Input And Output - Intro to Programming in Python PDF
Document Details
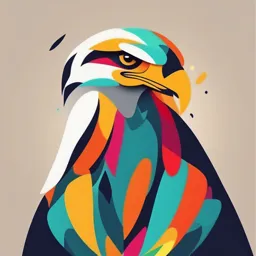
Uploaded by zarman145
Robert Sedgewick, Kevin Wayne, Robert Dondero
Tags
Summary
These slides cover input and output in Python programming. Topics include standard input and output, drawing, processing audio, and manipulating sound waves. The slides are designed to provide examples of abstractions.
Full Transcript
INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO 4. Input and Output 1.5 https://introcs.cs.princeton.edu/python...
INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO 4. Input and Output 1.5 https://introcs.cs.princeton.edu/python Slides ported to Python by James Pope Last updated on 2023/10/20 08:43 INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO 4. Input and Output Standard input and output Standard drawing Fractal drawings Animation Standard audio Basic building blocks for programming any program you might want to write object s functions and modules graphics, sound, and image I/O array s conditionals and Ability to interact with the outside world loops Mat text h I/O primitive data assignment types statements 3 Input and output Goal: Write Python programs that interact with the outside world via input and output devices. Typical INPUT devices Keyboard Trackpad Storage Network Camera Microphone Typical OUTPU T devices Storage Network Printer Speakers Display Our approach. Define input and output abstractions. Use operating system (OS) functionality to connect our Python programs to actual devices. 4 Abstraction plays an essential role in understanding computation. Interested in thinking more deeply about this concept? Consider taking a philosophy course. An abstraction is something that exists only as an idea. Example: "Printing" is the idea of a program producing text as output. Good abstractions simplify our view of the world, by unifying diverse real-world artifacts. This lecture. Abstractions for delivering input to or receiving output from our programs. 5 Quick review Terminal. An abstraction for providing input and output to a program. Input from command line % python3 drawcards.py 10 7♠ 2♥ Q♦ A♠ Q♠ 2♦ Q♥ 6♦ 5♥ 10♦ Output to standard output stream Virtual VT-100 terminal 6 Input-output abstraction (so far) A mental model of what a Python program does. command-line arguments Python program standard output stream 7 Review: command-line input Command-line input. An abstraction for providing arguments (strings) to a program. Basic properties Strings you type after the program name are available as argv, argv,... at run time. Arguments are available when the program begins execution. Need to call system conversion methods to convert the strings to other types of data. import sys import stdio import random % python randomint.py 6 def main(): 3 N = int(sys.argv) r = random.random() % python randomint.py 10000 t = int(r * N) 3184 stdio.writeln(t) if __name__ == '__main__': main() 8 Review: standard output Infinity. An abstraction describing something having no limit. Standard output stream. An abstraction for an infinite output sequence. Basic properties Strings from stdio.writeln() are added to the end of the standard output stream. Standard output stream is sent to terminal application by default. import sys import stdio import stdrandom % python randomseq.py 4 % python randomseq.py 1000000 0.9320744627218469 0.09474882292442943 0.4279508713950715 0.2832974030384712 def main(): 0.08994615071160994 0.1833964252856476 N = int(sys.argv) 0.6579792663546435 0.2952177517730442 for i in range(N): 0.8035985765979008 stdio.writeln( stdrandom.uniform() ) 0.7469424300071382 0.5835267075283997 No limit on amount of if __name__ == '__main__': main() 0.3455279612587455 output... 9 Improved input-output abstraction Add an infinite input stream. command-line standard input stream arguments Python program standard output stream 10 Standard input Infinity. An abstraction describing something having no limit. Standard input stream. An abstraction for an infinite input sequence. standard input stream Python program Advantages over command-line input Can provide new arguments while the program is executing. No limit on the amount of data we can input to a program. Conversion to primitive types is explicitly handled (stay tuned). 1 stdio library Developed for this course, but broadly useful Implement abstractions invented for UNIX in the 1970s. Available for download at booksite. module stdio bool isEmpty() true iff no more values int readInt() standard input stream read a value of type int float readFloat() read a value of type double bool readBool() read a value of type boolean Python program str readString() read a value of type String str[] readAllStrings() read the rest of the text 12 stdio library Developed for this course, but broadly useful Implement abstractions invented for UNIX in the 1970s. Available for download at booksite. stdio write(s) put s on the output stream Python program writeln() put a newline on the output stream writeln(String s) put s, then a newline on the stream writef(String f,...) formatted output standard output stream Q. These are similar to the print() function. Why not just use print()? A. We provide a consistent set of simple I/O abstractions in one place. A. We can make output independent of system, language, and locale. 13 writef Input/Output warmup Interactive input Prompt user to type inputs on standard input stream. Mix input stream with output stream. import stdio import sys def main(): % python addtwo.py stdio.write('Type the first integer: ') Type the first integer: 1 x = stdio.readInt() Type the second integer: 2 stdio.write('Type the second integer: ') Their sum is 3 y = stdio.readInt() summation = x + y stdio.writeln('Their sum is ' + str(summation) ) if __name__ == '__main__': main() 15 Input application: average the numbers on the standard input stream Average import sys import stdio Read a stream of numbers. def main(): Compute their average. total = 0.0 n = 0 Q. How do I specify the end of the stream? while not stdio.isEmpty(): number = stdio.readFloat() A. (standard for decades). total = total + number n = n + 1 A. (Windows). average = total / n stdio.writeln( str(average) ) if __name__ == '__main__': main() Key points No limit on the size of the input stream. % python average.py 10.0 5.0 6.0 Input and output can be interleaved. 3.0 7.0 32.0 10.5 16 Summary: prototypical applications of standard output and standard input randomseq.py: Generate a stream of random numbers average.py: Compute the average of a stream of numbers import sys import sys import stdio import stdio import stdrandom def main(): def main(): total = 0.0 N = int(sys.argv) n = 0 for i in range(N): while not stdio.isEmpty(): stdio.writeln( stdrandom.uniform() ) number = stdio.readFloat() total = total + number if __name__ == '__main__': main() n = n + 1 average = total / n stdio.writeln( str(average) ) Both streams are infinite (no limit on their size). if __name__ == '__main__': main() Q. Do I always have to type in my input data and print my output? A. No! Keep data and results in files on your computer, or use piping to connect programs. 17 Redirection: keep data in files on your computer Redirect standard output to a file Redirect from a file to standard input % python randomseq.py 1000000 > data.txt % python average.py < data.txt 0.4947655567740991 % more data.txt "redirect standard output to" "take standard input from" 0.09474882292442943 0.2832974030384712 0.1833964252856476 0.2952177517730442 0.8035985765979008 0.7469424300071382 0.5835267075283997 0.3455279612587455... RandomSeq standard output stream file standard input stream Average Slight problem. Still limited by maximum file size. 18 Piping: entirely avoid saving data Q. There's no room for a huge file on my computer. Now what? A. No problem! Use piping. Piping. Connect standard output of one program to standard input of another. set up a pipe % python randomseq.py 1000000 | python average.py 0.4997970473016028 % python randomseq.py 1000000 | python average.py 0.5002071875644842 RandomSeq standard output stream standard input stream Average Critical point. No limit within programs on the amount of data they can handle. It is the job of the system to collect data on standard output and provide it to standard input. 19 Redirecting Standard Output to a Toast Printer Streaming algorithms Early computing Amount of available memory was much smaller than amount of data to be processed. But dramatic increases happened every year. Redirection and piping enabled programs to handle much more data than computers could store. Modern computing Amount of available memory is much smaller than amount of data to be processed. Dramatic increases still happen every year. Streaming algorithms enable our programs to handle much more data than our computers can store. Lesson. Avoid limits within your program whenever possible. 21 INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO Image sources http://www.digitalreins.com/wp-content/uploads/2013/05/Binary-code.jpg http://en.wikipedia.org/wiki/Punched_tape#mediaviewer/File:Harwell-dekatron-witch-10.jpg INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO 4. Input and Output Standard input and output Standard drawing Fractal drawings Animation Further improvements to our I/O abstraction Add the ability to create a drawing. command-line standard input stream arguments Python program standard output stream stddraw library Developed for this course, but broadly useful. Available for download at booksite. Included in introcs software. 24 stddraw library line(x0, y0, x1, y1) point(x, y) text(x, y, s) circle(x, y, r) also filledCircle, square(x, y, r) filledSquare and filledPolygon polygon(x, y, r) picture(picture, x, y) place.gif,.jpg or.png file Python program setPenRadius(r) setPenColor(c) setXscale(x0, x1) reset x range to [x0, x1) setYscale(y0, y1) reset y range to [y0, y1) standard drawing 25 show(dt) stddraw library II "Hello, World" for stddraw import math import stddraw def main(): Trace of drawing c = math.sqrt(3.0) / 2.0; (0.5, 0.866025...) stddraw.setPenRadius(0.01); stddraw.line(0.0, 0.0, 1.0, 0.0); stddraw.line(1.0, 0.0, 0.5, c); stddraw.line(0.5, c, 0.0, 0.0); stddraw.point(0.5, c/3.0); Hello, World stddraw.text(0.5, 0.5, "Hello, World"); stddraw.show() if __name__ == '__main__': main() (0, 0) (1, 0) 27 "Hello, World" for StdDraw window for standard drawing virtual terminal for editor import math import stddraw def main(): c = math.sqrt(3.0) / 2.0; stddraw.setPenRadius(0.01); stddraw.line(0.0, 0.0, 1.0, 0.0); stddraw.line(1.0, 0.0, 0.5, c); stddraw.line(0.5, c, 0.0, 0.0); stddraw.point(0.5, c/3.0); stddraw.text(0.5, 0.5, "Hello, World"); stddraw.show() if __name__ == '__main__': main() % % python triangle.py virtual terminal for OS commands 28 Exercise: Draw a square using lines? Answer: Draw a square using lines? import stddraw import math def main(): stddraw.line(0.0, 0.0, 1.0, 0.0) stddraw.line(1.0, 0.0, 1.0, 1.0) stddraw.line(1.0, 1.0, 0.0, 1.0) stddraw.line(0.0, 1.0, 0.0, 0.0) stddraw.point(0.5, 0.5); if __name__ == "__main__": main() stddraw application: data visualization import stdio import stddraw % more < USA.txt bounding def main(): 669905.0 247205.0 1244962.0 490000.0 box x0 = stdio.readFloat() 1097038.8890 245552.7780 coordinates of read coords of y0 = stdio.readFloat() 1103961.1110 247133.3330 13,509 US cities bounding box x1 = stdio.readFloat() 1104677.7780 247205.5560... y1 = stdio.readFloat() % python plotfilter.py < USA.txt stddraw.setXscale(x0, x1) rescale stddraw.setYscale(y0, y1) stddraw.setPenRadius(0.0) while not stdio.isEmpty(): read in points and plot them x = stdio.readFloat() y = stdio.readFloat() stddraw.point(x, y) stddraw.show() if __name__ == '__main__': main() 31 stddraw application: plotting a function Goal. Plot in the interval. % python plotfunctionex.py 20 Method. Take N samples, regularly spaced. import sys import stdio 20 import stddraw import stdarray import math def main(): N = int(sys.argv) x = stdarray.create1D(N+1, 0.0) % python plotfunctionex.py 200 y = stdarray.create1D(N+1, 0.0) for i in xrange(0, N+1): x[i] = math.pi * i / N y[i] = math.sin(4*x[i]) + math.sin(20*x[i]) stddraw.setXscale(0, math.pi); 200 stddraw.setYscale(-2.0, +2.0); for i in xrange(0, N): stddraw.line(x[i], y[i], x[i+1], y[i+1]) stddraw.show() if __name__ == '__main__': main() 32 INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO 4. Input and Output Standard input and output Standard drawing Fractal drawings Animation stddraw application: a random game Draw an equilateral triangle, label the vertices R, G, 0 1 2 3 4 5 6 7 8 9 10 B and make R the current point. B G B R G R R R B G G Pick a vertex at random. B Draw a point halfway between that vertex and the current point. Repeat. 2 vertex ID probability new x new y 0 8 (0, 0) R(0) 1/3.5x.5y 3 9 (1, 0) G(1) 1/3.5x +.5.5y 1 10 (.5, √3/2) B(2) 1/3.5x +.25.5y +.433 4 7 6 5 R G 35 stddraw application: a random game % python chaos.py 10000 import sys import stddraw import stdrandom import math def main(): trials = int(sys.argv) c = math.sqrt(3.0) / 2.0; cx = [ 0.000, 1.000, 0.500 ] cy = [ 0.000, 0.000, c ] stddraw.setPenRadius(0.01) x = 0.0 y = 0.0 for t in xrange(0, trials): r = int(stdrandom.uniform() * 3) x = (x + cx[r]) / 2.0 y = (y + cy[r]) / 2.0 stddraw.point(x, y) stddraw.show() if __name__ == '__main__': main() 36 Sierpinski triangles in the wild 1 1 1 odd numbers 1 2 1 1 3 3 1 1 4 6 4 1 1 5 10 10 5 1 1 6 15 20 15 6 1 1 7 21 35 35 21 7 1 Pascal's triangle 37 Exercise: Chaos Game Easy modification. Color point according to random vertex chosen using stddraw.setPenColor() to change the pen color. Answer import stddraw import random import sys def main(): T = (sys.argv) cx = [0.000, 1.000, 0.500] cy = [0.000, 0.000, 0.866] x = 0.0 y = 0.0 for t in range(0, T): r = random.randrange(0,3) x = (x + cx[r]) / 2.0 y = (y + cy[r]) / 2.0 if r == 0: stddraw.setPenColor(stddraw.RED) elif r == 1: stddraw.setPenColor(stddraw.GREEN) elif r == 2: stddraw.setPenColor(stddraw.BLUE) stddraw.point(x, y) stddraw.show() if __name__ == "__main__": main() Iterated function systems What happens when we change the rules? % python ifs.py 10000 < coral.txt probability new x new y 40%.31x −.53y +.89 −.46x −.29y + 1.10 15%.31x −.08y +.22.15x −.45y +.34 45%.55y +.01.69x −.20y +.38 ifs.py is a data-driven program that takes the coefficients from standard input. % more coral.txt 3 0.40 0.15 0.45 3 3 0.307692 -0.531469 0.8863493 0.307692 -0.076923 0.2166292 0.000000 0.545455 0.0106363 3 3 -0.461538 -0.293706 1.0962865 0.153846 -0.447552 0.3383760 0.692308 -0.195804 0.3808254 40 Iterated function systems Another example of changing the rules % python ifs.py 10000 < barnsley.txt probability new x new y 2% 0,5.27y 15% −.14x +.26y +.57.25x +.22y -.04 13%.17x −.21y +.41.22x +.18y +.09 70%.78x +.03y +.11 −.03x +.74y +.27 % more barnsley.txt 4.02.15.13.70 4 3.000.000.500 -.139.263.570.170 -.215.408.781.034.1075 4 3.000.270.000.246.224 -.036.222.176.0893 -.032.739.270 41 Iterated function systems Simple iterative computations yield patterns that are remarkably similar to those found in the natural world. Q. What does computation tell us about nature? an IFS fern a real fern Q. What does nature tell us about computation? 20th century sciences. Formulas. a real plant an IFS plant 21st century sciences. Algorithms? Note. You have seen many practical applications of integrated function systems, in movies and games. 42 INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO Image sources http://paulbourke.net/fractals/gasket/cokegasket.gif http://www.buzzfeed.com/atmccann/11-awesome-math-foods#39wokfk http://sheilakh.deviantart.com/art/The-Legend-of-Sierpinski-308953447 http://commons.wikimedia.org/wiki/File:Lady_Fern_frond_-_normal_appearance.jpg http://img3.wikia.nocookie.net/__cb20100707172110/jamescameronsavatar/images/e/e1/Avatar_concept_art-3.jpg INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO 4. Input and Output Standard input and output Standard drawing Fractal drawings Animation Standard Audio Animation rx+vx To create animation with stddraw. Repeat the following: Clear the screen. ry+vy ry Move the object. vy vx Draw the object. Display and pause briefly. rx When display time is much greater than the screen-clear time, we have the illusion of motion. Bouncing ball. Ball has position (rx, ry) and constant velocity (vx, vy). To move the ball, update position to (rx+vx, ry+vy). If the ball hits a vertical wall, set vx to -vx. 45 If the ball hits a horizontal wall, set vy to -vy. Bouncing ball bouncingball.py % python bouncingball.py import stddraw RADIUS =.05 radius DT = 20.0 def main(): stddraw.setXscale(-1.0, 1.0) rescale coordinates stddraw.setYscale(-1.0, 1.0) rx =.480 ry =.860 position vx =.015 constant velocity vy =.023 while True: # Update ball position and draw it there. if abs(rx + vx) + RADIUS > 1.0: vx = -vx bounce if abs(ry + vy) + RADIUS > 1.0: vy = -vy rx = rx + vx ry = ry + vy update position stddraw.clear(stddraw.GRAY) clear background stddraw.filledCircle(rx, ry, RADIUS) and draw ball stddraw.show(0) 46 INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO 4. Input and Output Standard input and output Standard drawing Fractal drawings Animation Standard Audio A set of I/O abstractions for Python Developed for this course, but broadly useful stdio, instream, outstream, stddraw, and stdaudio. Available for download at booksite (and included in introcs software). command-line standard input stream arguments Python program standard output stream standard audio 48 Crash course in sound Sound is the perception of the vibration of molecules. A musical tone is a steady periodic sound. A pure tone is a sinusoidal waveform. pitch i frequency (440*2i/12) sinusodial waveform Western musical scale A 0 440 Concert A is 440 Hz. A♯ / B♭ 1 466,16 B 2 493,88 12 notes, logarithmic scale. C 3 523,25 C♯ / D♭ 4 554,37 D 5 587,33 D♯ / E♭ 6 622,25 E 7 659,26 F 8 698,46 F♯ / G♭ 9 739,99 G 10 783,99 G♯ / A♭ 11 830,61 49 Digital audio same as when plotting a To represent a wave, sample at regular intervals and save the values in an array. function samples/sec samples sampled waveform 1/40 second of concert A 5 512 137 11 025 275 22 050 551 CD standard 44 100 1102 Bottom line. You can write programs to manipulate sound (arrays of float values). 50 stdaudio library Developed for this course, also broadly useful Play a sound wave (array of double values) on your computer’s audio output. Convert to and from standard.wav file format. public class stdaudio void playFile(file) play the given.wav file void playSamples(float[] a) play the given sound wave API void playSample(s) play the sample for 1/44100 second void save(file, a) save array a to.wav file float[] read(file) read array from a.wav file return type usually in signature but not in Python Enables you to hear the results of your programs that manipulate sound. 5 1 "Hello, World" for stdaudio playthatnote.py import stdio import stdarray import stdaudio import math i import sys def tone( hz, duration ): N = int(44100.0 * duration) a = stdarray.create1D( N+1, 0.0 ) for i in range(0, N+1): a[i] = math.sin(2.0 * math.pi * i * hz / 44100.0) % python playthatnote.py 440.0 3.0 return a % python playthatnote.py 880.0 3.0 def main(): % python playthatnote.py 220.0 3.0 hz = float(sys.argv) duration = float(sys.argv) % python playthatnote.py 494.0 3.0 a = tone(hz, duration) stdaudio.playSamples(a) 52 Exercise: Frere Jacques (Brother John) Frere Jacques. Play A B C# A for 0.5s each using stdaudio. And repeat at least once? Exercise: Write Down Notes Exercise: Write Down Notes Answer Play that tune Read a list of tones and durations from standard input to play a tune. playthattune.py % more < elise.txt import stdio 7.125 import stdaudio 6.125 import playthatnote 7.125 6.125 import math 7.125 import sys 2.125 def tone( hz, duration ): 5.125 … 3.125 ABCDEFGA same function as before 0.25 def main():... tempo = float( sys.argv ) % python playthattune.py 2.0 < elise.txt while (not stdio.isEmpty()): pitch = stdio.readInt() 76 7672530 duration = stdio.readFloat() * tempo hz = 440.0 * math.pow(2, pitch / 12.0) a = tone(hz, duration) stdaudio.playSamples(a) % python playthattune.py 1.0 < elise.txt if __name__ == '__main__': main() 57 INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO INTRO TO PROGRAMMING IN PYTHON SEDGEWICK・WAYNE・DONDERO Title Text 1.5 https://introcs.cs.princeton.edu/python Slides ported to Python by James Pope Last updated on 2023/10/20 08:43