03_Handout_1(12).pdf
Document Details
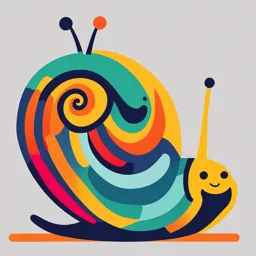
Uploaded by FascinatingCottonPlant4770
STI College
Full Transcript
SH1801 Conditionals and Loops I. Conditional Statements A. Decision Making Conditional statements are used to perform different actions based on different conditions. The if statement is one of the most frequently used conditional statements...
SH1801 Conditionals and Loops I. Conditional Statements A. Decision Making Conditional statements are used to perform different actions based on different conditions. The if statement is one of the most frequently used conditional statements. If the if statement’s condition expression evaluates to true, the block of code inside the if statement is executed. If the expression is found to be false, the code within the if statement is ignored and the first set of code after the end of the if statement (after the closing curly brace) is executed. Syntax: if (condition) { //Executes when the condition is true } Any of the following comparison operators may be used to form the condition: o Left Bracket () - greater than o Exclamation Mark and Equal Sign (!=) - not equal to o Double Equal Sign (= =) - equal to o Left Bracket and Equal Sign (=) - greater than or equal to int x = 7; if(x < 21) { System.out.println("Hello"); } B. if… else Statements An if statement can be followed by an optional else statement, which executes when the condition evaluates to false. int age = 30; if (age < 16) { System.out.println("Nope!"); } else { System.out.println("Welcome!"); } //Outputs "Welcome" II. Nested if Statements You can use one if-else statement inside another if or else statement. For example: int age = 25; if(age > 0) { if(age > 16) { System.out.println("Hello!"); }else { 03 Handout 1 *Property of STI [email protected] Page 1 of 7 SH1801 System. out.println("Not Allowed!"); } } else { System.out.println("Error"); } //Outputs "Hello!" III. else if Statements Instead of using nested if-else statements, you can use the else if statement to check multiple conditions. For example: int age = 25; if(age 320) { System.out.println("Bonjour!"); } } However, using the AND logical operator, denoted by two (2) ampersands (&&), is the easier way: if (age > 20 && money > 320) { System.out.println("Welcome!"); } B. The OR Operator The OR operator, denoted by two (2) vertical bars (||), checks if any one of the conditions is true. The condition becomes true, if any one of the operands evaluates to true. For example: int age = 31; int money = 50; if (age > 18 || money > 500) { 03 Handout 1 *Property of STI [email protected] Page 2 of 7 SH1801 System.out.println("Welcome!"); } //Outputs "Welcome!" C. The NOT Operator The NOT logical operator, denoted by a single exclamation point (!), is used to reverse the logical state of its operand. If a condition is true, the NOT logical operator will make it false. For example: int age = 25; if(!(age > 18)) { System.out.println("Too Young"); } else { System.out.println("Welcome"); } //Outputs "Welcome" V. The switch Statement A. The switch Statement A switch statement tests a variable for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each case. Syntax: switch (expression) { case value1 : //Statements break; //optional case value2 : //Statements break; //optional //You can have any number of case statements. default : //Optional //Statements } When the variable being switched on is equal to a case, the statements following that case will execute unit a break statement is reached. When a break statement is reached, the switch terminates, and the flow of control jumps to the next line after the switch statement. Not every case needs to contain a break. If no break appears, the flow of control will fall through to subsequent cases until a break is reached. The example tests day against a set of values and prints a corresponding message: int day = 3; switch(day) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; 03 Handout 1 *Property of STI [email protected] Page 3 of 7 SH1801 case 3: System.out.println("Wednesday"); break; } // Outputs "Wednesday" B. The default Statement A switch statement can have an option default case. The default case can be used for performing a task when none of the cases is matched. For example: int day = 3; switch(day) { case 6: System.out.println("Saturday"); break; case 7: System.out.println("Sunday"); break; default: System.out.println("Weekday"); } // Outputs "Weekday" VI. While Loops A loop statement allows to repeatedly execute a statement or group of statements. A while loop statement repeatedly executes a target statement as long as a given condition is true. For example: int x = 3; while(x > 0) { System.out.println(x); x--; } The while loops check for the condition x > 0. If it evaluates to true, it executes the statements within its body. Then it checks the statement again and repeats. When the expression is tested and the result is false, the loop body is skipped and the first statement after the while loop is executed. Example: int x = 6; while(x < 10) { System.out.println(x); 03 Handout 1 *Property of STI [email protected] Page 4 of 7 SH1801 x++; } System.out.println("Loop ended"); VII. For Loops Another loop structure is the for loop, which allows writing of a loop that needs to execute a specific number of times. Syntax: for (initialization; condition; increment/decrement) { statement(s) } Initialization expression executes only once during the beginning of the loop. The condition is evaluated each time the loop iterates. The loop executes the statement repeatedly until this condition returns false. Increment/Decrement executes after each iteration of the loop. The following example prints the numbers 1 through 5: for(int x = 1; x