName.methodName(); name = person.fullName(); name = person.fullName→will return the function definition Code Examples: https://www.w3schools.com/js/tryit.asp?filename=tryjs_objects_functio n https://www.w3schools.com/js/tryit.asp?filename=tryjs_objects_metho d 7 HTML Events ❑HTML event can be something the browser does, or something a user does. An HTML web page has finished loading An HTML input field was changed An HTML button was clicked ❑ 8 HTML Element - Example HTML – Element.. Example The time is? 9 HTML Events HTML Events The time is? Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_events1 10 HTML Events Key HTML Events Event Description onchange An HTML element has been changed onclick The user clicks an HTML element onmouseover The user moves the mouse over an HTML element onmouseout The user moves the mouse away from an HTML element onkeydown The user pushes a keyboard key onload The browser has finished loading the page 11 12 Strings in Javascript ❑Single or double quotes can be used to define a string: let studentName1 = "Ahmed"; // Double quotes let studentName1 = 'Ahmed'; // Single quotes ❑Quotes can be used within a string, as long as they don't match the quotes surrounding the string let answer1 = "It's alright"; let answer2 = "He is called 'Johnny'"; let answer3 = 'He is called "Johnny"'; 13 q Example: let text= 'It\'s alright.’; q Code Example: https://www.w3schools.com/js/tryit.asp ?filename=tryjs_string_escape_quotes2 Escape Characters 14 Strings Properties and Methods 15 String Methods and Properties 16 Length Property ❑ Length Property: let text = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let length = text.length; ❑Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_string_length 17 Variable Substitutions let firstName = “Mohamed"; let lastName = “Ahmed"; let text = `Welcome ${firstName}, ${lastName}!`; Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_templates_variables slice(start, end) substring(start, end) String Functions substr(start, length) Note: The first position (index) of a string is 0. 10 ❑ Extracts a part of a string and returns the extracted part in a new string. ❑ Example: let str = "Apple, Banana, Kiwi"; let part = str.slice(7, 13); Str.Slice() ❑Extracts a part of a string from position 7 to position 13 (13 not included): ❑Output→ Banana ❑ Code Example: ❑https://www.w3schools.com/js/tr yit.asp?filename=tryjs_string_slice 20 ❑ If the second parameter was omitted, the method will slice out the rest of the string ❑let part = str.slice(7); ❑ Output→ Banana, kiwi ------------------------------------------ ❑If a parameter is negative, the position is counted from the end of the string. let str = "Apple, Banana, Kiwi"; Str.Slice() let part = str.slice(-12, -6); Output→ Banana -------------------- let part = str.slice(-12); Output→ ???? ----------- Code Example: https://www.w3schools.com/js/tryit.asp?filename=tr yjs_string_slice_rest_negative 21 Substr() Method ❑The difference between slice(), and substr() is that the second parameter specifies the length of the extracted part. let str = "Apple, Banana, Kiwi"; let part = str.substr(7, 6); ❑Output→ Banana If the second parameter was omitted, substr() will slice out the rest of the string. let str = "Apple, Banana, Kiwi"; let part = str.substr(7); Output→ Banana, Kiwi 22 Substr() Method let str = "Apple, Banana, Kiwi"; let part = str.substr(-4); Output→ Kiwi If the first parameter is negative, the position counts from the end of the string. Code Example: https://www.w3schools.com/js/tryit.asp?filename =tryjs_string_substr2 23 Replace () ❑The replace() method does not change the string it is called on. ❑The replace() method returns a new string. ❑The replace() method replaces only the first match ❑ replace() method is case sensitive. let text = "MSA students and MSA staff!"; let newText = text.replace(“MSA", “CS"); Output→ CS students and MSA staff!"; Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_string_replace_first 24 Concat (); ❑joins two or more strings: let text1 = “First"; let text2 = “Name"; let text3 = text1.concat(" ", text2); Output→ First Name Code example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_string_concat 25 More String Methods A string is converted to upper case with toUpperCase(): A string is converted to lower case with toLowerCase(): let text1 = "Hello World!"; let text2 = text1.toUpperCase(); 26 More String Methods The trim() method removes whitespace from both sides of a string let text1 = " welcome! "; let text2 = text1.trim(); ❑ trimStart() ❑TrimEnd() ❑Code Example: ❑https://www.w3schools.com/js/tryit. asp?filename=tryjs_string_trimstart 27 split(); A string can be converted to an array with the split() method: text.split(",") // Split on commas text.split(" ") // Split on spaces text.split("|") // Split on pipe let text = "a b c d e f"; const myArray = text.split(“ "); myArray→ {a,b,c,d,e,f} Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_string_split 28