Write a C program to find the Fibonacci sequence of n terms using recursion.
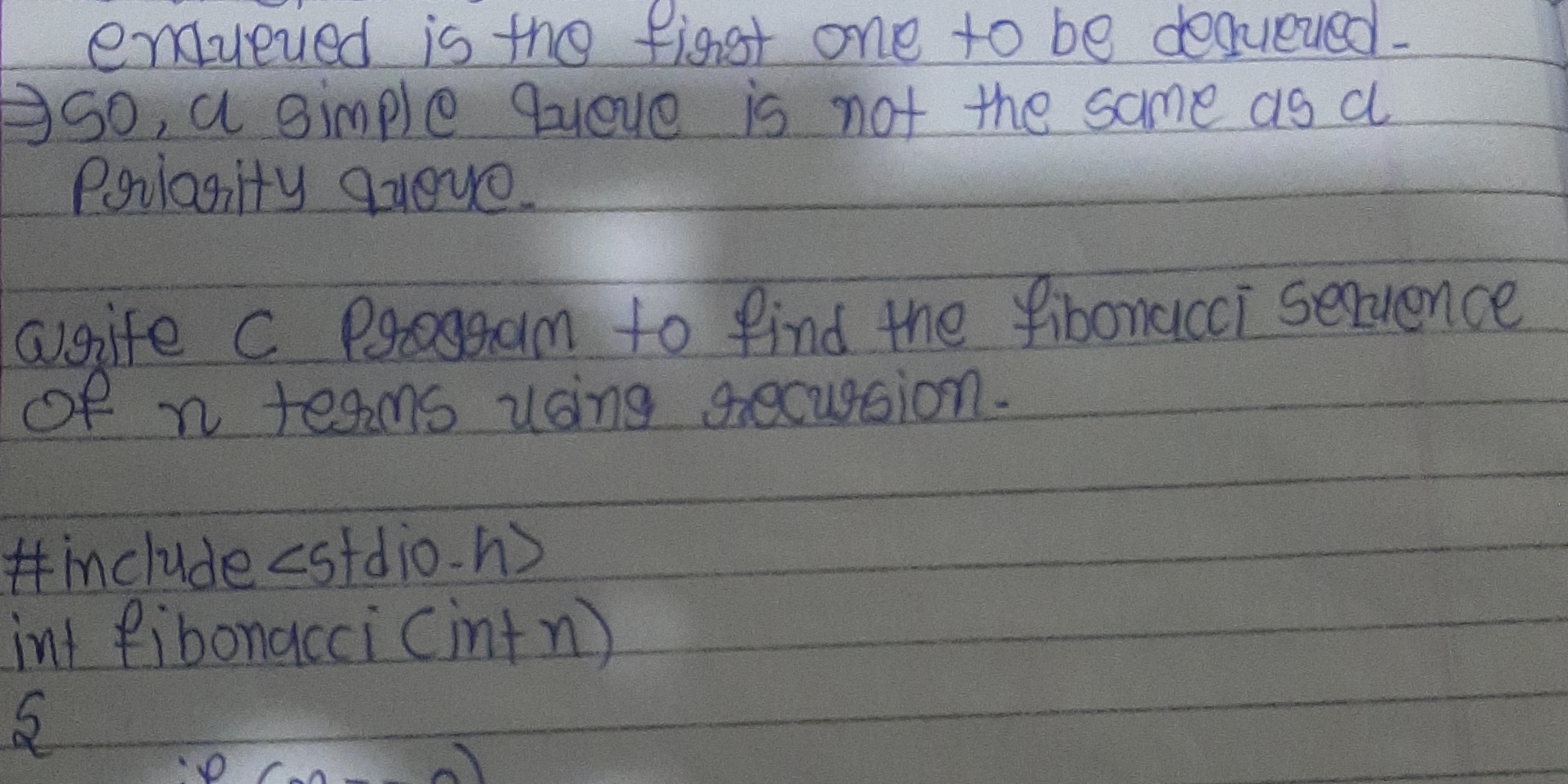
Understand the Problem
The question is asking to write a C program that calculates the Fibonacci sequence for a specified number of terms using recursion.
Answer
The C program calculates Fibonacci numbers using a recursive function and prints the sequence based on user input.
Answer for screen readers
The final C program to calculate the Fibonacci sequence using recursion is:
#include <stdio.h>
int fibonacci(int n) {
if (n == 0) return 0; // Base case for 0
if (n == 1) return 1; // Base case for 1
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
int main() {
int n;
printf("Enter the number of terms: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("%d ", fibonacci(i)); // Print each Fibonacci number
}
return 0;
}
Steps to Solve
- Include Necessary Libraries
Start by including the standard input-output library which allows for using functions like
printf
andscanf
.
#include <stdio.h>
- Define the Fibonacci Function Create a recursive function to calculate Fibonacci numbers. The base cases are defined first, returning 0 for Fibonacci(0) and 1 for Fibonacci(1). For other values, return the sum of the two previous Fibonacci numbers.
int fibonacci(int n) {
if (n == 0) return 0; // Base case for 0
if (n == 1) return 1; // Base case for 1
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
- Main Function to Handle User Input
In the
main
function, prompt the user for the number of terms they want in the Fibonacci sequence. Use a loop to call thefibonacci
function and print each term.
int main() {
int n;
printf("Enter the number of terms: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("%d ", fibonacci(i)); // Print each Fibonacci number
}
return 0;
}
- Compile and Run the Program Use a C compiler to compile your program and run it. Enter the desired number of terms when prompted.
The final C program to calculate the Fibonacci sequence using recursion is:
#include <stdio.h>
int fibonacci(int n) {
if (n == 0) return 0; // Base case for 0
if (n == 1) return 1; // Base case for 1
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
int main() {
int n;
printf("Enter the number of terms: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("%d ", fibonacci(i)); // Print each Fibonacci number
}
return 0;
}
More Information
The Fibonacci sequence is a series where each number is the sum of the two preceding ones, usually starting with 0 and 1. The sequence appears in various areas of mathematics, art, and nature.
Tips
- Not handling base cases: Forgetting to define the base cases for 0 and 1 can lead to infinite recursion.
- Overlooking user input validation: Ensure to check if the user input for
n
is non-negative, as Fibonacci is not defined for negative indices.
AI-generated content may contain errors. Please verify critical information