Chapter 4 Mathematical Functions, Characters, and Strings PDF
Document Details
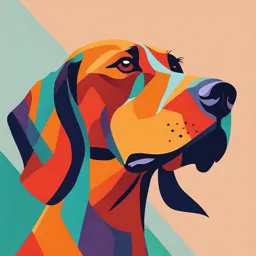
Uploaded by RenownedHeliotrope6321
Abu Dhabi University
2015
Liang
Tags
Summary
This document is chapter 4 of a textbook on Java programming, focusing on mathematical functions, characters, and strings. It covers methods in the Math class, representing and handling characters and strings, along with examples and explanations. The document also discusses topics like conversions, comparisions, and formatting strings.
Full Transcript
Chapter 4 Mathematical Functions, Characters, and Strings Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved....
Chapter 4 Mathematical Functions, Characters, and Strings Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 1 Motivations Suppose you need to estimate the area enclosed by four cities, given the GPS locations (latitude and longitude) of these cities, as shown in the following diagram. How would you write a program to solve this problem? You will be able to write such a program after completing this chapter. Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 2 Objectives To solve mathematics problems by using the methods in the Math class (§4.2). To represent characters using the char type (§4.3). To encode characters using ASCII and Unicode (§4.3.1). To represent special characters using the escape sequences (§4.4.2). To cast a numeric value to a character and cast a character to an integer (§4.3.3). To compare and test characters using the static methods in the Character class (§4.3.4). To introduce objects and instance methods (§4.4). To represent strings using the String objects (§4.4). To return the string length using the length() method (§4.4.1). To return a character in the string using the charAt(i) method (§4.4.2). To use the + operator to concatenate strings (§4.4.3). To read strings from the console (§4.4.4). To read a character from the console (§4.4.5). To compare strings using the equals method and the compareTo methods (§4.4.6). To obtain substrings (§4.4.7). To find a character or a substring in a string using the indexOf method (§4.4.8). To program using characters and strings (GuessBirthday) (§4.5.1). To convert a hexadecimal character to a decimal value (HexDigit2Dec) (§4.5.2). To revise the lottery program using strings (LotteryUsingStrings) (§4.5.3). To format output using the System.out.printf method (§4.6). Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 3 Mathematical Functions Java provides many useful methods in the Math class for performing common mathematical functions. Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 4 The Math Class Class constants: – PI –E Class methods: – Trigonometric Methods – Exponent Methods – Rounding Methods – min, max, abs, and random Methods Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 5 Trigonometric Methods sin(double a) Examples: cos(double a) Math.sin(0) returns 0.0 tan(double a) Math.sin(Math.PI / 6) returns 0.5 acos(double a) Math.sin(Math.PI / 2) asin(double a) returns 1.0 Math.cos(0) returns 1.0 atan(double a) Math.cos(Math.PI / 6) returns 0.866 Math.cos(Math.PI / 2) Radians returns 0 toRadians(90) Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 6 Exponent Methods exp(double a) Examples: Returns e raised to the power of a. log(double a) Math.exp(1) returns 2.71 Math.log(2.71) returns 1.0 Returns the natural logarithm of a. Math.pow(2, 3) returns 8.0 log10(double a) Math.pow(3, 2) returns 9.0 Returns the 10-based logarithm of Math.pow(3.5, 2.5) returns a. 22.91765 pow(double a, double b) Math.sqrt(4) returns 2.0 Math.sqrt(10.5) returns 3.24 Returns a raised to the power of b. sqrt(double a) Returns the square root of a. Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 7 Rounding Methods double ceil(double x) x rounded up to its nearest integer. This integer is returned as a double value. double floor(double x) x is rounded down to its nearest integer. This integer is returned as a double value. double rint(double x) x is rounded to its nearest integer. If x is equally close to two integers, the even one is returned as a double. int round(float x) Return (int)Math.floor(x+0.5). long round(double x) Return (long)Math.floor(x+0.5). Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 8 Rounding Methods Examples Math.ceil(2.1) returns 3.0 Math.ceil(2.0) returns 2.0 Math.ceil(-2.0) returns –2.0 Math.ceil(-2.1) returns -2.0 Math.floor(2.1) returns 2.0 Math.floor(2.0) returns 2.0 Math.floor(-2.0) returns –2.0 Math.floor(-2.1) returns -3.0 Math.rint(2.1) returns 2.0 Math.rint(2.0) returns 2.0 Math.rint(-2.0) returns –2.0 Math.rint(-2.1) returns -2.0 Math.rint(2.5) returns 2.0 Math.rint(-2.5) returns -2.0 Math.round(2.6f) returns 3 Math.round(2.0) returns 2 Math.round(-2.0f) returns -2 Math.round(-2.6) returns -3 Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 9 min, max, and abs max(a, b)and min(a, b) Examples: Returns the maximum or minimum of two parameters. Math.max(2, 3) returns 3 abs(a) Math.max(2.5, 3) returns Returns the absolute value of the 3.0 parameter. Math.min(2.5, 3.6) random() returns 2.5 Returns a random double value Math.abs(-2) returns 2 in the range [0.0, 1.0). Math.abs(-2.1) returns 2.1 Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved. 10 The random Method Generates a random double value greater than or equal to 0.0 and less than 1.0 (0 = 'A' && ch = 'a' && ch = '0' && ch