Lesson #4: Logical Operators and Selection Statements
Document Details
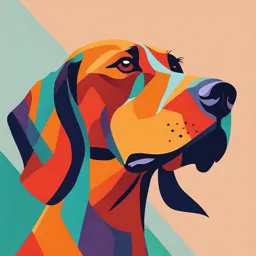
Uploaded by CoolestSard3147
Ryerson University
Denis Hamelin
Tags
Summary
This lesson focuses on Logical Operators and Selection Statements using C. Key topics covered include control structures, conditions, logical operators (AND, OR, NOT), and operator precedence. It also covers conditional statements such as 'if', 'else', and 'switch' statements.
Full Transcript
Lesson #4 Logical Operators and Selection Statements 4. Logical Operators and Selection Statements - Copyright © Denis Hamelin - Ryerson University Control Structures Control structures combine individual instructions into a single logical unit with one entry point a...
Lesson #4 Logical Operators and Selection Statements 4. Logical Operators and Selection Statements - Copyright © Denis Hamelin - Ryerson University Control Structures Control structures combine individual instructions into a single logical unit with one entry point at the top and one exit point at the bottom. 3 kinds of control structures: Sequence (default control structure) Selection (branches) Repetition (loops) 4. Logical Operators and Selection Statements - Copyright © Denis Hamelin - Ryerson University Conditions A condition is an expression that is either true or false. Ex: temperature=28; temperature < 0 will be false temperature >20 will be true Comparison (relational) operators are: < > = == != 4. Logical Operators and Selection Statements - Copyright © Denis Hamelin - Ryerson University Logical Operators A logical expression contains one or more comparison and/or logical operators. The logical operators are: &&: the and operator, true only when all operands are true. ||: the or operator, false only when all operands are false. !: the not operator, false when the operand is true, true when the operand is false. 4. Logical Operators and Selection Statements - Copyright © Denis Hamelin - Ryerson University Updated Operator Precedence Rule 2.1 Function calls 2.2 Unary operators (-, +, !, (int), (double)) 2.3 *, /, % 2.4 +, - 2.5 2.6 ==, != 2.7 && 2.8 || 2.9 = (assignment operator) 4. Logical Operators and Selection Statements - Copyright © Denis Hamelin - Ryerson University Fast Evaluation of Logical Expressions int x=3, y=4, z=10, w; w = x == 3 || y < 10; is w true or false? w = x%3-z=x/y || x 4 4. Logical Operators and Selection Statements - Copyright © Denis Hamelin - Ryerson University Building Logical Expressions Are x and y both greater than 10? x > 10 && y > 10 Is either x equal to 1 or 3? x == 1 || x == 3 Is x between y and z? x >= y && x