Introduction to Java Programming and Data Structures PDF
Document Details
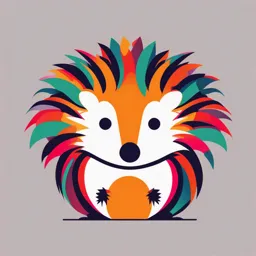
Uploaded by RegalHeather5514
2024
Y. Daniel Liang
Tags
Summary
This textbook provides an introduction to Java programming and data structures. It covers computer basics, programming languages, and various examples. The book also details programming languages and their uses, along with an examination of how Java works.
Full Transcript
Introduction to Java Programming and Data Structures Thirteenth Edition Chapter 1 Introduction to Computers, Programs, and Java Copyright © 2024 Pearson...
Introduction to Java Programming and Data Structures Thirteenth Edition Chapter 1 Introduction to Computers, Programs, and Java Copyright © 2024 Pearson Education, Inc. All Rights Reserved Objectives 1.1 To understand computer basics, programs, and programming languages 1.2 To understand the meaning of Java language specification, J DK, and IDE 1.3 To write a simple Java program (§1.7). 1.4 To display output on the console (§1.7). 1.5 To explain the basic syntax of a Java program (§1.7). 1.6 To create, compile, and run Java programs (§1.8). 1.7 To use sound Java programming style and document programs properly (§1.9). 1.8 To explain the differences between syntax errors, runtime errors, and logic errors (§1.10). 1.9 To develop Java programs using NetBeans (§1.11). 1.10 To develop Java programs using Eclipse (§1.12). Copyright © 2024 Pearson Education, Inc. All Rights Reserved What Is a Computer? A computer consists of a CPU, memory, hard disk, floppy disk, monitor, printer, and communication devices. Bus Storage Communication Input Output Memory CPU Devices Devices Devices Devices e.g., Disk, CD, e.g., Modem, e.g., Keyboard, e.g., Monitor, and Tape and NIC Mouse Printer Copyright © 2024 Pearson Education, Inc. All Rights Reserved Programs Computer programs, known as software, are instructions to the computer. You tell a computer what to do through programs. Without programs, a computer is an empty machine. Computers do not understand human languages, so you need to use computer languages to communicate with them. Programs are written using programming languages. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Programming Languages (1 of 3) 1.Machine Language 2.Assembly Language 3.High-Level Language Machine language is a set of primitive instructions built into every computer. The instructions are in the form of binary code, so you have to enter binary codes for various instructions. Program with native machine language is a tedious process. Moreover the programs are highly difficult to read and modify. For example, to add two numbers, you might write an instruction in binary like this: 1101101010011010 Copyright © 2024 Pearson Education, Inc. All Rights Reserved Programming Languages (2 of 3) Machine Language Assembly Language High-Level Language Assembly languages were developed to make programming easy. Since the computer cannot understand assembly language, however, a program called assembler is used to convert assembly language programs into machine code. For example, to add two numbers, you might write an instruction in assembly code like this: ADDF3 R1, R2, R3 Copyright © 2024 Pearson Education, Inc. All Rights Reserved Programming Languages (3 of 3) Machine Language Assembly Language High-Level Language The high-level languages are English-like and easy to learn and program. For example, the following is a high-level language statement that computes the area of a circle with radius 5: area 5 * 5 * 3.1415; Copyright © 2024 Pearson Education, Inc. All Rights Reserved Popular High-Level Languages Language Description Ada Named for Ada Lovelace, who worked on mechanical general-purpose computers. The Ada language was developed for the Department of Defense and is used mainly in defense projects. BASIC Beginner’s All-purpose Symbolic Instruction Code. It was designed to be learned and used easily by beginners. C Developed at Bell Laboratories. C combines the power of an assembly language with the ease of use and portability of a high-level language. C++ C++ is an object-oriented language, based on C. C# Pronounced “C Sharp.” It is a hybrid of Java and C++ and was developed by Microsoft. COBOL COmmon Business Oriented Language. Used for business applications. FORTRAN FORmula TRANslation. Popular for scientific and mathematical applications. Java Developed by Sun Microsystems, now part of Oracle. It is widely used for developing platform- independent Internet applications. Pascal Named for Blaise Pascal, who pioneered calculating machines in the seventeenth century. It is a simple, structured, general-purpose language primarily for teaching programming. Python A simple general-purpose scripting language good for writing short programs. Visual Basic Visual Basic was developed by Microsoft and it enables the programmers to rapidly develop graphical user interfaces. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Interpreting/Compiling Source Code A program written in a high-level language is called a source program or source code. Because a computer cannot understand a source program, a source program must be translated into machine code for execution. The translation can be done using another programming tool called an interpreter or a compiler. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Interpreting Source Code An interpreter reads one statement from the source code, translates it to the machine code or virtual machine code, and then executes it right away, as shown in the following figure. Note that a statement from the source code may be translated into several machine instructions. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Compiling Source Code A compiler translates the entire source code into a machine-code file, and the machine-code file is then executed, as shown in the following figure. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Why Java? The answer is that Java enables users to develop and deploy applications on the Internet for servers, desktop computers, and small hand-held devices. The future of computing is being profoundly influenced by the Internet, and Java promises to remain a big part of that future. Java is the Internet programming language. Java is a general purpose programming language. Java is the Internet programming language. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Java, Web, and Beyond Java can be used to develop standalone applications. Java can be used to develop applications running from a browser. Java can also be used to develop applications for hand-held devices. Java can be used to develop applications for Web servers. Copyright © 2024 Pearson Education, Inc. All Rights Reserved JDK Versions JDK 1.02 (1995) JDK 1.1 (1996) JDK 1.2 (1998) JDK 1.3 (2000) JDK 1.4 (2002) JDK 1.5 (2004) a. k. a. JDK 5 or Java 5 JDK 1.6 (2006) a. k. a. JDK 6 or Java 6 JDK 1.7 (2011) a. k. a. JDK 7 or Java 7 JDK 1.8 (2014) a. k. a. JDK 8 or Java 8 Java 9, 10, 11, 12, 13, 14. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Popular Java IDEs NetBeans Eclipse Copyright © 2024 Pearson Education, Inc. All Rights Reserved A Simple Java Program Listing 1.1 // This program prints Welcome to Java! public class Welcome { public static void main(String[] args) { System.out.println("Welcome to Java!"); } } Welcome Note: Clicking the green button displays the source code with interactive animation. You can also run the code in a browser. Internet connection is needed for this button. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Trace a Program Execution (1 of 3) Copyright © 2024 Pearson Education, Inc. All Rights Reserved Trace a Program Execution (2 of 3) Copyright © 2024 Pearson Education, Inc. All Rights Reserved Trace a Program Execution (3 of 3) Copyright © 2024 Pearson Education, Inc. All Rights Reserved Two More Simple Examples WelcomeWithThreeMessages ComputeExpression Copyright © 2024 Pearson Education, Inc. All Rights Reserved Creating, Compiling, and Running Programs Copyright © 2024 Pearson Education, Inc. All Rights Reserved Compiling Java Source Code You can port a source program to any machine with appropriate compilers. The source program must be recompiled, however, because the object program can only run on a specific machine. Nowadays computers are networked to work together. Java was designed to run object programs on any platform. With Java, you write the program once, and compile the source program into a special type of object code, known as bytecode. The bytecode can then run on any computer with a Java Virtual Machine, as shown below. Java Virtual Machine is a software that interprets Java bytecode. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Supplements on the Companion Website See Supplement I.B for installing and configuring JDK See Supplement I.C for compiling and running Java from the command window for details www.pearsonhighered.com/liang Copyright © 2024 Pearson Education, Inc. All Rights Reserved Anatomy of a Java Program Class name Main method Statements Statement terminator Reserved words Comments Blocks Copyright © 2024 Pearson Education, Inc. All Rights Reserved Class Name Every Java program must have at least one class. Each class has a name. By convention, class names start with an uppercase letter. In this example, the class name is Welcome. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Main Method Line 2 defines the main method. In order to run a class, the class must contain a method named main. The program is executed from the main method. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Statement A statement represents an action or a sequence of actions. The statement System.out.println(“Welcome to Java!”) in the program in Listing 1.1 is a statement to display the greeting “Welcome to Java!”. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Statement Terminator Every statement in Java ends with a semicolon (;). Copyright © 2024 Pearson Education, Inc. All Rights Reserved Keywords Keywords are words that have a specific meaning to the compiler and cannot be used for other purposes in the program. For example, when the compiler sees the word class, it understands that the word after class is the name for the class. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Blocks A pair of braces in a program forms a block that groups components of a program. public class Test { Class block public static void main(String[] args) { System.out.println("Welcome to Java!"); Method block } } Copyright © 2024 Pearson Education, Inc. All Rights Reserved Special Symbols Character Name Description {} Opening and closing Denotes a block to enclose statements. braces () Opening and closing Used with methods. parentheses [] Opening and closing Denotes an array. brackets // Double slashes Precedes a comment line. “” Opening and closing Enclosing a string (i.e., sequence of quotation marks characters). ; Semicolon Marks the end of a statement. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Braces { … } Copyright © 2024 Pearson Education, Inc. All Rights Reserved Parentheses ( … ) Copyright © 2024 Pearson Education, Inc. All Rights Reserved Statement Terminator ; Copyright © 2024 Pearson Education, Inc. All Rights Reserved // comments Copyright © 2024 Pearson Education, Inc. All Rights Reserved “ string ” Copyright © 2024 Pearson Education, Inc. All Rights Reserved Programming Style and Documentation Appropriate Comments Naming Conventions Proper Indentation and Spacing Lines Block Styles Copyright © 2024 Pearson Education, Inc. All Rights Reserved Appropriate Comments Include a summary at the beginning of the program to explain what the program does, its key features, its supporting data structures, and any unique techniques it uses. Include your name, class section, instructor, date, and a brief description at the beginning of the program. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Naming Conventions Choose meaningful and descriptive names. Class names: – Capitalize the first letter of each word in the name. For example, the class name ComputeExpression. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Proper Indentation and Spacing Indentation – Indent two spaces. Spacing – Use blank line to separate segments of the code. Copyright © 2024 Pearson Education, Inc. All Rights Reserved Block Styles Use end-of-line style for braces. Next-line public class Test style { public static void main(String[] args) { System.out.println("Block Styles"); } } End-of-line style public class Test { public static void main(String[] args) { System.out.println("Block Styles"); } } Copyright © 2024 Pearson Education, Inc. All Rights Reserved Programming Errors Syntax Errors – Detected by the compiler Runtime Errors – Causes the program to abort Logic Errors – Produces incorrect result Copyright © 2024 Pearson Education, Inc. All Rights Reserved Syntax Errors public class ShowSyntaxErrors { public static main(String[] args) { System.out.println("Welcome to Java); } } ShowSyntaxErrors Copyright © 2024 Pearson Education, Inc. All Rights Reserved Runtime Errors public class ShowRuntimeErrors { public static void main(String[] args) { System.out.println(1 / 0); } } ShowRuntimeErrors Copyright © 2024 Pearson Education, Inc. All Rights Reserved Logic Errors public class ShowLogicErrors { public static void main(String[] args) { System.out.println("Celsius 35 is Fahrenheit degree "); System.out.println((9 / 5) * 35 + 32); } } Output: Celsius 35 is Fahrenheit degree 67 Logic Errors ShowLogicErrors Copyright © 2024 Pearson Education, Inc. All Rights Reserved Compiling and Running Java From NetBeans See Supplement II.B on the Website for details Copyright © 2024 Pearson Education, Inc. All Rights Reserved Compiling and Running Java From Eclipse See Supplement II.D on the Website for details Copyright © 2024 Pearson Education, Inc. All Rights Reserved Copyright This work is protected by United States copyright laws and is provided solely for the use of instructors in teaching their courses and assessing student learning. Dissemination or sale of any part of this work (including on the World Wide Web) will destroy the integrity of the work and is not permitted. The work and materials from it should never be made available to students except by instructors using the accompanying text in their classes. All recipients of this work are expected to abide by these restrictions and to honor the intended pedagogical purposes and the needs of other instructors who rely on these materials. Copyright © 2024 Pearson Education, Inc. All Rights Reserved